今天有空接着Qt创建主窗口File菜单的实现,创建主窗口对于我来说确实有些难度,平时不努力学C/C++,现在从头开始很费劲.现在感慨,书到用时方恨少呀。接下来做一个简单的文本编辑器,给文本编辑器添加信号与槽.前面子类化QMainWindow、菜单栏和工具栏、状态栏都做好了。接下来实现文件菜单的信号与槽的链接。
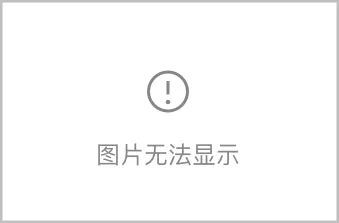
先实现菜单栏中的菜单栏中文件栏的exit动作和帮助栏中的信号与槽,这是最简单的。帮助栏一个是关于,一个是Qt版本信息。
about要建立创建一个about()函数,用来显现一个带有标题,文本内容的的about消息框,这个about消息框父控件是自己本身。About()函数寻找图标是通过icon()函数查找,作为最后使用的信息图标。这里要用到上一篇QMessageBox创建消息框的知识,而about Qt直接用QApplication调用Qt图形化应用程序的qApp对象来实现,qApp是一个全局指针指的是独立的应用对象。而退出动作也是,直接调用qApp中的closeAllWindows()函数来实现。在mainwindow.h头文件类MainWindow主窗口对象里添加Q_OBJECT信号和槽的一个宏定义。定义一个私有信号槽about()函数,当点击about时,触发触发器发出信号,给链接的制定的about槽函数,然后实现对于主窗口about()函数的功能。点击信号与槽链接在mainwindow.cppC++源文件中定义,运行效果如下:
connect(exitAction, SIGNAL(triggered()), qApp, SLOT(closeAllWindows()));
connect(aboutQtAction, SIGNAL(triggered()), qApp, SLOT(aboutQt()));
connect(aboutAction, SIGNAL(triggered()), this, SLOT(about()));
void MainWindow::about()
{
QMessageBox::about(this, tr("About Recent Files"),
tr("The <b>Recent Files</b> example demonstrates how to provide a "
"recently used file menu in a Qt application."));
}
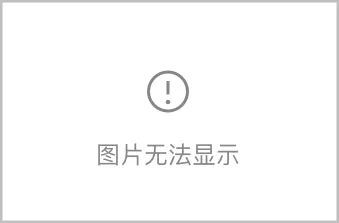
接着实现新建文件的功能,其实这个也蛮简单的,在mainwindow.h头文件中声明newFile()函数,在mainwindow.cpp文件中添加信号与槽的链接。
connect(newAction, SIGNAL(triggered()), this, SLOT(newFile()));
下面是newFile()函数实现的功能,创建一个主窗口对象,将主窗口对象窗口部件显示出来,当点击new是,触发新建触发器发出信号到指定的槽中,然后调用newFile函数来实现新建一个主窗口功能。当想要关闭所有的主窗口时,先关闭打开的消息框,点击File栏中的exit退出或直接Ctrl+Q即可。
void MainWindow::newFile()
{
MainWindow *other = new MainWindow;
other->show();
}
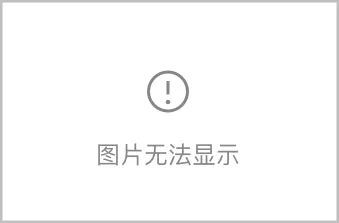
先定义字符串strippedName()函数,用字符创strippedName函数调用窗口部件设置标题setWindowTitle()函数周围的文件名来缩短排除路径。在函数里面构造一个新的QFileInfo()文件信息函数,获取特定文件的相关信息。该文件包含绝对路径或相对路径。为什么我先定义这个strippedName()函数呢?因为当你创建open()函数这个动作链接信号与槽,都会用到这个函数。依此根据错误提示,找到的最后面的函数就是strippedName()函数。然后根据错误提示,反过来添加其所有满足的函数功能。接着就是创建更新最近文件数据动作updateRecentFileActions()函数,在创建函数前先声明一个枚举类型构造函数,给枚举类型MaxRecentFile赋值。然后定义一个更新文件的指针数组动作变量。在主函数中用createActions()函数中用for循环来记录文件文件,用setVisible()显示文件。最后添加设置setCurrentFile()函数。在声明设置当前文件函数前前,先定义一个字符串变量,curfile.用来获取文件名的字符串变量。接着就可以添加其他功能了,比如打开、另存、保存。如果有剪切、复制、粘贴功能都可以添加。如果有做程序员想法,可以研究一下代码。很难讲的能面面俱全的。接下来实现打开功能,声明一个下载文件函数loadFile()用来判断文件的权限,获取文件名等,然后添加open()函数来实现打开文件,书写代码的顺序已经说了,在头文件声明所要用到的类、函数、对象;在源文件调用函数实现具体功能:
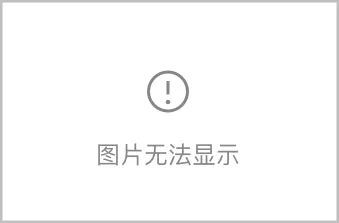
接下来添加保存功能,另存功能是一样道理的。先添加保存文件saveFile()函数,在添加saveAs()函数,在添加save()函数。运行效果如下:
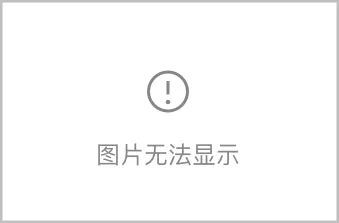
最后实现打开文件的记录功能,在头文件中添加void openRecentFile()信号槽,在源文件中调用openRecentFile()实现具体的功能。然后在createActions()函数中,添加信号与槽的的链接。
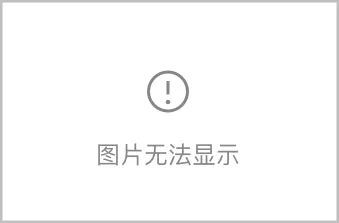
mainwindow.h头文件
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow();
private slots:
void newFile();
void open();
void save();
void saveAs();
void openRecentFile();
void about();
private:
void createActions();
void createMenus();
void createToolBars();
void createStatusBar();
void saveFile(const QString &fileName);
void loadFile(const QString &fileName);
void setCurrentFile(const QString &fileName);
void updateRecentFileActions();
QString strippedName(const QString &fullFileName);
QString curFile;
QTextEdit *textEdit;
QLabel *locationLabel;
QMenu *fileMenu;
QMenu *recentFilesMenu;
QMenu *helpMenu;
QToolBar *fileToolBar;
QAction *newAction;
QAction *openAction;
QAction *saveAction;
QAction *saveAsAction;
QAction *exitAction;
QAction *aboutAction;
QAction *aboutQtAction;
QAction *separatorAction;
enum { MaxRecentFiles = 5 };
QAction *recentFileActions[MaxRecentFiles];
};
{
Q_OBJECT
public:
MainWindow();
private slots:
void newFile();
void open();
void save();
void saveAs();
void openRecentFile();
void about();
private:
void createActions();
void createMenus();
void createToolBars();
void createStatusBar();
void saveFile(const QString &fileName);
void loadFile(const QString &fileName);
void setCurrentFile(const QString &fileName);
void updateRecentFileActions();
QString strippedName(const QString &fullFileName);
QString curFile;
QTextEdit *textEdit;
QLabel *locationLabel;
QMenu *fileMenu;
QMenu *recentFilesMenu;
QMenu *helpMenu;
QToolBar *fileToolBar;
QAction *newAction;
QAction *openAction;
QAction *saveAction;
QAction *saveAsAction;
QAction *exitAction;
QAction *aboutAction;
QAction *aboutQtAction;
QAction *separatorAction;
enum { MaxRecentFiles = 5 };
QAction *recentFileActions[MaxRecentFiles];
};
mainwindow.cpp源文件
MainWindow::MainWindow()
{
textEdit = new QTextEdit;
setCentralWidget(textEdit);
{
textEdit = new QTextEdit;
setCentralWidget(textEdit);
createActions();
createMenus();
createToolBars();
createStatusBar();
createMenus();
createToolBars();
createStatusBar();
setWindowTitle(tr("Text Edit"));
resize(500, 400);
}
resize(500, 400);
}
void MainWindow::newFile()
{
MainWindow *other = new MainWindow;
other->show();
}
{
MainWindow *other = new MainWindow;
other->show();
}
void MainWindow::open()
{
QString fileName = QFileDialog::getOpenFileName(this);
if (!fileName.isEmpty())
loadFile(fileName);
}
void MainWindow::save()
{
if (curFile.isEmpty())
saveAs();
else
saveFile(curFile);
}
{
QString fileName = QFileDialog::getOpenFileName(this);
if (!fileName.isEmpty())
loadFile(fileName);
}
void MainWindow::save()
{
if (curFile.isEmpty())
saveAs();
else
saveFile(curFile);
}
void MainWindow::saveAs()
{
QString fileName = QFileDialog::getSaveFileName(this);
if (fileName.isEmpty())
return;
{
QString fileName = QFileDialog::getSaveFileName(this);
if (fileName.isEmpty())
return;
saveFile(fileName);
}
}
void MainWindow::openRecentFile()
{
QAction *action = qobject_cast<QAction *>(sender());
if (action)
loadFile(action->data().toString());
}
{
QAction *action = qobject_cast<QAction *>(sender());
if (action)
loadFile(action->data().toString());
}
void MainWindow::about()
{
QMessageBox::about(this, tr("About Recent Files"),
tr("The <b>Recent Files</b> example demonstrates how to provide a "
"recently used file menu in a Qt application."));
}
{
QMessageBox::about(this, tr("About Recent Files"),
tr("The <b>Recent Files</b> example demonstrates how to provide a "
"recently used file menu in a Qt application."));
}
void MainWindow::createActions()
{
newAction = new QAction(tr("&New"), this);
newAction->setIcon(QIcon(":/images/new.png"));
newAction->setShortcut(tr("Ctrl+N"));
newAction->setStatusTip(tr("Create a new file"));
connect(newAction, SIGNAL(triggered()), this, SLOT(newFile()));
openAction = new QAction(tr("&Open..."), this);
openAction->setIcon(QIcon(":/images/open.png"));
openAction->setShortcut(tr("Ctrl+O"));
openAction->setStatusTip(tr("Open an existing file"));
connect(openAction, SIGNAL(triggered()), this, SLOT(open()));
openAction->setIcon(QIcon(":/images/open.png"));
openAction->setShortcut(tr("Ctrl+O"));
openAction->setStatusTip(tr("Open an existing file"));
connect(openAction, SIGNAL(triggered()), this, SLOT(open()));
saveAction = new QAction(tr("&Save"), this);
saveAction->setIcon(QIcon(":/images/save.png"));
saveAction->setShortcut(tr("Ctrl+S"));
saveAction->setStatusTip(tr("Save the document to disk"));
connect(saveAction, SIGNAL(triggered()), this, SLOT(save()));
saveAction->setIcon(QIcon(":/images/save.png"));
saveAction->setShortcut(tr("Ctrl+S"));
saveAction->setStatusTip(tr("Save the document to disk"));
connect(saveAction, SIGNAL(triggered()), this, SLOT(save()));
saveAsAction = new QAction(tr("Save &As..."), this);
saveAsAction->setStatusTip(tr("Save the document under a new name"));
connect(saveAsAction, SIGNAL(triggered()), this, SLOT(saveAs()));
saveAsAction->setStatusTip(tr("Save the document under a new name"));
connect(saveAsAction, SIGNAL(triggered()), this, SLOT(saveAs()));
for (int i = 0; i < MaxRecentFiles; ++i) {
recentFileActions[i] = new QAction(this);
recentFileActions[i]->setVisible(false);
connect(recentFileActions[i], SIGNAL(triggered()),
this, SLOT(openRecentFile()));
}
recentFileActions[i] = new QAction(this);
recentFileActions[i]->setVisible(false);
connect(recentFileActions[i], SIGNAL(triggered()),
this, SLOT(openRecentFile()));
}
exitAction = new QAction(tr("E&xit"), this);
exitAction->setShortcut(tr("Ctrl+Q"));
exitAction->setStatusTip(tr("Exit the application"));
connect(exitAction,SIGNAL(triggered()),qApp,SLOT(closeAllWindows()));
exitAction->setShortcut(tr("Ctrl+Q"));
exitAction->setStatusTip(tr("Exit the application"));
connect(exitAction,SIGNAL(triggered()),qApp,SLOT(closeAllWindows()));
aboutAction = new QAction(tr("&About"), this);
aboutAction->setStatusTip(tr("Show the application's About box"));
connect(aboutAction, SIGNAL(triggered()),this,SLOT(about()));
aboutAction->setStatusTip(tr("Show the application's About box"));
connect(aboutAction, SIGNAL(triggered()),this,SLOT(about()));
aboutQtAction = new QAction(tr("About &Qt"), this);
aboutQtAction->setStatusTip(tr("Show the Qt library's About box"));
connect(aboutQtAction,SIGNAL(triggered()),qApp,SLOT(aboutQt()));
}
aboutQtAction->setStatusTip(tr("Show the Qt library's About box"));
connect(aboutQtAction,SIGNAL(triggered()),qApp,SLOT(aboutQt()));
}
void MainWindow::createMenus()
{
fileMenu = menuBar()->addMenu(tr("&File"));
fileMenu->addAction(newAction);
fileMenu->addAction(openAction);
fileMenu->addAction(saveAction);
fileMenu->addAction(saveAsAction);
separatorAction = fileMenu->addSeparator();
for (int i = 0; i < MaxRecentFiles; ++i)
fileMenu->addAction(recentFileActions[i]);
fileMenu->addSeparator();
fileMenu->addAction(exitAction);
{
fileMenu = menuBar()->addMenu(tr("&File"));
fileMenu->addAction(newAction);
fileMenu->addAction(openAction);
fileMenu->addAction(saveAction);
fileMenu->addAction(saveAsAction);
separatorAction = fileMenu->addSeparator();
for (int i = 0; i < MaxRecentFiles; ++i)
fileMenu->addAction(recentFileActions[i]);
fileMenu->addSeparator();
fileMenu->addAction(exitAction);
menuBar()->addSeparator();
helpMenu = menuBar()->addMenu(tr("&Help"));
helpMenu->addAction(aboutAction);
helpMenu->addAction(aboutQtAction);
}
helpMenu->addAction(aboutAction);
helpMenu->addAction(aboutQtAction);
}
void MainWindow::createToolBars()
{
fileToolBar = addToolBar(tr("&File"));
fileToolBar->addAction(newAction);
fileToolBar->addAction(openAction);
fileToolBar->addAction(saveAction);
}
{
fileToolBar = addToolBar(tr("&File"));
fileToolBar->addAction(newAction);
fileToolBar->addAction(openAction);
fileToolBar->addAction(saveAction);
}
void MainWindow::createStatusBar()
{
locationLabel = new QLabel(" ready ");
locationLabel->setAlignment(Qt::AlignHCenter);
locationLabel->setMinimumSize(locationLabel->sizeHint());
statusBar()->addWidget(locationLabel);
{
locationLabel = new QLabel(" ready ");
locationLabel->setAlignment(Qt::AlignHCenter);
locationLabel->setMinimumSize(locationLabel->sizeHint());
statusBar()->addWidget(locationLabel);
}
void MainWindow::loadFile(const QString &fileName)
{
QFile file(fileName);
if (!file.open(QFile::ReadOnly | QFile::Text)) {
QMessageBox::warning(this, tr("Recent Files"),
tr("Cannot read file %1:\n%2.")
.arg(fileName)
.arg(file.errorString()));
return;
}
{
QFile file(fileName);
if (!file.open(QFile::ReadOnly | QFile::Text)) {
QMessageBox::warning(this, tr("Recent Files"),
tr("Cannot read file %1:\n%2.")
.arg(fileName)
.arg(file.errorString()));
return;
}
QTextStream in(&file);
QApplication::setOverrideCursor(Qt::WaitCursor);
textEdit->setPlainText(in.readAll());
QApplication::restoreOverrideCursor();
QApplication::setOverrideCursor(Qt::WaitCursor);
textEdit->setPlainText(in.readAll());
QApplication::restoreOverrideCursor();
setCurrentFile(fileName);
statusBar()->showMessage(tr("File loaded"), 2000);
}
statusBar()->showMessage(tr("File loaded"), 2000);
}
void MainWindow::saveFile(const QString &fileName)
{
QFile file(fileName);
if (!file.open(QFile::WriteOnly | QFile::Text)) {
QMessageBox::warning(this, tr("Recent Files"),
tr("Cannot write file %1:\n%2.")
.arg(fileName)
.arg(file.errorString()));
return;
}
{
QFile file(fileName);
if (!file.open(QFile::WriteOnly | QFile::Text)) {
QMessageBox::warning(this, tr("Recent Files"),
tr("Cannot write file %1:\n%2.")
.arg(fileName)
.arg(file.errorString()));
return;
}
QTextStream out(&file);
QApplication::setOverrideCursor(Qt::WaitCursor);
out << textEdit->toPlainText();
QApplication::restoreOverrideCursor();
QApplication::setOverrideCursor(Qt::WaitCursor);
out << textEdit->toPlainText();
QApplication::restoreOverrideCursor();
setCurrentFile(fileName);
statusBar()->showMessage(tr("File saved"), 2000);
}
statusBar()->showMessage(tr("File saved"), 2000);
}
void MainWindow::setCurrentFile(const QString &fileName)
{
curFile = fileName;
if (curFile.isEmpty())
setWindowTitle(tr("Recent Files"));
else
setWindowTitle(tr("%1 - %2").arg(strippedName(curFile))
.arg(tr("Recent Files")));
{
curFile = fileName;
if (curFile.isEmpty())
setWindowTitle(tr("Recent Files"));
else
setWindowTitle(tr("%1 - %2").arg(strippedName(curFile))
.arg(tr("Recent Files")));
QSettings settings("Trolltech", "Recent Files Example");
QStringList files = settings.value("recentFileList").toStringList();
files.removeAll(fileName);
files.prepend(fileName);
while (files.size() > MaxRecentFiles)
files.removeLast();
QStringList files = settings.value("recentFileList").toStringList();
files.removeAll(fileName);
files.prepend(fileName);
while (files.size() > MaxRecentFiles)
files.removeLast();
settings.setValue("recentFileList", files);
foreach (QWidget *widget, QApplication::topLevelWidgets()) {
MainWindow *mainWin = qobject_cast<MainWindow *>(widget);
if (mainWin)
mainWin->updateRecentFileActions();
}
}
MainWindow *mainWin = qobject_cast<MainWindow *>(widget);
if (mainWin)
mainWin->updateRecentFileActions();
}
}
void MainWindow::updateRecentFileActions()
{
QSettings settings("Trolltech", "Recent Files Example");
QStringList files = settings.value("recentFileList").toStringList();
{
QSettings settings("Trolltech", "Recent Files Example");
QStringList files = settings.value("recentFileList").toStringList();
int numRecentFiles = qMin(files.size(), (int)MaxRecentFiles);
for (int i = 0; i < numRecentFiles; ++i) {
QString text = tr("&%1 %2").arg(i + 1).arg(strippedName(files[i]));
recentFileActions[i]->setText(text);
recentFileActions[i]->setData(files[i]);
recentFileActions[i]->setVisible(true);
}
for (int j = numRecentFiles; j < MaxRecentFiles; ++j)
recentFileActions[j]->setVisible(false);
QString text = tr("&%1 %2").arg(i + 1).arg(strippedName(files[i]));
recentFileActions[i]->setText(text);
recentFileActions[i]->setData(files[i]);
recentFileActions[i]->setVisible(true);
}
for (int j = numRecentFiles; j < MaxRecentFiles; ++j)
recentFileActions[j]->setVisible(false);
separatorAction->setVisible(numRecentFiles > 0);
}
}
QString MainWindow::strippedName(const QString &fullFileName)
{
return QFileInfo(fullFileName).fileName();
}
{
return QFileInfo(fullFileName).fileName();
}
嘿嘿...感觉到搞程序的很累...
本文转自 chen138 51CTO博客,原文链接:http://blog.51cto.com/chenboqiang/324957,如需转载请自行联系原作者