Knight Moves
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions:20913 | Accepted: 9702 |
Description
Background
Mr Somurolov, fabulous chess-gamer indeed, asserts that no one else but him can move knights from one position to another so fast. Can you beat him?
The Problem
Your task is to write a program to calculate the minimum number of moves needed for a knight to reach one point from another, so that you have the chance to be faster than Somurolov.
For people not familiar with chess, the possible knight moves are shown in Figure 1.
Mr Somurolov, fabulous chess-gamer indeed, asserts that no one else but him can move knights from one position to another so fast. Can you beat him?
The Problem
Your task is to write a program to calculate the minimum number of moves needed for a knight to reach one point from another, so that you have the chance to be faster than Somurolov.
For people not familiar with chess, the possible knight moves are shown in Figure 1.
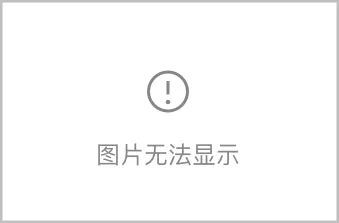
Input
The input begins with the number n of scenarios on a single line by itself.
Next follow n scenarios. Each scenario consists of three lines containing integer numbers. The first line specifies the length l of a side of the chess board (4 <= l <= 300). The entire board has size l * l. The second and third line contain pair of integers {0, ..., l-1}*{0, ..., l-1} specifying the starting and ending position of the knight on the board. The integers are separated by a single blank. You can assume that the positions are valid positions on the chess board of that scenario.
Next follow n scenarios. Each scenario consists of three lines containing integer numbers. The first line specifies the length l of a side of the chess board (4 <= l <= 300). The entire board has size l * l. The second and third line contain pair of integers {0, ..., l-1}*{0, ..., l-1} specifying the starting and ending position of the knight on the board. The integers are separated by a single blank. You can assume that the positions are valid positions on the chess board of that scenario.
Output
For each scenario of the input you have to calculate the minimal amount of knight moves which are necessary to move from the starting point to the ending point. If starting point and ending point are equal,distance is zero. The distance must be written on a single line.
Sample Input
3 8 0 0 7 0 100 0 0 30 50 10 1 1 1 1
Sample Output
5 28 0
#include<iostream> #include<cstdio> #include<queue> #include<cstring> #define maxn 350 using namespace std; int n; int x1,x2,y1,y2; struct node{ int x; int y; int sum; node(int a,int b,int c) { x=a; y=b; sum=c; } }; int go[8][2]={{1,2},{1,-2},{2,1},{2,-1},{-1,2},{-1,-2},{-2,1},{-2,-1}}; int vis[310][310]; int BFS() { queue<node> que; memset(vis,0,sizeof vis); que.push(node(x1,y1,0)); vis[x1][y1]=1; while(!que.empty()) { node temp=que.front(); for(int i=0;i<8;i++) { int x0=temp.x+go[i][0]; int y0=temp.y+go[i][1]; int sum0=temp.sum+1; if(x0>=0&&x0<n&&y0>=0&&y0<n) { if(x0==x2&&y0==y2) return sum0; else if(!vis[x0][y0]) { que.push(node(x0,y0,sum0)); vis[x0][y0]=1; } } } que.pop(); } return 0; } int main() { int t; scanf("%d",&t); while(t--) { scanf("%d%d%d%d%d",&n,&x1,&y1,&x2,&y2); if(x1==x2&&y1==y2) printf("0\n"); else printf("%d\n",BFS()); } return 0; }
版权声明:本文博客原创文章,博客,未经同意,不得转载。
本文转自mfrbuaa博客园博客,原文链接:http://www.cnblogs.com/mfrbuaa/p/4751873.html,如需转载请自行联系原作者