本篇文章讲解了Spring的通过内部Bean设置Bean的属性。
类似内部类,内部Bean与普通的Bean关联不同的是:
1 普通的Bean,在其他的Bean实例引用时,都引用同一个实例。
2 内部Bean,每次引用时都是新创建的实例。
鉴于上述的场景,内部Bean是一个很常用的编程模式。
下面先通过前文所述的表演者的例子,描述一下主要的类:
package com.spring.test.setter; import com.spring.test.action1.PerformanceException; import com.spring.test.action1.Performer; public class Instrumentalist implements Performer{ private String song; private int age; private Instrument instrument; public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getSong() { return song; } public void setSong(String song) { this.song = song; } public Instrument getInstrument() { return instrument; } public void setInstrument(Instrument instrument) { this.instrument = instrument; } public Instrumentalist(){} public Instrumentalist(String song,int age,Instrument instrument){ this.song = song; this.age = age; this.instrument = instrument; } public void perform() throws PerformanceException { System.out.println("Instrumentalist age:"+age); System.out.print("Playing "+song+":"); instrument.play(); } }
其他代码,如下:
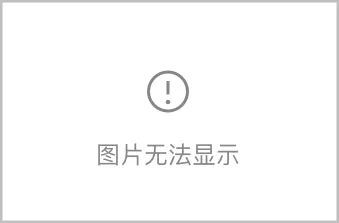
package com.spring.test.setter; public interface Instrument { public void play(); }
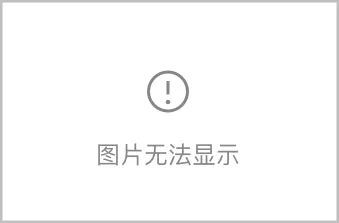
package com.spring.test.setter; public class Saxophone implements Instrument { public Saxophone(){} public void play() { System.out.println("TOOT TOOT TOOT"); } }
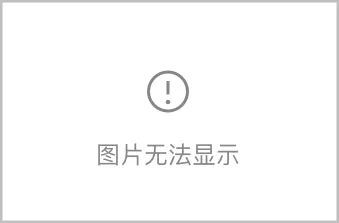
package com.spring.test.action1; public interface Performer { void perform() throws PerformanceException; }
如果使用 设值注入 需要设定属性和相应的setter getter方法。
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://www.springframework.org/schema/beans" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="kenny" class="com.spring.test.setter.Instrumentalist"> <property name="song" value="Jingle Bells" /> <property name="age" value="25" /> <property name="instrument"> <bean class="com.spring.test.setter.Saxophone"/> </property> </bean> </beans>
如果使用 构造注入 需要构造函数。
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://www.springframework.org/schema/beans" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="kenny-constructor" class="com.spring.test.setter.Instrumentalist"> <constructor-arg value="Happy New Year"/> <constructor-arg value="30"/> <constructor-arg> <bean class="com.spring.test.setter.Saxophone"/> </constructor-arg> </bean> </beans>
应用上下文使用方法:
public class test { public static void main(String[] args) throws PerformanceException { ApplicationContext ctx = new ClassPathXmlApplicationContext("bean.xml"); Instrumentalist performer = (Instrumentalist)ctx.getBean("kenny"); performer.perform(); Instrumentalist performer2 = (Instrumentalist)ctx.getBean("kenny-constructor"); performer2.perform(); } }
本文转自博客园xingoo的博客,原文链接:【Spring实战】—— 6 内部Bean,如需转载请自行联系原博主。