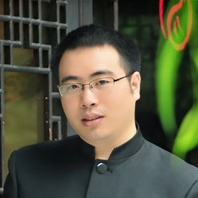
14年企业级软件及互联网产品研丰富发经验,曾任国内知名软件外包企业高级项目经理、手游公司技术总监。现任深圳前海童育汇电子商务股份有限公司产品研发负责人。
我们常常会遇到需要利用并行处理,尽量发挥多核或多CPU的潜能,提高程序运行效率的场景。在.NET环境下,常用的做法是使用Thread,多线程方式进行并行处理。但在.Net4.0中,微软提供一种新的概念——Task(任务),换句话说,并行处理由“多线程”进化为了“多任务”的方式。
C#中如果采用Process调用应用程序失败,请添加下列第一行,设置应用程序所在的路径。 Directory.SetCurrentDirectory([应用程序所在路径]); Process process = new Process(); process.
1、客户端:一个IT经理走进一家拉面馆说:“你们需要客户端吗?” 老板说:“面一般是伙计端,忙的时候才需要客户端。” 2、云计算:中国一留学生去美国打工的当过报童,不带计算器,习惯动作抬头望天时心算找零。
今日,网上偶然看到360安全卫士提醒更新——“360隐私保护器”。 真是不用不知道,一用吓一跳,大家天天使用的腾讯QQ,居然是潜伏在我们电脑里的一个重大特务分子,幸好360将其揪了出来。
vs2008支持.net3.5,而vs2005支持.net2.0,所以使用vs2005打开vs2008的项目,要确定你的项目是.net2.0的。下面介绍2种方法:方法1:用记事本打开.sln文件,你将看到Microsoft Visual Studio Solution File, Format Version 10.
Oracle Conversion Functions Version 11.1 Note: Functions for converting to date, numeric, string, and timestamp data types can be found through the related links.
最新做一些基金业绩比较的工作,必不可少的需要用到晨星、银河这两家证监会认可的大佬发布的业绩排行榜。但却存在一个很大的问题,两家公司的榜单格式都是自定义的,没有统一标准,也不是传统的二维表。 为了在此基础上开发的程序比较方便的获取数据,所以用VBA做了如下 《评级报告数据转换.xla》的EXCEL插件,较好的将榜单转换为了传统的二维表,这样不管是直接使用,还是导入数据库都比较方便,特分享与大家,有需要的同学可以下载试用。
项目中遇到一个需求,需要将多行合并为一行。表结构如下:NAME Null Type------------------------ --------- -----N_SEC_CODE NOT NULL CHAR(6)C_RESEARCHER_CODE NOT NULL VARCHAR2(20)此表保存了“股票”与“研究员”的对应关系数据,一般而言,对于同一只股票而言,可能有多个研究员对其进行跟踪研究。
最近用.Net新建了一个Webservice,在项目添加Log4Net后,测试状态下一切正常。 但一旦发布网站到IIS以后,发现日志不能正常记录了。屡次失败后,偶然想到,难道是文件夹权限的问题。 于是尝试为此服务文件夹的已验证用户设置修改和写入权限,日志记录OK! 留此备忘。
在过去差不多一个月的时间里,先后看到这样两则新闻: 1、微软新推的搜索引擎网站bing(必应)http://cn.bing.com/,因搜索结果可能包含YY视频内容,而且不需要进入视频所属网站,直接点击即可播放的便利功能。
昨晚和同事们在酒吧狂欢,回家倒头狂睡之后,睁开朦胧的睡眼,2009年已悄然来到。 好久没为空间写点什么了,枕边放着笔记本,躺在床上,决定为新年的第一天写上一篇。 2008,给我们带来了不少不好的回忆,天灾人祸。
如果在ORACLE里面用惯了Sequence的兄弟们,要在SqlServer里实现Sequence,就会发现没有现成的Sequence对象可以Create了。那应该怎么办呢? 当然这点小问题是难不倒我们程序员的,“max+1啊”,有人会说这样的方式。
一、更新: 常会遇到 OleDbException - "标准表达式中数据类型不匹配。"使用 OleDb 向 Access (.mdb) 插入 DateTime 数据时经常触发该异常。 解决办法: 对于日期型字段设置参数类型parameter.OleDbType = OleDbType.Date; 不要使用 DbDate, DbTime, DbTimeStamp。
5.12大地震——谢可欣 小学二年级血肉容容痛在心,救人一命乐开怀。房屋倒塌没了家,众志成城建家园。 帮小妹妹在我的博客里发一个,表示一下她对地震痛苦的看法和重建家园心愿。
GridView自带了数据排序功能。在设计视图下,只能对GridView的排序数据列和排序方向进行静态设置。在后台程序中,则需要用Attributes方式对GridView的这两个属性进行动态设置。 示例如下: (前台) DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.
资料来自:http://www.mozlite.com/Article/sqlserver2005.aspx 以下列出的是SqlServer2005在编程过程中的链接字符串,可用于C++,VC++,ASP,PHP,.NET等等语言中。
工作中我们常常需要加壳(escape)后传输或保存HTML文本,UI层使用时再进行脱壳(unescape)。 很庆幸.net为我们提供了非常好用的HttpUtility类,加壳时可采用HtmlEncode方法,脱壳时采用HtmlDecode。
很多时候我们需要在GridView的RowCommand之类的事件中需要获取当前行的一些关联性的数据值。但这些数据值又没有直接体现在GridView的列中。这个时候该怎么办呢? 有同学喜欢用隐藏列的方式,把需要使用但不显示的字段绑定到此列上,同时设置列宽为0或不显示,使用时可以用常规的取某行某列的方式来获取数据。
近日的一系列工作是做网站的营运维护,因此做了大量的支持工具。有Excel中写VBA的,也有直接C#做的工具。有时需要在C#中执行Excel VBA宏,甚至有时还需要在执行了VBA宏之后,获取返回值再进行相应的处理。
Webservice客户端使用一个WebMethod时,如果WebMethod内部出现异常,不管异常是系统级异常或者自定义的异常,均会被包装为SoapException类型的异常,返回给客户端。
update某个表时,如果需要和其他表关联,这样的SQL语句在ORACLE中实现起来比较呕心。各个where条件需要分别与一堆select语句嵌套使用才可,晕~~ 以下sql语句是在工作中写出的,做个备忘录: -- 更新应收账款净额update t_0303009 t1 set t1.
1、创建测试工程 2、创建测试CASE 3、创建测试列表,并拖动测试CASE到测试列表 4、测试运行测试——代码覆盖率——勾选要测试的项目 5、运行测试 6、查看代码覆盖率结果
项目中我们继承.net系统异常类做成了一个自定义的异常类:WPSYSException在实际程序中发现异常情况时,需要构造并抛出此类异常时,代码如下: //如果输入数组为空,报异常 if ((inDoubleArray == null) || (inDoubleArray.
做一个2个数据库间数据比较工具时遇到ORACLE中汉字排序的问题。 使用一下SQL select * from T_0303003 order by stock_holder 进行选取数据时(stock_holder为存放中文的字段),结果发现两库返回的记录顺序不一致。
昨天受令用VBA做个工具,查看我们项目数据库中某些分析结果表的数据,当然要结合主表之类的形式展现出来。 数据库为ORACLE 9i,因此选用了ADO方式,访问提供器采用了安装ORACLE客户端后自带的Provider Oracle for OLEDB。
现象: C#程序中需要以Provider=OraOLEDB.Oracle.1方式访问ORACLE数据库。但程序执行时报异常:未在本地计算机注册“OraOLEDB.Oracle.1”提供程序 解决: 服务器ORACLE为10g,虽然安装时选择了Oracle Data Provider for .net 和Oracle Provider for OLE DB。
用VBA做工具的过程中,遇见这样一个问题。使用FSO方式或者直接OPEN文件方式,生成的文本文件采用的字符集为当前操作系统默认字符集,不能选择字符集类型。这样的文件作为应用程序的配置文件或者作为js代码文件,常常会因为字符集不是UTF-8,不能直接使用,需要利用记事本进行一次人工的字符集转换。
下午检查一段SQL时,发现获取最新数据时,可以采用两种方式的SQL写法:1、取记录后按日期逆序后取ROWNUM=1,2、对表的日期取MAX,再和原表关联,取出最大日期对应的数据。为了验证效率,做了以下实验。
ASP.NET 2.0中CSS失效的问题总结 经常有人遇到ASP.NET 2.0(ASP.NET 1.x中可能是有效的)中CSS失效的问题,现将主要原因和解决方法罗列如下: 1,CSS文件路径不正确 这个问题属于Web开发中的基础问题,一般采用相对路径会出现这样的问题,或者样式文件写在了母版页里面,在内容页与母版页不在同一级目录下时会出现这样的问题。
很多时候不想写一些心情上的事了,单是一些技术上的觉悟、技巧之类的随笔。 老婆也在问我:怎么不像以前那样常写些东西了呢。我的解释是:在郁闷的时候、心情不好的时候才会有更多的感悟,如果开心了,生活优越了,让我感动的事也就越来越少。
在访问Formview模板内控件时遇到两个问题。 一是调用语句过早而访问不到Formview模板内控件,二是究竟用什么方法或属性来访问。 比如说其中有一个ID为UserNameTextBox的TextBox,我们要把当前已登陆用户的用户名传给它怎么做呢? 注意:formview在Page_Load的时候是不会呈现外观的,也就不会有TextBox了,所以在Page_Load里写程序无论怎么写也找不到formview1控件模板里的子控件UserNameTextBox。
今天有朋友请教在CS的WinForm中如何打印DataGridView中的内容。 网上搜索一番之后,还是在藏宝库CodeProject中找到一篇好文章《DataGridView Printing by Selecting Columns and Rows》(http://www.codeproject.com/KB/grid/PrintDataGrid_CS.aspx)效果图 【打印设置画面】 【打印预览画面】解决方案构成 这个打印解决方案由一个打印设置的窗体,及一个打印类组成。
在我参与的项目中,自打微软的 AJAX 提供了“ModalPopupExtender”这个玩意以后,网页上的提示信息或错误信息较多地采用了模式弹出的方式,用户体验更友好,效果也更酷。当然有时侯,需要在前台js中进行一些输入检查之类的操作,然后用对应的错误信息去设置弹出域中的错误文本,然后再让域弹出。
介绍大家三个在项目中用到的DataTable的小巧实用的方法(排序、检索、合并): 一、排序1 获取DataTable的默认视图2 对视图设置排序表达式3 用排序后的视图导出的新DataTable替换就DataTable(Asc升序可省略,多列排序用","隔开) DataView dv = dt.
缓存迷惑:项目中用到了股票代码的输入框,想使用经典ajax效果——自动完成。 可是与头头讨论时,头头觉得一输入字符就要服务器相应,而且预计的使用人数有点大的情况下,太耗服务器资源了。说服不了头头,只有采用变通的方式,把代码表放到js里,使用纯js实现自动完成功能。
看论坛里很多人都在问如果获取GridView当行的问题,当然解决这个问题有好几个方法:1 加RowCommand事件中,判断请求的发出按钮控件名,根据传递的参数来获取当前行中我们需要的参数。2 GridView设置datakeynames方式。
纯属小技巧,高手见笑了。一提到如何在前台JS调用后台C#方法,AJAX成为了必然的想法。只是实现的细节采用AJAX 1.0或者AjaxPro的区别。其实如果不用AJAX,我们也能够很方便地利用JS调用后台方法。
AjaxControlToolkit的日历控件(CalendarExtender)增加了全球化及本地化支持。 只要在中增加EnableScriptGlobalization="true"EnableScriptLocalization="true"两个属性即可
做前台功能设计的时候,遇到以下需求:文本框显示数据库中原来的文本,鼠标点击文本框即变为编辑状态,编辑结束时执行内容存储的后台事件。显示文本时的样式和与编辑状态时的样式风格也要求有不同。实验中遇到以下问题:初次页面展现时,如果文本框输入不正确,触发了验证控件。
目前参加项目是从去年开始的,去年用的ATLAS做了一些东西,今天上面要求升级为AJAX 1.0的版本。先从微软网站下载了ASP.NET AJAX 1.0(版本号:1.0.61025.0) 以及 3月2日发布的 AjaxControlToolkit (版本号:1.0.10301.0)接下来就是安装。
项目中在UpdatePanel范围内加入了一个GridView控件。如果此页面初始化有数据时,分页效果一切正常。但是当初始化时没有数据,页面中点击按钮加载数据后,再点分页数字时报异常:“Microsoft JScript 运行时错误: 缺少对象”经多方请教,几经周折后,终于搞定这个问题。
30分钟正则表达式指导 by Jim Hollenhorst 译 寒带鱼 你是否曾经想过正则表达式是什么,怎样能够快速得到对它的一个基本的认识?我的目的就是在30分钟内带你入门并且对正则表达式有一个基本的理解。
模式窗口中点击按钮让父窗口跳转到新的页面,不可用 opener.document.location 方式来设置,只能在父窗口把当前window对象传递到模式窗口,模式窗口使用 dialogArguments 来获得父窗口对象。
VS2005中提供了代码段的便捷功能,敲入一个关键字,当其在下拉列表中显示图标为一个文件的时候,敲两下TAB键,便能快速的获取这个代码片段,剩下所需做的就是移动到对应的位置,填入参数性质的东西替换预留的位置。
很多时候我们需要链接转向(Url Rewriting),例如二级域名转向、文章访问链接等场合。让我们看两个例子:1 你现在看到的当前作者的博客园的域名:http://heekui.cnblogs.com 实际上是 http://www.cnblogs.com/heekui 的一种链接重写(Url Rewriting)。
GridView中的超级链接,可以设置一个模版列,放入超级链接的控件,设置绑定参数即可。数据绑定方式有两种,如下示例:Eval方式 Bind方式 推荐使用第一种方式,可以在一个里放入多个绑定,而第二种只能如此绑定一个值做超级链接的控件,我们也有多种选择:1 asp:LinkButton 示例 2 asp:HyperLink示例 3 a标签示例 a标签:链接js事件: 绑定多个数据项的时候,也能采用下列方式(推荐使用): LinkButton 不好设置,推荐使用HyperLink或者a的方式,简单实用。
WEB开发有些时候我们会遇到弹出文件对话框,选择一个本地文件的需求。在ASP.NET 2.0下,我们可以直接采用FileUpload控件来做到这一点。这是一个组合控件,由一个文本框和一个按钮组成,实质就是html下的input(file)控件。
打开网页后.在文本框中无法输入文字.并且点鼠标右键也不好用. 原因:修复恶意插件后.破坏了IE内文件. 解决办法:从正常的机器上拷贝c:\windows\system32\mshtmled.dll到本机的system32目录下即可。
回到家了,没有开发环境,这几天只能看网页学习一下。这是csdn上转载的一篇,有些东东还是值得一看的。1. oncontextmenu="window.event.returnValue=false" 将彻底屏蔽鼠标右键no 可用于Table 2.
一直期待ATLAS能够提供AutoComplete的扩展特性,终于不负众望,在最新版的ASP.NET AJAX Control Toolkit 已经包含了这个特性:AutoCompleteExtender。