AddComponentMenu
AddComponentMenu属性允许您将脚本放置在“组件”菜单中的任何位置,而不仅仅是“组件 - >脚本”菜单。
您可以使用它来更好地组织“组件”菜单,这样可以在添加脚本时改进工作流程。重要提示:您需要重新启动。
- componentOrder 组件菜单中的组件顺序(低于顶部)。
- AddComponentMenu 在“组件”菜单中添加一个项目。
using UnityEngine;
[AddComponentMenu("Transform/Follow Transform")]
public class FollowTransform : MonoBehaviour
{
}
效果如下
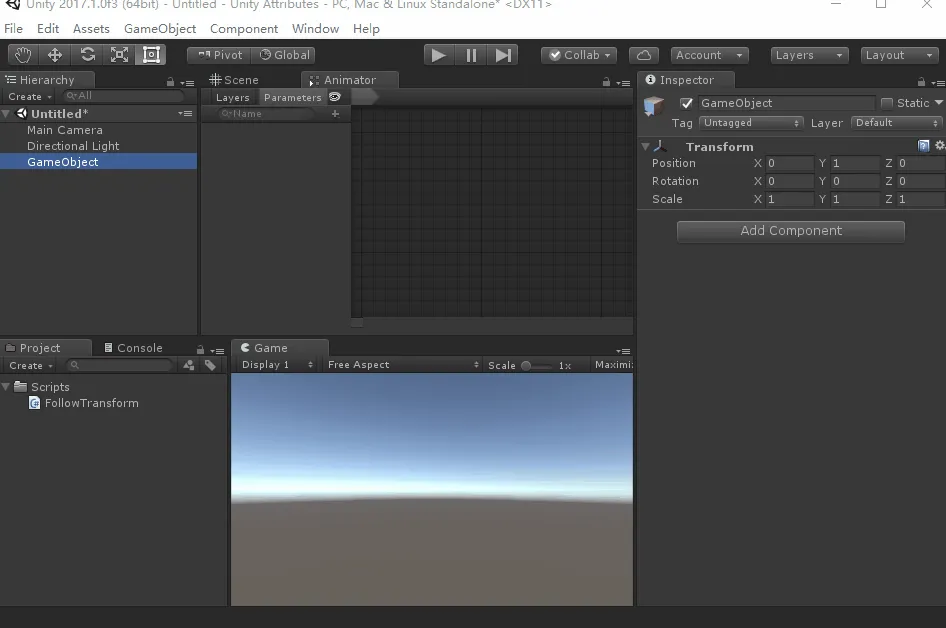
AssemblyIsEditorAssembly
装配级属性。具有此属性的程序集中的任何类将被视为编辑器类。
构造函数
AssemblyIsEditorAssembly
ContextMenu
ContextMenu属性允许您向上下文菜单添加命令。在所附脚本的督察中。当用户选择上下文菜单时,将执行该功能。这对于自动从脚本设置场景数据是最有用的。该功能必须是非静态的。
using UnityEngine;
public class ContextTesting : MonoBehaviour
{
/// Add a context menu named "Do Something" in the inspector
/// of the attached script.
[ContextMenu("Do Something")]
void DoSomething()
{
Debug.Log("Perform operation");
}
}
- ContextMenu 将功能添加到组件的上下文菜单。
效果如下

ContextMenuItemAttribute
使用此属性将上下文菜单添加到调用命名方法的字段。
using UnityEngine;
using System.Collections;
public class ExampleClass : MonoBehaviour
{
[ContextMenuItem("Reset", "ResetBiography")]
[Multiline(8)]
public string playerBiography = "";
void ResetBiography()
{
playerBiography = "";
}
}
效果如下

CreateAssetMenu
fileName
新创建的此类实例使用的默认文件名。(创建文件必须以 .asset 结尾)
menuName
此类型显示的名称显示在“资产/创建”菜单中。
order
菜单项在资产/创建菜单中的位置。
using UnityEngine;
[CreateAssetMenu(fileName = "自定义资源.asset", menuName = "菜单/子项0")]
public class CreateAsset : ScriptableObject
{
public string Name = "自定义资源";
public Vector3[] Pos = new Vector3[10];
}
效果如下

DelayedAttribute
用于在脚本中使float,int或string变量的属性被延迟。
当使用此属性时,float,int或text字段将不会返回一个新的值,直到用户按下enter或焦点离开该字段。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class DelayedAttributeExample : MonoBehaviour
{
[DelayedAttribute()]
public string content;
}
效果如下
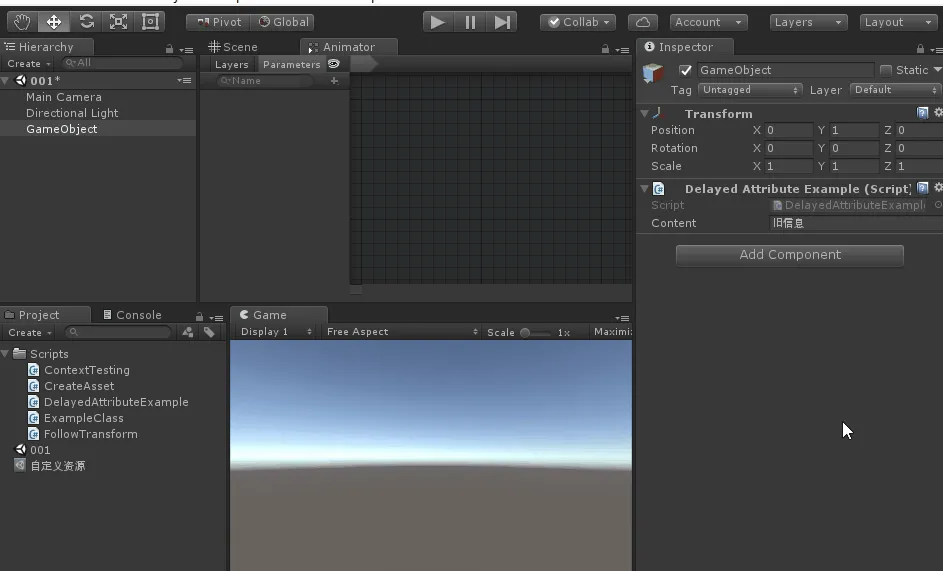
DisallowMultipleComponent
防止将相同类型(或子类型)的MonoBehaviour多次添加到GameObject。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[DisallowMultipleComponent]
public class Disallow : MonoBehaviour
{
}
效果如下

ExecuteInEditMode
使脚本的所有实例在编辑模式下执行。
默认情况下,MonoBehaviours只能在播放模式下执行。
通过添加此属性,MonoBehaviour的任何实例将在编辑器不处于播放模式时执行其回调函数。
这些功能不会像播放模式一样被调用。
更新仅在场景中的某些内容更改时才调用。当游戏视图接收到一个事件时,OnGUI被调用。OnRenderObject和其他渲染回调函数在场景视图或游戏视图的每次重绘时都被调用。
另请参见:runInEditMode。
using UnityEngine;
[ExecuteInEditMode]
public class PrintAwake : MonoBehaviour
{
void Awake()
{
Debug.Log("Editor causes this Awake");
}
void Update()
{
Debug.Log("Editor causes this Update");
}
}
效果如下

GUITargetAttribute
控制对应的OnGUI在那个Display上显示
using UnityEngine;
public class ExampleClass1 : MonoBehaviour
{
// Label will appear on display 0 and 1 only
[GUITarget(0, 1)]
void OnGUI()
{
GUI.Label(new Rect(10, 10, 300, 100), "Visible on TV and Wii U GamePad only");
}
}
效果如下

HeaderAttribute
在Inspector 中显示属性对应属性的注释
使用此
PropertyAttribute在检查器中的某些字段上方添加标题。
标题是使用DecoratorDrawer完成的。
using UnityEngine;
using System.Collections;
public class ExampleClass : MonoBehaviour
{
[Header("生命 Settings")]
public int health = 0;
public int maxHealth = 100;
[Header("防御 Settings")]
public int shield = 0;
public int maxShield = 0;
}
效果如下
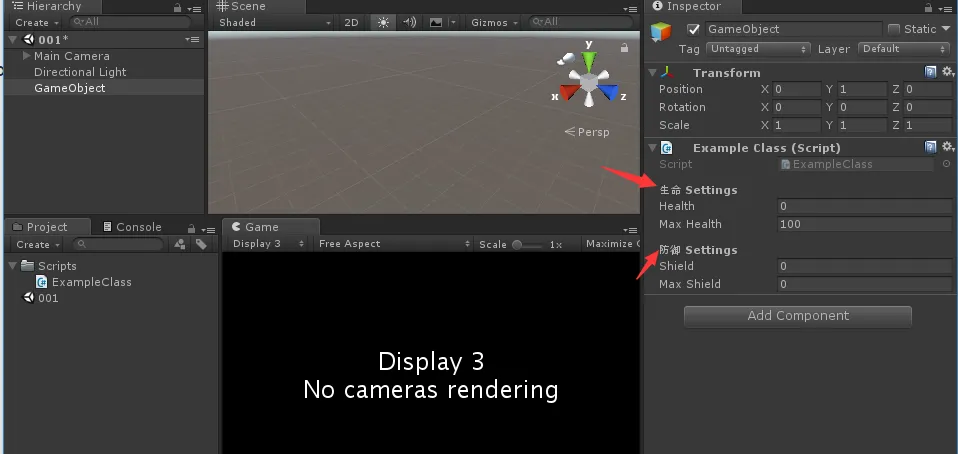
HelpURLAttribute
帮助图标对应跳转的URL(注意此类需要继承MonoBehaviour,不继承在2017测试跳转地址无效)
using UnityEngine;
using UnityEditor;
[HelpURL("http://www.jianshu.com/u/84e03bc5c4a6")]
public class MyComponent:MonoBehaviour
{
}
效果如下

HideInInspector
隐藏在Inspector面板中显示的Public属性
using UnityEngine;
using System.Collections;
public class ExampleClass : MonoBehaviour
{
[HideInInspector]
public int Hide = 0;
[Header("生命 Settings")]
public int health = 0;
public int maxHealth = 100;
[Header("防御 Settings")]
public int shield = 0;
public int maxShield = 0;
}
效果如下

ImageEffectAllowedInSceneView
具有此属性的任何图像效果可以渲染到场景视图相机中。
如果您希望将图像效果应用于场景视图相机,则会添加此属性。效果将应用于相同的位置,并且具有与相机效果相同的值。(5.4开始新加的特性,目前不知道怎么用)
MultilineAttribute
用于使字符串值的属性显示在多行文本区域中。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Example1 : MonoBehaviour
{
[MultilineAttribute(10)]
public string str = "";
}
效果如下

PreferBinarySerialization
这对于包含大量数据的自定义资产类型很有用。始终保持存储为二进制可以提高读/写性能,并在磁盘上生成更紧凑的表示。(说白了就是一二进制形式保存提高性能)
using UnityEngine;
[CreateAssetMenu]
[PreferBinarySerialization]
public class CustomData : ScriptableObject
{
public float[] lotsOfFloatData = new[] { 1f, 2f, 3f };
public byte[] lotsOfByteData = new byte[] { 4, 5, 6 };
}
PropertyAttribute
派生自定义属性属性的基类。
使用它来创建脚本变量的自定义属性。
自定义属性可以与自定义的
[PropertyDrawer(https://docs.unity3d.com/2017.2/Documentation/ScriptReference/PropertyDrawer.html)类挂钩,以控制如何在Inspector中显示具有该属性的脚本变量。
另请参见:PropertyDrawer类。
RangeAttribute
用于在脚本中创建一个float或int变量的属性被限制在一个特定的范围内。
当使用此属性时,float或int将在Inspector中显示为滑块,而不是默认数字字段
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Example1 : MonoBehaviour
{
[RangeAttribute(0,1000f)]
public int speed;
}
效果如下

RequireComponent
RequireComponent属性自动将所需组件添加为依赖关系。
当您将一个使用RequireComponent的脚本添加到GameObject时,所需的组件将自动添加到GameObject中。这有助于避免安装错误。例如,脚本可能需要将Rigidbody总是添加到同一个GameObject中。使用RequireComponent这将自动完成,因此您永远不会得到设置错误。请注意,RequireComponent仅在组件添加到GameObject的时刻检查缺少的依赖关系。GameObject缺少新依赖关系的组件的现有实例将不会自动添加这些依赖关系。(自动添加指定组建,有组件的不添加,没有的添加)
using UnityEngine;
[RequireComponent(typeof(Rigidbody))]
public class PlayerScript : MonoBehaviour
{
Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
rb.AddForce(Vector3.up);
}
}
效果如下

RuntimeInitializeOnLoadMethodAttribute
允许运行时类方法在运行时加载游戏时被初始化,而不需要用户的操作。
标记[RuntimeInitializeOnLoadMethod]的方法在游戏加载后被调用。这是在Awake方法被调用之后。
注意:[RuntimeInitializeOnLoadMethod]不保证标记的方法的执行顺序。调用的方法需静态
场景加载前调用
[RuntimeInitializeOnLoadMethodAttribute(RuntimeInitializeLoadType.BeforeSceneLoad)]
场景加载后调用
[RuntimeInitializeOnLoadMethod(RuntimeInitializeLoadType.AfterSceneLoad)]
// Create a non-MonoBehaviour class which displays
// messages when a game is loaded.
using UnityEngine;
class MyClass
{
[RuntimeInitializeOnLoadMethod]
static void OnRuntimeMethodLoad()
{
Debug.Log("场景加载和游戏运行后");
}
[RuntimeInitializeOnLoadMethod]
static void OnSecondRuntimeMethodLoad()
{
Debug.Log("SecondMethod场景加载和游戏运行后");
}
}
效果如下

SelectionBaseAttribute
将此属性添加到脚本类以将其GameObject标记为用于场景视图挑选的选择基础对象。
在Unity Scene View中,单击选择对象时,Unity将尝试找出最适合您选择的对象。如果单击作为预制体一部分的对象,则选择预制根的根,因为预制根被视为选择库。您也可以使其他对象也被视为选择库。您需要使用SelectionBase属性创建一个脚本类,然后您需要将该脚本添加到GameObject中。
using UnityEngine;
[SelectionBase]
public class PlayerScript : MonoBehaviour
{
}
效果如下

SerializeField
在Inspector可以看到标记为private的属性
using UnityEngine;
public class SomePerson : MonoBehaviour
{
//This field gets serialized because it is public.
public string firstName = "John";
//This field does not get serialized because it is private.
private int age = 40;
//This field gets serialized even though it is private
//because it has the SerializeField attribute applied.
[SerializeField]
private bool hasHealthPotion = true;
void Start()
{
if (hasHealthPotion)
Debug.Log("Person's first name: " + firstName + " Person's age: " + age);
}
}
效果如下

SharedBetweenAnimatorsAttribute
SharedBetweenAnimatorsAttribute是一个属性,指定该StateMachineBehaviour应仅实例化一次,并在所有Animator实例之间共享。
此属性减少每个控制器实例的内存占用。
程序员可以选择哪个StateMachineBehaviour可以使用此属性。
请注意,如果您的StateMachineBehaviour更改一些成员变量,它将影响使用它的所有其他Animator实例。
另请参见:StateMachineBehaviour类。
using UnityEngine;
[SharedBetweenAnimators]
public class AttackBehaviour : StateMachineBehaviour
{
public override void OnStateEnter(Animator animator, AnimatorStateInfo stateInfo, int layerIndex)
{
Debug.Log("OnStateEnter");
}
}
SpaceAttribute
使用此
PropertyAttribute在检查器中添加一些间距。
间距使用DecoratorDrawer完成。
using UnityEngine;
using System.Collections;
public class ExampleClass2 : MonoBehaviour
{
public int health = 0;
public int maxHealth = 100;
[Space(10)]
public int shield = 0;
public int maxShield = 0;
}
效果如下

TextAreaAttribute
使用高度灵活和可滚动的文本区域编辑字符串的属性。
您可以指定TextArea的最小和最大行,并且字段将根据文本的大小进行扩展。如果文本大于可用区域,则会显示一个滚动条。
using UnityEngine;
public class TextAreaExample : MonoBehaviour
{
[TextArea()]
public string MyTextArea;
}
效果如下

TooltipAttribute
在“检查器”窗口中指定一个字段的工具提示。
using UnityEngine;
using System.Collections;
public class ExampleClass3 : MonoBehaviour
{
[Tooltip("Health 值 从 0 到 100.")]
public int health = 0;
}
效果如下

UnityAPICompatibilityVersionAttribute
声明一个程序集与特定的Unity API兼容(API明智)。由内部工具使用,以避免处理程序集,以确定程序集是否可能使用旧的Unity API。
(目前没弄明白怎么用)