作为一名小白,android界面的还是应该要认识的
那么这篇文章的目的就是兼容android4.4和android5.0用两种方法来实现沉浸式状态栏(小白耐心看完,代码不多主要是图多)
第一种方法:设置状态栏透明化
我在qq空间随便get了一张手机截图,不知道是什么app的天气预报。这种方式利用的是将状态栏透明化(另一种方式状态栏设置颜色待会再说)
Google从Android kitkat(Android 4.4)开始(模仿IOS),给开发者提供了一套能透明的系统ui样式给状态栏和导航栏,所以要是实现这种浸入式导航栏,必须得android 4.4 以上的系统,而且android 4.4的系统和android 5.0的系统透明状态栏所实现的效果是不一样,什么区别?国际惯例上图吧(左4.4,右5.*)
虽然我们看到4.4 和5.* 的区别,这种效果的区别不是因为做法不同引起的,这里就介绍一下透明化式状态栏的效果
Activity.cs 直接用代码的方式,就这几行行代码,并没有去判断是否是5.*系统,仅仅只是设置状态栏为透明的。继承的主题是android自带的主题Theme.Light.NotitleBar。虽然你也可以写xml文件里面,但是在xamarin android 里面我发现设置状态栏透明属性无效,这的确是一个尴尬的地方,如果你知道怎么在xml文件里面设置状态栏的透明属性,欢迎评论。
[Activity(Label = "FirstActivity",MainLauncher =true,Theme = "@android:style/Theme.Light.NoTitleBar")]
public class FirstActivity : Activity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.First);
if (Build.VERSION.SdkInt >= Build.VERSION_CODES.Kitkat)
{
//透明状态栏
Window.AddFlags(WindowManagerFlags.TranslucentStatus);
//透明导航栏
Window.AddFlags(WindowManagerFlags.TranslucentNavigation);
}
}
}
布局文件是这样的:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:fitsSystemWindows="true"
android:layout_width="match_parent"
android:layout_height="140dp"
android:textSize="24dp"
android:background="@color/colorPrimary"
android:text="你好,沉浸式状态栏"
android:textColor="@color/white" />
</LinearLayout>
这个布局文件要注意的是:fitsSystemWIndows
属性,他是干嘛的呢?如果不设置为true的,可以看到效果是这样的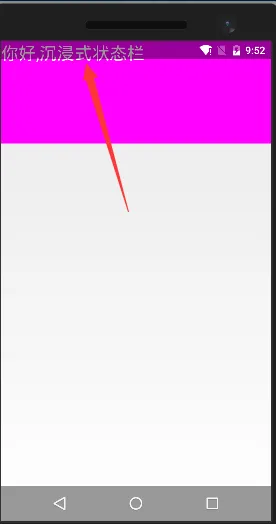
fitsSystemWindows属性的作用
简单的说就是:设置起bool值为true时就会自动调整view的padding属性,给system windows留出空间,实际效果: 当status bar为透明或半透明时(4.4以上),系统会设置view的paddingTop值为一个适合的值(status bar的高度)让view的内容不被上拉到状态栏,当在不占据status bar的情况下(4.4以下)会设置paddingTop值为0(因为没有占据status bar所以不用留出空间)。
第二种方法:设置状态栏的颜色

这里我使用Toolbar来展示这个效果,当然你也可以自定义标题栏来做出这个效果来。先上图
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:toolbar="http://schemas.android.com/apk/res-auto" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/colorPrimary" android:fitsSystemWindows="true"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="@color/colorPrimary" toolbar:logo="@drawable/menu" android:subtitle="子标题" toolbar:title="toolbar的标题" android:textColor="@color/white"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="toolbar的使用" android:textColor="@color/white"/> </android.support.v7.widget.Toolbar> <!--内容布局--> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:background="@android:color/white" android:orientation="vertical"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="显示信息" /> </LinearLayout> </LinearLayout>Activity.cs如下。还有android4.4是不能设置状态栏颜色的,要判断android4.4和android5.*。值得注意的是添加的Flag不是方法一的那种Translucent,而是DrawsSystemBarBackgrounds 它才能修改状态栏的颜色(android5.*)
[Activity(Label = "FirstActivity123",MainLauncher =true,Theme = "@style/TranslucentTheme")] public class FirstActivity : AppCompatActivity { protected override void OnCreate(Bundle savedInstanceState) { base.OnCreate(savedInstanceState); SetContentView(Resource.Layout.First); var toolBar = FindViewById<Android.Support.V7.Widget.Toolbar>(Resource.Id.toolbar); SetSupportActionBar(toolBar); SupportActionBar.SetDisplayShowTitleEnabled(false);//去掉标题 if (Build.VERSION.SdkInt >= BuildVersionCodes.Lollipop) { //清除透明状态栏,使内容不再覆盖状态栏 Window.ClearFlags(WindowManagerFlags.TranslucentStatus); Window.AddFlags(WindowManagerFlags.DrawsSystemBarBackgrounds); var Color = Resources.GetColor(Resource.Color.colorPrimary); Window.SetStatusBarColor(Color); //透明导航栏 部分手机导航栏不是虚拟的,比如小米的 Window.AddFlags(WindowManagerFlags.TranslucentNavigation); Window.SetNavigationBarColor(Color); } if (Build.VERSION.SdkInt >= BuildVersionCodes.Kitkat&&Build.VERSION.SdkInt <= BuildVersionCodes.Lollipop) { //状态栏透明 Window.AddFlags(WindowManagerFlags.TranslucentStatus); //透明导航栏 Window.AddFlags(WindowManagerFlags.TranslucentNavigation); } } public override bool OnCreateOptionsMenu(IMenu menu) { MenuInflater.Inflate(Resource.Drawable.base_toolbar_menu,menu); return true; } }
Theme translucent 继承的v7兼容包主题Theme.AppCompat.Light
<?xml version="1.0" encoding="utf-8" ?> <resources> <style name="TranslucentTheme" parent="AppTheme.Base"> </style> <style name="AppTheme.Base" parent="Theme.AppCompat.Light"> <item name="windowActionBar">false</item> <item name="windowNoTitle">true</item> </style> </resources>
菜单文件就没必要贴出来了,主要是感受一下标题栏的颜色和状态栏设置成一样的。
小结:对于每个Activity都要去这样设置,既不简洁又麻烦,所以可以写个父类或者写个工具Class。作者:张林
标题:Xamarin android沉浸式状态栏 原文地址:http://blog.csdn.net/kebi007/article/details/70215993
转载随意注明出处