#include <QApplication>
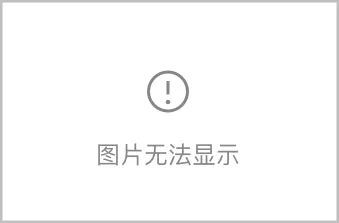
#include <QHBoxLayout>
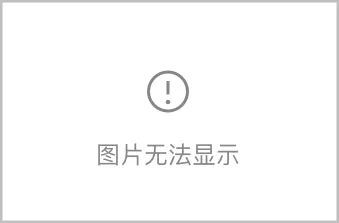
#include <QSlider>
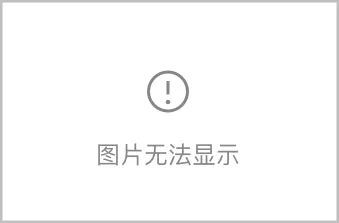
#include <QSpinBox>
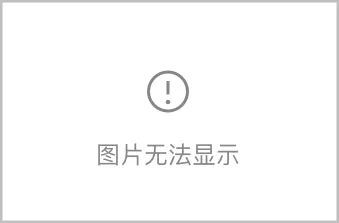
#include <QLabel>
int main(
int argc,
char *argv[])
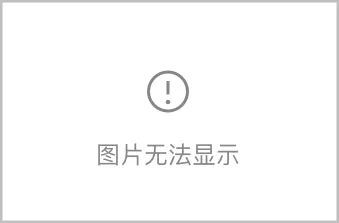
{
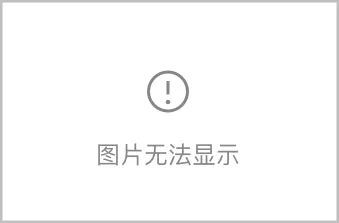
QApplication app(argc, argv);
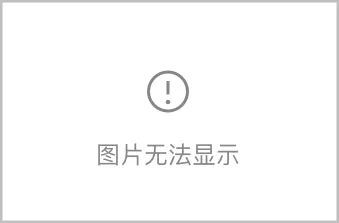
QWidget *window =
new QWidget;
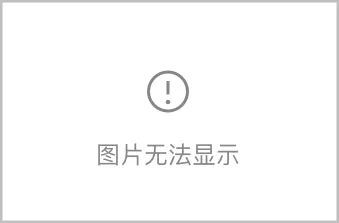
window->setWindowTitle(
"Enter Your Age");
//标题显示:输入你的年龄
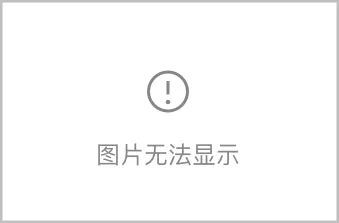
QSpinBox *spinBox =
new QSpinBox;
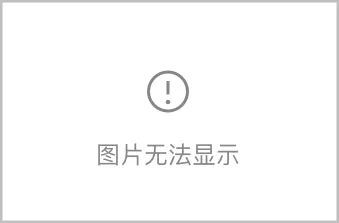
QSlider *slider =
new QSlider(Qt::Horizontal);
//滑块组件
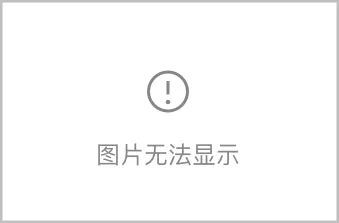
spinBox->setRange(0, 130);
//设置各自的取值范围
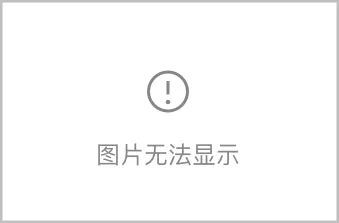
slider->setRange(0, 130);
//滑块和Spin组件的值的变化都会对应的改变
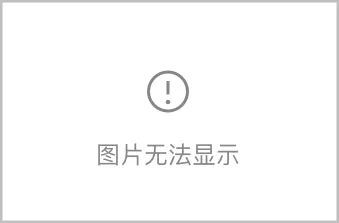
QObject::connect(spinBox, SIGNAL(valueChanged(
int)),
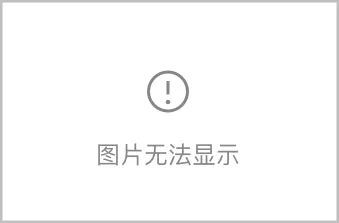
slider, SLOT(setValue(
int)));
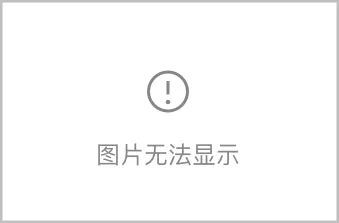
QObject::connect(slider, SIGNAL(valueChanged(
int)),
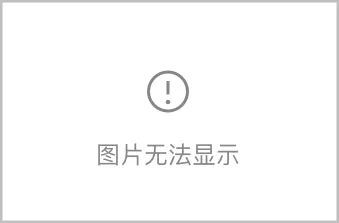
spinBox, SLOT(setValue(
int)));
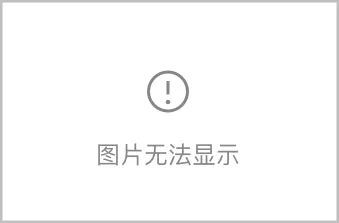
spinBox->setValue(35);
//注意这里的设置也会影响slider
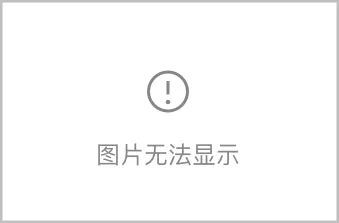
QHBoxLayout *layout =
new QHBoxLayout;
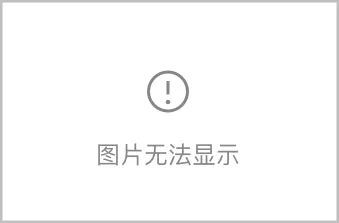
layout->addWidget(spinBox);
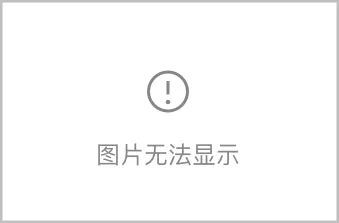
layout->addWidget(slider);
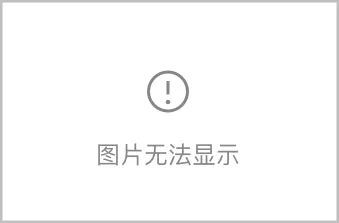
QLabel* label=
new QLabel(
"your age:");
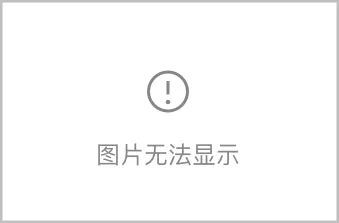
QVBoxLayout* mainLayout=
new QVBoxLayout;
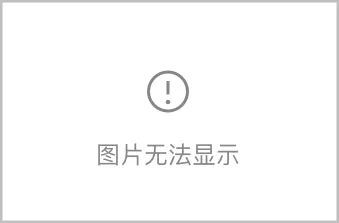
mainLayout->addWidget(label);
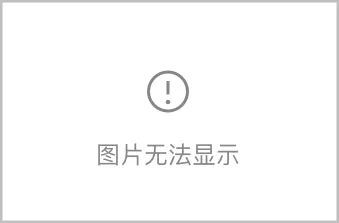
mainLayout->addLayout(layout);
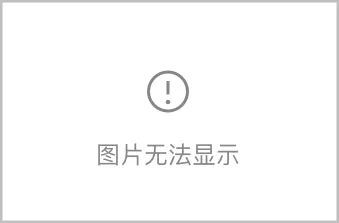
window->setLayout(mainLayout);
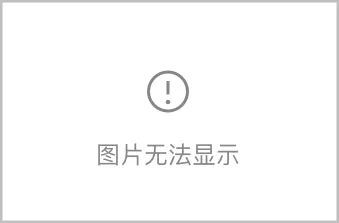
window->resize(300,50);
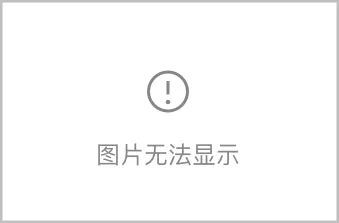
window->show();
return app.exec();
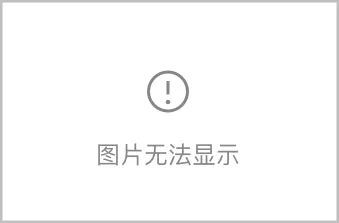
}