1). Navigation 官方文档
2). 安装
yarn add react-navigation
# or with npm
# npm install --save react-navigation
3). 定义页面
- Home.js
import React, {PureComponent} from 'react';
import {
Button,
StyleSheet, Text,
View
} from 'react-native';
/**
* @FileName: Find
* @Author: mazaiting
* @Date: 2018/6/12
* @Description:
*/
class Home extends PureComponent {
static navigationOptions = {
title: 'Home'
};
render() {
return (
<View style={styles.container}>
<Text>首页</Text>
<Button
title='跳转'
onPress={() => {
this.props.navigation.navigate('Detail')
}}
/>
</View>
)
}
}
/**
* 样式属性
*/
const styles = StyleSheet.create({
container: {
backgroundColor: '#DDD'
}
});
/**
* 导出当前Module
*/
module.exports = Home;
- Find.js
import React, {PureComponent} from 'react';
import {
StyleSheet, Text,
View
} from 'react-native';
/**
* @FileName: Find
* @Author: mazaiting
* @Date: 2018/6/12
* @Description:
*/
class Find extends PureComponent {
static navigationOptions = {
title: 'Find'
};
render() {
return (
<View style={styles.container}>
<Text>发现</Text>
</View>
)
}
}
/**
* 样式属性
*/
const styles = StyleSheet.create({
container: {
backgroundColor: '#DDD'
}
});
/**
* 导出当前Module
*/
module.exports = Find;
- Detail.js
import React, {PureComponent} from 'react';
import {
StyleSheet, Text,
View
} from 'react-native';
/**
* @FileName: Mine
* @Author: mazaiting
* @Date: 2018/6/12
* @Description:
*/
class Detail extends PureComponent {
static navigationOptions = {
title: 'Detail'
};
render() {
return (
<View style={styles.container}>
<Text>详细页面</Text>
</View>
)
}
}
/**
* 样式属性
*/
const styles = StyleSheet.create({
container: {
backgroundColor: '#DDD'
}
});
/**
* 导出当前Module
*/
module.exports = Detail;
4). 创建导航栈
/**
* 主页 栈
*/
const HomeStack = createStackNavigator({
Home: HomeScreen,
Detail: DetailScreen,
});
/**
* 发现栈
*/
const FindStack = createStackNavigator({
Find: FindScreen,
Detail: DetailScreen
});
5). 创建底部导航器
import React from 'react';
import {createStackNavigator, createBottomTabNavigator} from 'react-navigation';
import TabBarItem from './TabBarItem';
import HomeScreen from './Home';
import FindScreen from './Find';
import DetailScreen from './Detail';
/**
* 主页 栈
*/
const HomeStack = createStackNavigator({
Home: HomeScreen,
Detail: DetailScreen,
});
/**
* 发现栈
*/
const FindStack = createStackNavigator({
Find: FindScreen,
Detail: DetailScreen
});
/**
* 底部导航器
* 官网 https://reactnavigation.org/docs/en/getting-started.html
*/
const BottomTabNavigatorArticle = createBottomTabNavigator(
{
Home: HomeStack,
Find: FindStack
},
{
navigationOptions: ({navigation}) => ({
tabBarIcon: ({focused, tintColor}) => {
const {routeName} = navigation.state;
switch (routeName) {
case 'Home':
return (
<TabBarItem
tintColor={tintColor}
focused={focused}
normalImage={require('./../../../img/tab_home.png')}
selectedImage={require('./../../../img/tab_home_press.png')}
/>
);
case 'Find':
return (
<TabBarItem
tintColor={tintColor}
focused={focused}
normalImage={require('./../../../img/tab_find.png')}
selectedImage={require('./../../../img/tab_find_press.png')}
/>
);
}
},
tabBarOptions: {
activeTintColor: 'tomato',
inactiveTintColor: 'gray'
}
})
}
);
/**
* 导出当前Module
*/
module.exports = BottomTabNavigatorArticle;
其中TabBarItem.js
import React, {PureComponent} from 'react';
import {
Image
} from 'react-native';
// npm install --save prop-types
import PropTypes from 'prop-types';
const ImageSourcePropType = require('ImageSourcePropType');
/**
* @FileName: TabBarItem
* @Author: mazaiting
* @Date: 2018/6/12
* @Description:
*/
class TabBarItem extends PureComponent {
/**
* 属性参数
属性名称: React.PropTypes.array,
属性名称: React.PropTypes.bool,
属性名称: React.PropTypes.func,
属性名称: React.PropTypes.number,
属性名称: React.PropTypes.object,
属性名称: React.PropTypes.string,
*/
static propTypes = {
focused: PropTypes.bool.isRequired,
selectedImage: ImageSourcePropType,
normalImage: ImageSourcePropType
};
render() {
const image = this.props.focused ? this.props.selectedImage : this.props.normalImage;
return (
<Image source={image} style={{tintColor: this.props.tintColor, width: 22, height: 22}}/>
)
}
}
/**
* 导出当前Module
*/
module.exports = TabBarItem;
6). 注册启动
import BottomTabNavigatorArticle from './js/article/tab/BottomTabNavigatorArticle'
// 设置关闭警告提示
global.console.disableYellowBox = true;
AppRegistry.registerComponent('abcd', () => BottomTabNavigatorArticle);
7). 预览效果
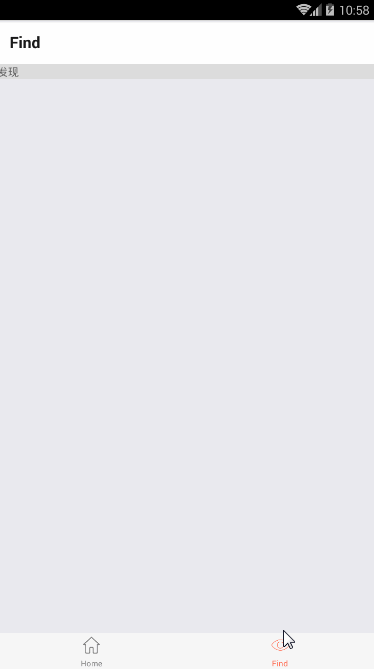
图1.gif
8). 设置导航器标题居中
- Home.js
static navigationOptions = {
title: 'Home',
headerTitleStyle: {
flex: 1,
textAlign: 'center',
}
};
- Detail.js
static navigationOptions = {
title: 'Detail',
// 如果导航器左边没有控件,则只设置这一句
headerTitleStyle: {
flex: 1,
textAlign: 'center',
},
// 如果导航器左侧有控件,则添加这一句
headerRight: (
// 该View可替换为其他组件
<View style={{height: 44,width: 55,justifyContent: 'center',paddingRight:15} }/>
)
};
-
效果
图2.gif