在 Android开发之自定义View(一)中,讲解了最复杂的一种自定义View,本次将剩下的两种讲完~~~ go,go,go
继承原有的控件,在原有控件基础上进行修改,如TextView,这种方式常见且简单。以实现一个显示粗体文本的TextView例子来讲解。
1、自定义属性
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="BoldTextView">
<attr name="textIsBlod" format="boolean" />
</declare-styleable>
</resources>
2、创建一个类继承自TextView,很简单,内容都是前面讲过的
public class BoldTextView extends TextView
{
private TextPaint paint;
public BoldTextView(Context context, AttributeSet attrs)
{
super(context, attrs);
TypedArray params = context.obtainStyledAttributes(attrs,
R.styleable.BoldTextView);
// 得到自定义控件的属性值。
boolean textIsBlod = params.getBoolean(
R.styleable.BoldTextView_textIsBlod, false);
setTextblod(textIsBlod);
params.recycle();
}
// 设置粗体 其实就是将画笔变粗即可
public void setTextblod(boolean textblod)
{
if (textblod)
{
paint = super.getPaint();
paint.setFakeBoldText(true);
}
}
}
3、布局
<com.example.diyview.BoldTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="粗体字,你能看出来吗?"
android:textSize="30sp"
app:textIsBlod="true" />
4、运行测试
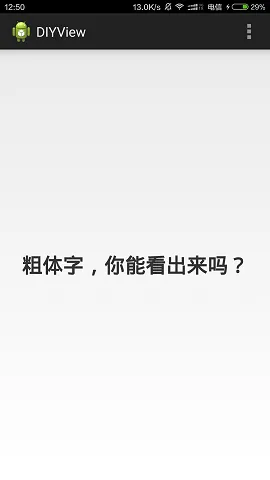
自定义View4.png
重新拼装组合,这种方式也比较常见。以实现一个图片文字按钮例子来讲解。
1、自定义属性
<declare-styleable name="ImgBtn">
<attr name="imgbtn_title" format="string" />
<attr name="imgbtn_icon" format="reference" />
</declare-styleable>
2、组合控件布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
android:padding="@dimen/activity_vertical_margin" >
<ImageView
android:id="@+id/icon"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
3、创建一个类继承自LinearLayout
public class ImgBtn extends LinearLayout
{
private TextView title;
private ImageView icon;
public ImgBtn(Context context)
{
super(context);
}
public ImgBtn(Context context, AttributeSet attrs)
{
super(context, attrs);
// 加载布局
LayoutInflater.from(context).inflate(R.layout.diy_view, this, true);
// 找到控件
title = (TextView) findViewById(R.id.title);
icon = (ImageView) findViewById(R.id.icon);
// 设置属性
TypedArray params = context.obtainStyledAttributes(attrs,
R.styleable.ImgBtn);
int resId = params.getResourceId(R.styleable.ImgBtn_imgbtn_icon,
R.drawable.ic_launcher);
// 设置图表
setIcon(resId);
String title = params.getString(R.styleable.ImgBtn_imgbtn_title);
// 设置文本
setTitle(title);
params.recycle();
}
public void setIcon(int resId)
{
icon.setImageResource(resId);
}
public void setTitle(String text)
{
title.setText(text);
}
}
3、Activity布局
<com.example.diyview.ImgBtn
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:imgbtn_icon="@drawable/icon_set"
app:imgbtn_title="设置" />
4、运行测试
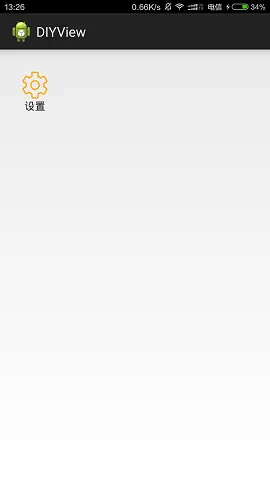
自定义View5.png