这是最终的文件目录结构:
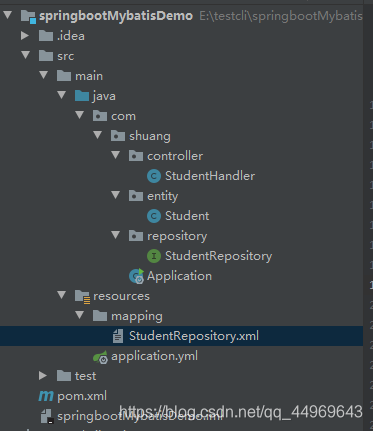
**新建maven工程**
1,配置pom.xml
```yaml
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.5.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-atarter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.15</version>
</dependency>
<dependency>
<groupId>org.projectlomok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
```
2,创建数据表
```sql
create table studentMB(
id int primary key auto_increment,
name varchar(11),
score double,
birthday date,
);
```
3,创建对应的实体类
```java
package com.shuang.entity;
import lombok.Data;
import java.util.Date;
@Data
public class Student {
private Long id;
private String name;
private Double score;
private Date birthday;
}
```
4、创建StudentRepository
```java
package com.shuang.repository;
import com.shuang.entity.Student;
import java.util.List;
public interface StudentRepository {
public List<Student> findAll();
public Student findById(Long id);
public void save(Student student);
public void update(Student student);
public void deleteById(Long id);
}
```
5,在resources/mapping路径下创建StudentRepository对应的Mapper.xml
```yaml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!-- namespace:该mapper.xml映射文件的唯一标识 -->
<mapper namespace="org.shuang.repository.StudentRepository">
<select id="findAll" resultType="Student">
select * from student;
</select>
<select id="findById" parameterType="java.lang.Long" resultType="Student">
select * from student where id=#{id};
</select>
<insert id="save" parameterType="Student">
insert into student(name,score,birthday) values(#{name},#{score},#{birthday});
</insert>
<update id="update" parameterType="Student">
update student set name=#{name},score=#{score},birthday=#{birthday} where id=#{id};
<update>
<delete id="deleteById" parameterType="java.lang.Long">
delete from student where id=#{id};
</delete>
</mapper>
```
6,创建StudentHandler,注入StudentRepository
```java
package com.shuang.controller;
import com.shuang.entity.Student;
import com.shuang.repository.StudentRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
public class StudentHandler {
@Autowired
private StudentRepository studentRepository;
@GetMapping("/findAll")
public List<Student> findAll(){
return studentRepository.findAll();
}
@PostMapping("/findById/{id}")
public void save(@RequestBody Student student){
studentRepository.save(student);
}
@PutMapping("/update")
public void update(@RequestBody Student student){
studentRepository.update(student);
}
@DeleteMapping("/deleteById/{id}")
public void deleteById(@PathVariable("id") Long id){
studentRepository.deleteById(id);
}
```
7,application.yml
```yaml
spring:
datasource:
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=UTF-8
&serverTimezone=GMT
username: shuang
password: mysql215311?
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis:
mapper-locations: classpath:/mapping/*.xml
type-aliases-package: com.shuang.entity
```
```
```
8,创建Application
```java
package com.shuang;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.shuang.repository")
public class Application {
public static void main(String [] args){
SpringApplication.run(Application.class,args);
}
}
```
启动:使用端口就可以访问了
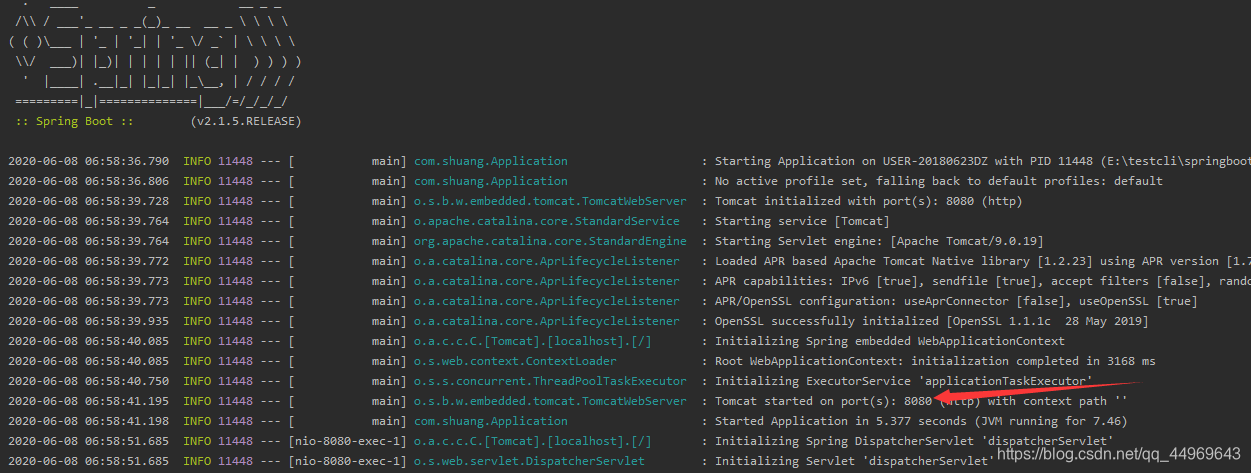