Updated: November 2007
Compares two specified String objects and returns an integer that indicates their relationship to one another in the sort order.
Namespace: System
Assembly: mscorlib (in mscorlib.dll)
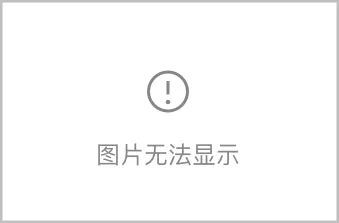
Visual Basic (Declaration)
|
Public Shared Function Compare ( _ strA As String, _ strB As String _ ) As Integer |
Visual Basic (Usage)
|
Dim strA As String Dim strB As String Dim returnValue As Integer returnValue = (strA, _ strB) |
C#
|
public static int Compare( string strA, string strB ) |
Visual C++
|
public: static int Compare( String^ strA, String^ strB ) |
J#
|
public static int Compare( String strA, String strB ) |
JScript
|
public static function Compare( strA : String, strB : String ) : int |
Parameters
- strA
-
Type:
System..::.String
The first String.
- strB
-
Type:
System..::.String
The second String.
Return Value
Type: System..::.Int32A 32-bit signed integer indicating the lexical relationship between the two comparands.
Value |
Condition |
---|---|
Less than zero |
strA is less than strB. |
Zero |
strA equals strB. |
Greater than zero |
strA is greater than strB. |
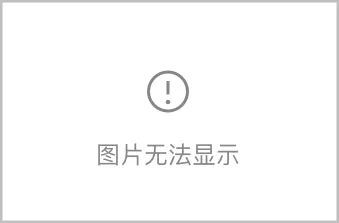
The comparison uses the current culture to obtain culture-specific information such as casing rules and the alphabetic order of individual characters. For example, a culture could specify that certain combinations of characters be treated as a single character, or uppercase and lowercase characters be compared in a particular way, or that the sorting order of a character depends on the characters that precede or follow it.
The comparison is performed using word sort rules. For more information about word, string, and ordinal sorts, see System.Globalization..::.CompareOptions.
One or both comparands can be nullNothingnullptra null reference (Nothing in Visual Basic). By definition, any string, including the empty string (""), compares greater than a null reference; and two null references compare equal to each other.
The comparison terminates when an inequality is discovered or both strings have been compared. However, if the two strings compare equal to the end of one string, and the other string has characters remaining, then the string with remaining characters is considered greater. The return value is the result of the last comparison performed.
Unexpected results can occur when comparisons are affected by culture-specific casing rules. For example, in Turkish, the following example yields the wrong results because the file system in Turkish does not use linguistic casing rules for the letter 'i' in "file".
C#
|
static bool IsFileURI(String path) { return ((path, 0, "file:", 0, 5, true) == 0); } |
Visual Basic
|
Shared Function IsFileURI(ByVal path As String) As Boolean If (path, 0, "file:", 0, 5, True) = 0 Then Return True Else Return False End If End Function |
Compare the path name to "file" using an ordinal comparison. The correct code to do this is as follows:
C#
|
static bool IsFileURI(String path) { return ((path, 0, "file:", 0, 5, StringComparison.OrdinalIgnoreCase) == 0); } |
Visual Basic
|
Shared Function IsFileURI(ByVal path As String) As Boolean If (path, 0, "file:", 0, 5, StringComparison.OrdinalIgnoreCase) = 0 Then Return True Else Return False End If End Function |
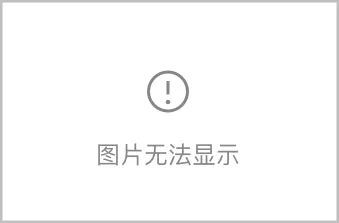
In the following code example, the ReverseStringComparer class demonstrates how you can evaluate two strings with the Compare method.
Visual Basic
|
Imports System Imports System.Text Imports System.Collections Public Class SamplesArrayList Public Shared Sub Main() Dim myAL As New ArrayList() ' Creates and initializes a new ArrayList. myAL.Add("Eric") myAL.Add("Mark") myAL.Add("Lance") myAL.Add("Rob") myAL.Add("Kris") myAL.Add("Brad") myAL.Add("Kit") myAL.Add("Bradley") myAL.Add("Keith") myAL.Add("Susan") ' Displays the properties and values of the ArrayList. Console.WriteLine("Count: {0}", myAL.Count) PrintValues("Unsorted", myAL) myAL.Sort() PrintValues("Sorted", myAL) Dim comp as New ReverseStringComparer myAL.Sort(comp) PrintValues("Reverse", myAL) Dim names As String() = CType(myAL.ToArray(GetType(String)), String()) End Sub 'Main Public Shared Sub PrintValues(title As String, myList As IEnumerable) Console.Write("{0,10}: ", title) Dim sb As New StringBuilder() Dim s As String For Each s In myList sb.AppendFormat("{0}, ", s) Next s sb.Remove(sb.Length - 2, 2) Console.WriteLine(sb) End Sub 'PrintValues End Class 'SamplesArrayList Public Class ReverseStringComparer Implements IComparer Function Compare(x As Object, y As Object) As Integer implements IComparer.Compare Dim s1 As String = CStr (x) Dim s2 As String = CStr (y) 'negate the return value to get the reverse order Return - [String].Compare(s1, s2) End Function 'Compare End Class 'ReverseStringComparer |
C#
|
using System; using System.Text; using System.Collections; public class SamplesArrayList { public static void Main() { // Creates and initializes a new ArrayList. ArrayList myAL = new ArrayList(); myAL.Add("Eric"); myAL.Add("Mark"); myAL.Add("Lance"); myAL.Add("Rob"); myAL.Add("Kris"); myAL.Add("Brad"); myAL.Add("Kit"); myAL.Add("Bradley"); myAL.Add("Keith"); myAL.Add("Susan"); // Displays the properties and values of the ArrayList. Console.WriteLine( "Count: {0}", myAL.Count ); PrintValues ("Unsorted", myAL ); myAL.Sort(); PrintValues("Sorted", myAL ); myAL.Sort(new ReverseStringComparer() ); PrintValues ("Reverse" , myAL ); string [] names = (string[]) myAL.ToArray (typeof(string)); } public static void PrintValues(string title, IEnumerable myList ) { Console.Write ("{0,10}: ", title); StringBuilder sb = new StringBuilder(); foreach (string s in myList) { sb.AppendFormat( "{0}, ", s); } sb.Remove (sb.Length-2,2); Console.WriteLine(sb); } } public class ReverseStringComparer : IComparer { public int Compare (object x, object y) { string s1 = x as string; string s2 = y as string; //negate the return value to get the reverse order return - (s1,s2); } } |
Visual C++
|
using namespace System; using namespace System::Text; using namespace System::Collections; ref class ReverseStringComparer: public IComparer { public: virtual int Compare( Object^ x, Object^ y ) { String^ s1 = dynamic_cast<String^>(x); String^ s2 = dynamic_cast<String^>(y); //negate the return value to get the reverse order return -String::Compare( s1, s2 ); } }; void PrintValues( String^ title, IEnumerable^ myList ) { Console::Write( "{0,10}: ", title ); StringBuilder^ sb = gcnew StringBuilder; { IEnumerator^ en = myList->GetEnumerator(); String^ s; while ( en->MoveNext() ) { s = en->Current->ToString(); sb->AppendFormat( "{0}, ", s ); } } sb->Remove( sb->Length - 2, 2 ); Console::WriteLine( sb ); } void main() { // Creates and initializes a new ArrayList. ArrayList^ myAL = gcnew ArrayList; myAL->Add( "Eric" ); myAL->Add( "Mark" ); myAL->Add( "Lance" ); myAL->Add( "Rob" ); myAL->Add( "Kris" ); myAL->Add( "Brad" ); myAL->Add( "Kit" ); myAL->Add( "Bradley" ); myAL->Add( "Keith" ); myAL->Add( "Susan" ); // Displays the properties and values of the ArrayList. Console::WriteLine( "Count: {0}", myAL->Count.ToString() ); PrintValues( "Unsorted", myAL ); myAL->Sort(); PrintValues( "Sorted", myAL ); myAL->Sort( gcnew ReverseStringComparer ); PrintValues( "Reverse", myAL ); array<String^>^names = dynamic_cast<array<String^>^>(myAL->ToArray( String::typeid )); } |
J#
|
import System.*; import System.Text.*; import System.Collections.*; public class SamplesArrayList { public static void main(String[] args) { // Creates and initializes a new ArrayList. ArrayList myAL = new ArrayList(); myAL.Add("Eric"); myAL.Add("Mark"); myAL.Add("Lance"); myAL.Add("Rob"); myAL.Add("Kris"); myAL.Add("Brad"); myAL.Add("Kit"); myAL.Add("Bradley"); myAL.Add("Keith"); myAL.Add("Susan"); // Displays the properties and values of the ArrayList. Console.WriteLine("Count: {0}", (Int32)myAL.get_Count()); PrintValues("Unsorted", myAL); myAL.Sort(); PrintValues("Sorted", myAL); myAL.Sort(new ReverseStringComparer()); PrintValues("Reverse", myAL); String names[] = (String[])(myAL.ToArray(String.class.ToType())); } //main public static void PrintValues(String title, IEnumerable myList) { Console.Write("{0,10}: ", title); StringBuilder sb = new StringBuilder(); IEnumerator objEnum = myList.GetEnumerator(); while (objEnum.MoveNext()) { String s = System.Convert.ToString(objEnum.get_Current()); sb.AppendFormat("{0}, ", s); } sb.Remove(sb.get_Length() - 2, 2); Console.WriteLine(sb); } //PrintValues } //SamplesArrayList public class ReverseStringComparer implements IComparer { public int Compare(Object x, Object y) { String s1 = System.Convert.ToString(x); String s2 = System.Convert.ToString(y); //negate the return value to get the reverse order return -(s1, s2); } //Compare } //ReverseStringComparer |
JScript
|
import System; import System.Text; import System.Collections; public class SamplesArrayList { public static function Main() : void { // Creates and initializes a new ArrayList. var myAL : ArrayList = new ArrayList(); myAL.Add("Eric"); myAL.Add("Mark"); myAL.Add("Lance"); myAL.Add("Rob"); myAL.Add("Kris"); myAL.Add("Brad"); myAL.Add("Kit"); myAL.Add("Bradley"); myAL.Add("Keith"); myAL.Add("Susan"); // Displays the properties and values of the ArrayList. Console.WriteLine( "Count: {0}", myAL.Count ); PrintValues ("Unsorted", myAL ); myAL.Sort(); PrintValues("Sorted", myAL ); myAL.Sort(new ReverseStringComparer() ); PrintValues ("Reverse" , myAL ); var names : String [] = (String[])(myAL.ToArray (System.String)); } public static function PrintValues(title : String, myList: IEnumerable ) : void { Console.Write ("{0,10}: ", title); var sb : StringBuilder = new StringBuilder(); for (var s : String in myList) { sb.AppendFormat( "{0}, ", s); } sb.Remove (sb.Length-2,2); Console.WriteLine(sb); } } public class ReverseStringComparer implements IComparer { public function Compare (x, y) : int { //negate the return value to get the reverse order return - (String(x), String(y)); } } SamplesArrayList.Main(); |