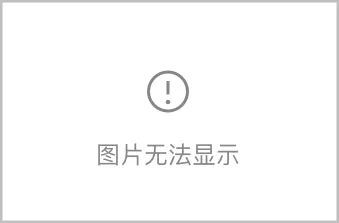
1 #include <stdio.h> 2 #include <stdlib.h> 3 #include <unistd.h> 4 #include <time.h> 5 #include <errno.h> 6 #include <string.h> 7 8 int main() 9 { 10 time_t now; 11 struct tm *ptime; 12 char *ptstr; 13 char timebuf[100]; 14 memset(timebuf,0,100); 15 //获取时间,秒数 16 now = time(&now); 17 printf("Global time is:\n"); 18 //转化为国际时间 19 ptime = gmtime(&now); 20 ptime->tm_year += 1900; 21 ptime->tm_mon += 1; 22 printf("%d-%d-%d %d:%d:%d\n",ptime->tm_year,ptime->tm_mon,ptime->tm_mday, 23 ptime->tm_hour,ptime->tm_min,ptime->tm_sec); 24 printf("Local time is:\n"); 25 //转化为本地时间 26 ptime = localtime(&now); 27 ptime->tm_year += 1900; 28 ptime->tm_mon += 1; 29 printf("%d-%d-%d %d:%d:%d\n",ptime->tm_year,ptime->tm_mon,ptime->tm_mday, 30 ptime->tm_hour,ptime->tm_min,ptime->tm_sec); 31 //将tm结构转换为字符串 32 ptstr = asctime(ptime); 33 printf("tm time stirng is: %s",ptstr); 34 //将time_t类型转换为字符串 35 ptstr = ctime(&now); 36 printf("time_t time string is: %s",ptstr); 37 //date 格式化输出时间 38 strftime(timebuf,100,"%YYear %mMonth %dDay %A %X",ptime); 39 printf("time buf is:%s\n",timebuf); 40 return 0; 41 }
程序执行结果如下所示:
总结:加深对Unix的系统数据文件及时间日期的认识,能够调用系统函数获取系统相关数据。