picasso是Square公司开源的一个Android图形缓存库,地址http://square.github.io/picasso/,可以实现图片下载和缓存功能。
picasso使用简单,如下
- Picasso.with(context).load("http://i.imgur.com/DvpvklR.png").into(imageView);
主要有以下一些特性:
- 在adapter中回收和取消当前的下载;
- 使用最少的内存完成复杂的图形转换操作;
- 自动的内存和硬盘缓存;
- 图形转换操作,如变换大小,旋转等,提供了接口来让用户可以自定义转换操作;
- 加载载网络或本地资源;
代码分析
Cache,缓存类
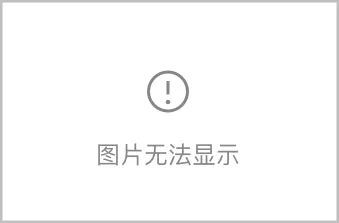
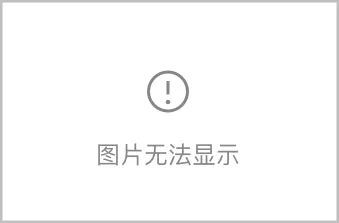
Lrucacha,主要是get和set方法,存储的结构采用了LinkedHashMap,这种map内部实现了lru算法(Least Recently Used 近期最少使用算法)。
最后一个参数的解释:
- this.map = new LinkedHashMap<String, Bitmap>(0, 0.75f, true);
最后一个参数的解释:
true if the ordering should be done based on the last access (from least-recently accessed to most-recently accessed), and false if the ordering should be the order in which the entries were inserted.
因为可能会涉及多线程,所以在存取的时候都会加锁。而且每次set操作后都会判断当前缓存区是否已满,如果满了就清掉最少使用的图形。代码如下
当操作封装好以后,会将Request传到另一个结构中Action。
可以看到,在decode生成原始bitmap,之后会做需要的转换transformResult和applyCustomTransformations。最后在将最终的结果传递到上层dispatcher.dispatchComplete(this)。
Dispatcher内有一个HandlerThread,所有的请求都会通过这个thread转换,也就是请求也是异步的,这样应该是为了Ui线程更加流畅,同时保证请求的顺序,因为handler的消息队列。
外部调用的是dispatchXXX方法,然后通过handler将请求转换到对应的performXXX方法。
例如生成Action以后就会调用dispather的dispatchSubmit()来请求执行,
- private void trimToSize(int maxSize) {
- while (true) {
- String key;
- Bitmap value;
- synchronized (this) {
- if (size < 0 || (map.isEmpty() && size != 0)) {
- throw new IllegalStateException(getClass().getName()
- + ".sizeOf() is reporting inconsistent results!");
- }
- if (size <= maxSize || map.isEmpty()) {
- break;
- }
- Map.Entry<String, Bitmap> toEvict = map.entrySet().iterator()
- .next();
- key = toEvict.getKey();
- value = toEvict.getValue();
- map.remove(key);
- size -= Utils.getBitmapBytes(value);
- evictionCount++;
- }
- }
- }
Request,操作封装类
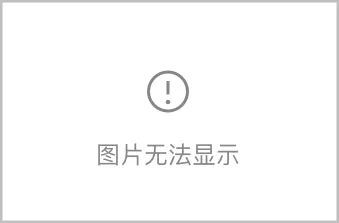
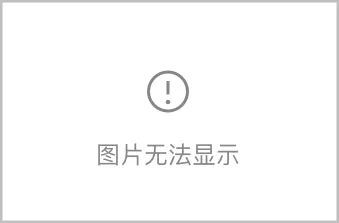
所有对图形的操作都会记录在这里,供之后图形的创建使用,如重新计算大小,旋转角度,也可以自定义变换,只需要实现Transformation,一个bitmap转换的接口。
- public interface Transformation {
- /**
- * Transform the source bitmap into a new bitmap. If you create a new bitmap instance, you must
- * call {@link android.graphics.Bitmap#recycle()} on {@code source}. You may return the original
- * if no transformation is required.
- */
- Bitmap transform(Bitmap source);
- /**
- * Returns a unique key for the transformation, used for caching purposes. If the transformation
- * has parameters (e.g. size, scale factor, etc) then these should be part of the key.
- */
- String key();
- }
当操作封装好以后,会将Request传到另一个结构中Action。
Action
Action代表了一个具体的加载任务,主要用于图片加载后的结果回调,有两个抽象方法,complete和error,也就是当图片解析为bitmap后用户希望做什么。最简单的就是将bitmap设置给imageview,失败了就将错误通过回调通知到上层。
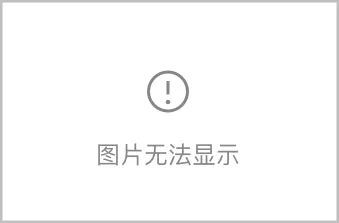
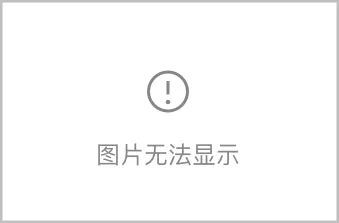
ImageViewAction实现了Action,在complete中将bitmap和imageview组成了一个PicassoDrawable,里面会实现淡出的动画效果。
有了加载任务,具体的图片下载与解析是在哪里呢?这些都是耗时的操作,应该放在异步线程中进行,就是下面的BitmapHunter。
- @Override
- public void complete(Bitmap result, Picasso.LoadedFrom from) {
- if (result == null) {
- throw new AssertionError(String.format(
- "Attempted to complete action with no result!\n%s", this));
- }
- ImageView target = this.target.get();
- if (target == null) {
- return;
- }
- Context context = picasso.context;
- boolean debugging = picasso.debugging;
- PicassoDrawable.setBitmap(target, context, result, from, noFade,
- debugging);
- if (callback != null) {
- callback.onSuccess();
- }
- }
有了加载任务,具体的图片下载与解析是在哪里呢?这些都是耗时的操作,应该放在异步线程中进行,就是下面的BitmapHunter。
BitmapHunter
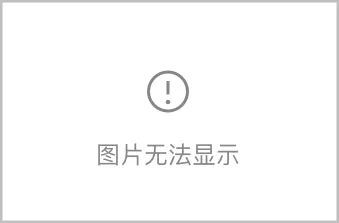
BitmapHunter是一个Runnable,其中有一个decode的抽象方法,用于子类实现不同类型资源的解析。
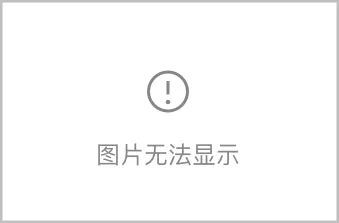
- @Override
- public void run() {
- try {
- Thread.currentThread()
- .setName(Utils.THREAD_PREFIX + data.getName());
- result = hunt();
- if (result == null) {
- dispatcher.dispatchFailed(this);
- } else {
- dispatcher.dispatchComplete(this);
- }
- } catch (IOException e) {
- exception = e;
- dispatcher.dispatchRetry(this);
- } catch (Exception e) {
- exception = e;
- dispatcher.dispatchFailed(this);
- } finally {
- Thread.currentThread().setName(Utils.THREAD_IDLE_NAME);
- }
- }
- abstract Bitmap decode(Request data) throws IOException;
- Bitmap hunt() throws IOException {
- Bitmap bitmap;
- if (!skipMemoryCache) {
- bitmap = cache.get(key);
- if (bitmap != null) {
- stats.dispatchCacheHit();
- loadedFrom = MEMORY;
- return bitmap;
- }
- }
- bitmap = decode(data);
- if (bitmap != null) {
- stats.dispatchBitmapDecoded(bitmap);
- if (data.needsTransformation() || exifRotation != 0) {
- synchronized (DECODE_LOCK) {
- if (data.needsMatrixTransform() || exifRotation != 0) {
- bitmap = transformResult(data, bitmap, exifRotation);
- }
- if (data.hasCustomTransformations()) {
- bitmap = applyCustomTransformations(
- data.transformations, bitmap);
- }
- }
- stats.dispatchBitmapTransformed(bitmap);
- }
- }
- return bitmap;
- }
可以看到,在decode生成原始bitmap,之后会做需要的转换transformResult和applyCustomTransformations。最后在将最终的结果传递到上层dispatcher.dispatchComplete(this)。
基本的组成元素有了,那这一切是怎么连接起来运行呢,答案是Dispatcher。
Dispatcher任务调度器
在bitmaphunter成功得到bitmap后,就是通过dispatcher将结果传递出去的,当然让bitmaphunter执行也要通过Dispatcher。
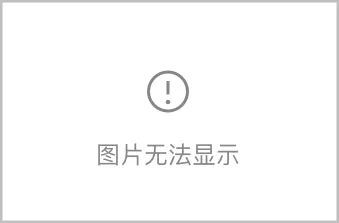
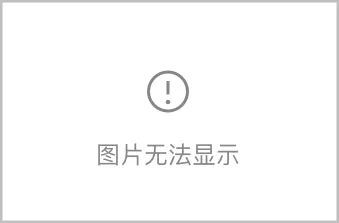
外部调用的是dispatchXXX方法,然后通过handler将请求转换到对应的performXXX方法。
例如生成Action以后就会调用dispather的dispatchSubmit()来请求执行,
- void dispatchSubmit(Action action) {
- handler.sendMessage(handler.obtainMessage(REQUEST_SUBMIT, action));
- }
handler接到消息后转换到performSubmit方法
- void performSubmit(Action action) {
- BitmapHunter hunter = hunterMap.get(action.getKey());
- if (hunter != null) {
- hunter.attach(action);
- return;
- }
- if (service.isShutdown()) {
- return;
- }
- hunter = forRequest(context, action.getPicasso(), this, cache, stats,
- action, downloader);
- hunter.future = service.submit(hunter);
- hunterMap.put(action.getKey(), hunter);
- }
这里将通过action得到具体的BitmapHunder,然后交给ExecutorService执行。
下面是Picasso.with(context).load("http://i.imgur.com/DvpvklR.png").into(imageView)的过程,
- public static Picasso with(Context context) {
- if (singleton == null) {
- singleton = new Builder(context).build();
- }
- return singleton;
- }
- public Picasso build() {
- Context context = this.context;
- if (downloader == null) {
- downloader = Utils.createDefaultDownloader(context);
- }
- if (cache == null) {
- cache = new LruCache(context);
- }
- if (service == null) {
- service = new PicassoExecutorService();
- }
- if (transformer == null) {
- transformer = RequestTransformer.IDENTITY;
- }
- Stats stats = new Stats(cache);
- Dispatcher dispatcher = new Dispatcher(context, service, HANDLER,
- downloader, cache, stats);
- return new Picasso(context, dispatcher, cache, listener,
- transformer, stats, debugging);
- }
在Picasso.with()的时候会将执行所需的所有必备元素创建出来,如缓存cache、执行executorService、调度dispatch等,在load()时创建Request,在into()中创建action、bitmapHunter,并最终交给dispatcher执行。