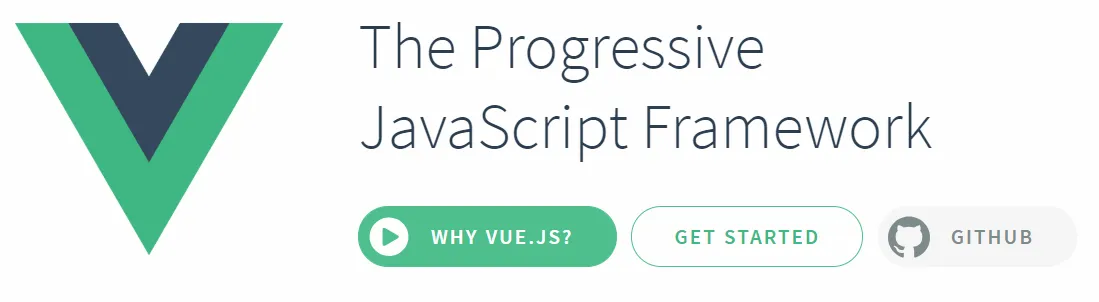
Vue.js
Vue.js 2.0
每天进步一点点,你终将会变成你想要成为的那个人
VueDemo_01:初识vue
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Vue01</title>
<script src="js/vue.min.js"></script>
<script>
window.onload = function () {
//创建一个实例
new Vue({
//element 这里面放‘选择器 ’
el:'#app',
//data 也是固定的名字 这里面放置数据
data:{
message:"我的第一个vue例子"
}
})
}
</script>
</head>
<body>
<div id="app">
<p>{{ message }}</p>
<input type="text" v-model="message">
</div>
</body>
</html>
new Vue
:创建一个Vue实例(对象),可以起名字也可以不起el
:element 的缩写 这里面放‘选择器 ’data
:data 也是固定的名字 这里面放置数据v-model="message"
:指令 v-model
用于数据绑定{{ message }}
:取出数据
VueDemo_02:data
data
:数据类型可以有很多种...
<script src="js/vue.min.js"></script>
<script>
window.onload = function () {
//创建一个实例
new Vue({
//element 这里面放‘选择器 ’
el:'#app',
//data 也是固定的名字 这里面放置数据
data:{
message:"我的第一个vue例子",
arr:["Spring","Hibernate","SpringBoot","Mybatis"],
jsonObj:{a:"Spring",b:"Hibernate",c:"SpringBoot",d:"Mybatis"}
}
})
}
</script>
v-for
指令:循环取值v-for="(value,index) in arr">{{value}} {{index}}
:v-for="(json,index,key) in jsonObj">{{json}} {{index}}{{key}}
:value
:data中的数据名称index
:索引key
:json的key
<body>
<div id="app">
<!-- v-for -->
<ul >
<li v-for="(value,index) in arr">{{value}} {{index}}</li>
</ul>
<ol>
<li v-for="(json,index,key) in jsonObj">{{json}} {{index}}{{key}}</li>
</ol>
</div>
</body>
说明一下:vue 2.x开始 删除了 v-for 指令当中的 $index如果需要使用 需要在 v-for 指令当中添加需要的参数(就是要按照上面的格式书写)
VueDemo_03:Vue中的事件
v-on:click
:点击事件
<body>
<!-- v-on:click-->
<div id="app">
<input type="button" value="按钮" v-on:click="show()">
<ul>
<li v-for="value in arr">{{value}}</li>
</ul>
</div>
</body>
methods:
这个参数也是固定的 事件方法在这里面定义
<script src="js/vue.min.js"></script>
<script>
window.onload = function () {
//创建一个实例
new Vue({
//element 这里面放‘选择器 ’
el:'#app',
//data 也是固定的名字 这里面放置数据
data:{
message:"我的第一个vue例子",
arr:["Spring","Hibernate","SpringBoot","Mybatis"],
jsonObj:{a:"Spring",b:"Hibernate",c:"SpringBoot",d:"Mybatis"}
},
methods:{
show:function () {
alert("弹出框");
}
}
})
}
</script>
VueDemo_04:一个添加的点击事件
<script src="js/vue.min.js"></script>
<script>
window.onload = function () {
//创建一个实例
new Vue({
//element 这里面放‘选择器 ’
el:'#app',
//data 也是固定的名字 这里面放置数据
data:{
message:"我的第一个vue例子",
arr:["Spring","Hibernate","SpringBoot","Mybatis"],
jsonObj:{a:"Spring",b:"Hibernate",c:"SpringBoot",d:"Mybatis"}
},
methods:{
add:function () {
this.arr.push("SpringMVC");
}
}
})
}
</script>
因为数据是双向绑定的,所以在点击按钮之后页面上展示的数组的数据也会随之增加
<!-- v-on:click-->
<div id="app">
<input type="button" value="按钮" v-on:click="add()">
<ul>
<li v-for="value in arr">{{value}}</li>
</ul>
</div>