四.案例分析(Example)
1、场景
使用抽象工厂
+
反射
+
配置文件实现数据访问层程序。结构
如下图所示
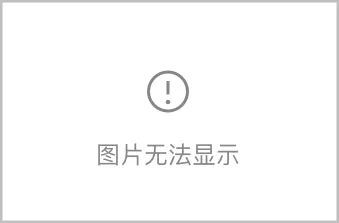
用反射
+
抽象工厂
+
配置文件的数据访问程序。
Assembly.Load("
程序集名称
").CreateInstance("
命名空间
.
类名称
")
。比如:
IProduct product=(IProduct)Assembly.Load("
抽象工程模式
").CreateInstance("
抽象工程模式
.SqlServerProduct")
。
常用做法是:
Private static readonly string AssemblyName="
抽象工程模式
";
Private static readonly string DB=ConfiurationManager.AppSettings["db"];
配置文件如下:
<configuration>
<appSettings>
<add key="db" value="Sqlserver"/>
<appSettings>
<configuration>
通过读配置文件给
DB
字符赋值,在配置文件中写明当前使用的是
SqlServer
还是
Access
数据库。反射
+
抽象工厂
+
配置文件解决方案解决了数据访问时的可维护、可扩展问题
2、代码
1
、对象
Uer
、
Product
及其相对应的操作
|
public
interface IUser
{
void
Insert();
}
public
class SqlServerUser
:IUser
{
public void
Insert()
{
Console
.WriteLine("{0}
插入用户
."
,this
.GetType().Name);
}
}
public
class AccessUser
: IUser
{
public void
Insert()
{
Console
.WriteLine("{0}
插入用户
."
, this
.GetType().Name);
}
}
public
interface IProduct
{
void
GetProduct();
}
public
class SqlServerProduct
: IProduct
{
public void
GetProduct()
{
Console
.WriteLine("{0}
查询商品
."
, this
.GetType().Name);
}
}
public
class AccessProduct
: IProduct
{
public void
GetProduct()
{
Console
.WriteLine("{0}
查询商品
."
, this
.GetType().Name);
}
}
|
2
、数据访问类
DataAccess
|
public
class DataAccess
{
private static readonly string
AssemblyName = "AbstractFactoryReflection"
;
private static readonly string
db = "SqlServer"
;
public static IUser
CreateUser()
{
string
className = AssemblyName + "."
+ db + "User"
;
IUser
user = (IUser
)Assembly
.Load(AssemblyName).CreateInstance(className);
return
user;
}
public static IProduct
CreateProduct()
{
string
className = AssemblyName + "."
+ db + "Product"
;
return
(IProduct
)Assembly
.Load(AssemblyName).CreateInstance(className);
}
}
|
3
、客户端代码
|
static
void
{
IUser
user = DataAccess
.CreateUser();
user.Insert();
IProduct
product = DataAccess
.CreateProduct();
product.GetProduct();
Console
.ReadKey();
}
|
五、总结(Summary)
抽象工厂模式(
Abstract Factory Pattern
),提供一个创建一系列相关或者相互依赖对象的接口,而无需制定他们的具体类。抽象工厂模式的典型应用就是,使用抽象工厂
+
反射
+
配置文件实现数据访问层程序。
本文转自 灵动生活 51CTO博客,原文链接:http://blog.51cto.com/smartlife/260399,如需转载请自行联系原作者