摘要: Error creating bean with name 'XXX': Requested bean is currently in creation: Is there an unresolvable circular reference?; nested exception is org.springframework.beans.factory.BeanCurrentlyInCreationException:
如果把MapperScan单独配置,就不会有警告
DataSource,SqlSessionFactory,MapperScan有依赖关系.
如果把MapperScan单独配置,就不会有警告,例如:
code:
DataSource:
import com.alibaba.druid.spring.boot.autoconfigure.DruidDataSourceBuilder; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Primary; import javax.sql.DataSource; @Configuration public class DataSourceConfig { @ConfigurationProperties("spring.datasource.druid") @Bean public DataSource equipDataSource() { return DruidDataSourceBuilder.create().build(); } }
SqlSessionFactory:
import org.apache.ibatis.session.SqlSessionFactory; import org.mybatis.spring.SqlSessionFactoryBean; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.core.io.support.PathMatchingResourcePatternResolver; import javax.sql.DataSource; @SuppressWarnings("SpringJavaAutowiringInspection") @Configuration public class MyBatisConfig { @Bean public SqlSessionFactory sqlSessionFactory(DataSource dataSource) throws Exception { PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver(); SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean(); factoryBean.setDataSource(dataSource); factoryBean.setTypeAliasesPackage("com.app.domain.entity");// 指定基包 factoryBean.setMapperLocations(resolver.getResources("classpath:mapper/**/*.xml"));// return factoryBean.getObject(); } }
MapperScannerConfigurer
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import tk.mybatis.mapper.common.BaseMapper; import tk.mybatis.spring.mapper.MapperScannerConfigurer; import java.util.Properties; @Configuration public class MapperConfig { @Bean public MapperScannerConfigurer mapperScannerConfigurer() { MapperScannerConfigurer mapperScannerConfigurer = new MapperScannerConfigurer(); mapperScannerConfigurer.setBasePackage("com.app.domain.mapper"); Properties properties = new Properties(); // 这里要特别注意,不要把BaseMapper放到 basePackage 中,也就是不能同其他Mapper一样被扫描到。 properties.setProperty("notEmpty", "false"); properties.setProperty("IDENTITY", "MYSQL"); mapperScannerConfigurer.setProperties(properties); mapperScannerConfigurer.setMarkerInterface(BaseMapper.class); return mapperScannerConfigurer; } }
https://my.oschina.net/doctor2014/blog/386431
Requested bean is currently in creation: Is there an unresolvable circular reference?

bean.xml配置如下:
<?xml version="1.0" encoding="GBK"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"
default-autowire="byName">
<bean id="a" class="BeanA" scope="prototype"/>
<bean id="b" class="BeanB" scope="prototype"/>
</beans>
BeanA类如下:
public class BeanA
{
private BeanB b;
public BeanB getB() {
return b;
}
public void setB(BeanB b) {
this.b = b;
}
}
BeanB类如下:
public class BeanB
{
private BeanA a;
public BeanA getA() {
return a;
}
public void setA(BeanA a) {
this.a = a;
}
}
程序执行的堆栈信息:
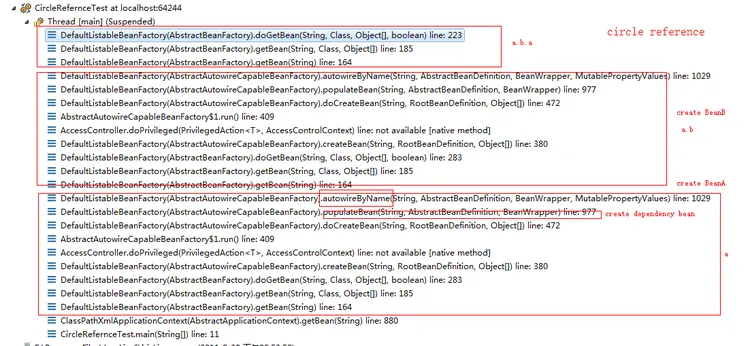
if (isPrototypeCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
}
会抛出一个异常:Requested bean is currently in creation: Is there an unresolvable circular reference,然后scope是singlton或者其他的时候bean的创建过程不一样,不会有一个这样子的判断,因而不会抛出这样子的错误。
因为scope=prototype,所以每次请求对应不同的bean,所以在创建的时候有一个依赖的先后顺序,而如果bean配置成singleton,则属性间初始化的顺序可以无所谓了,所有的bean都只有一份,所以在bean是singleton时候不会报unresolvable circular reference的错误。