前几天做了一个
JScript版的CollecionBase类
,用来解决需要使用集合作为主要数据结构的类的基类。不过当时挺忙的没有给出继承的示例,搞得有的网友对JavaScript继承比较迷惑,于是今天使用四种方式来分别实现了4个ArrayList派生类。
关于使用JavaScript进行面向对象编程(OOP),网上已有很多的文章说过了。这里我推荐两篇文章大家看看,如果没有理解怎么使用JavaScript的Function对象的prototype属性来实现类定义及其原理,那么就仔细看看' 面向对象的JavaScript编程 '、' 面向对象的Jscript '和' Classical Inheritance in JavaScript '哦(特别是第一篇及其相关讨论的文章),否则后面一头雾水不能怪我啦
。
那个CollectionBase就不列了,第一个链接就是它了,下面是用四种方法实现的JavaScript'继承'(以后就不打引号了,反正知道JavaScript的继承不是经典OO里的继承就行了):
构造继承法:
<script language="javascript">
function
ArrayList01()
{
this.base =
CollectionBase;
this
.base();
this.m_Array = this
.m_InnerArray;
this.foo = function
()
{
document.write(this + ': ' + this.m_Count + ': ' + this.m_Array + '<br />
');
}
;
this.Add = function
(item)
{
this.InsertAt(item, this
.m_Count);
}
;
this.InsertAt = function
(item, index)
{
this.m_InnerArray.splice(index, 0
, item);
this.m_Count++
;
}
;
this.toString = function
()
{
return
'[class ArrayList01]';
}
;
}
</script>
原形继承法:
<script language="JavaScript">
function
ArrayList02()
{
this.InsertAt = function
(item, index)
{
this.m_InnerArray.splice(index, 0
, item);
this.m_Count++
;
}
;
this.m_Array = this
.m_InnerArray;
this.toString = function
()
{
return
'[class ArrayList02]';
}
;
}
ArrayList02.prototype
= new
CollectionBase();
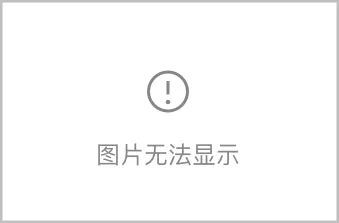
ArrayList02.prototype.foo = function
()
{
document.write(this + ': ' + this.m_Count + ': ' + this.m_Array + '<br />
');
}
;
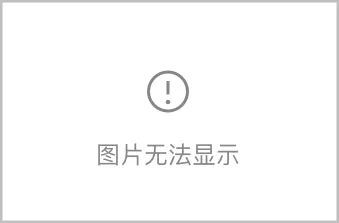
ArrayList02.prototype.InsertAt = function
(item, index)
{
this.m_InnerArray.splice(index, 0
, item);
this.m_Count++
;
}
;
</script>
实例继承法:
<script language="javascript">
function
ArrayList03()
{
var base = new
CollectionBase();
base.m_Array =
base.m_InnerArray;
base.foo = function
()
{
document.write(this + ': '+ this.m_Count + ': ' + this.m_Array + '<br />
');
}
;
base.InsertAt = function
(item, index)
{
this.m_InnerArray.splice(index, 0
, item);
this.m_Count++
;
}
;
base.toString = function
()
{
return
'[class ArrayList03]';
}
;
return
base;
}
</script>
附加继承法:
<script language="JavaScript">
function
ArrayList04()
{
this.base = new
CollectionBase();
for ( var key in this
.base )
{
if ( !this
[key] )
{
this[key] = this
.base[key];
}
}
this.m_Array = this
.m_InnerArray;
this.InsertAt = function
(item, index)
{
this.m_InnerArray.splice(index, 0
, item);
this.m_Count++
;
}
;
}
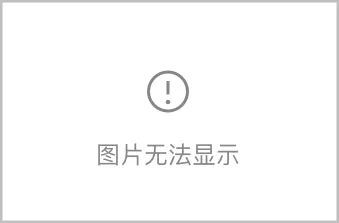
ArrayList04.prototype.foo
= function
()
{
document.write(this + ': ' + this.m_Count + ': ' + this.m_Array + '<br />
');
}
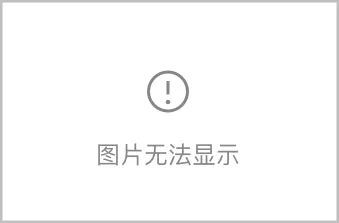
ArrayList04.prototype.toString
= function
()
{
return
'[class ArrayList04]';
}
</script>
关于使用JavaScript进行面向对象编程(OOP),网上已有很多的文章说过了。这里我推荐两篇文章大家看看,如果没有理解怎么使用JavaScript的Function对象的prototype属性来实现类定义及其原理,那么就仔细看看' 面向对象的JavaScript编程 '、' 面向对象的Jscript '和' Classical Inheritance in JavaScript '哦(特别是第一篇及其相关讨论的文章),否则后面一头雾水不能怪我啦
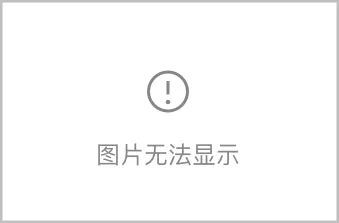
那个CollectionBase就不列了,第一个链接就是它了,下面是用四种方法实现的JavaScript'继承'(以后就不打引号了,反正知道JavaScript的继承不是经典OO里的继承就行了):
构造继承法:
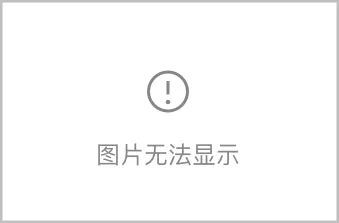
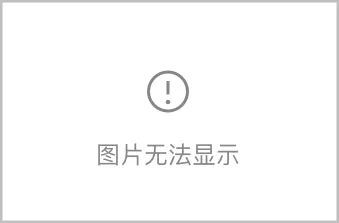
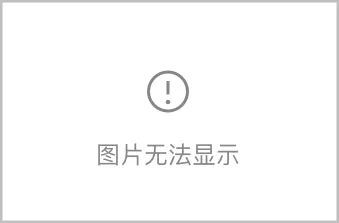
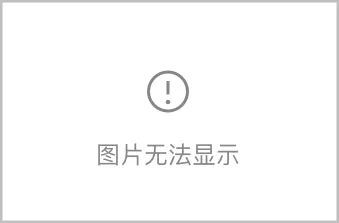
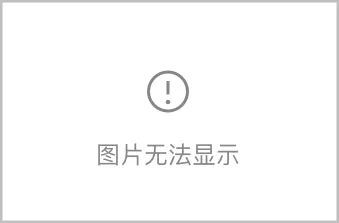
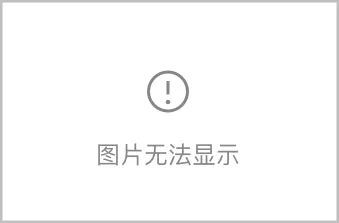
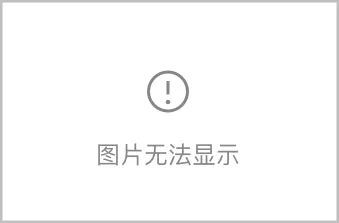
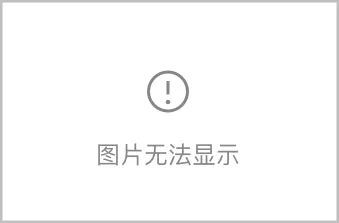
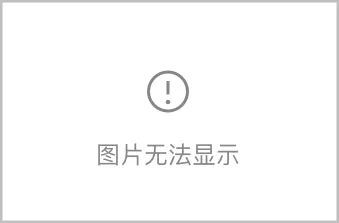
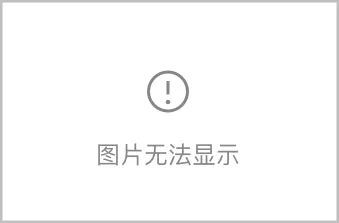
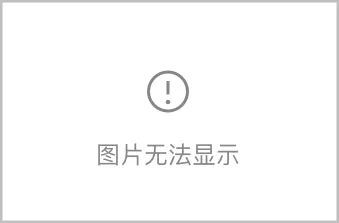
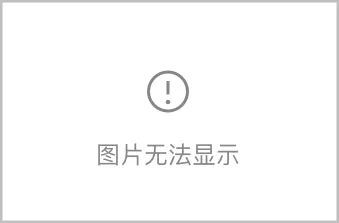
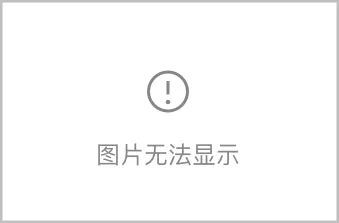
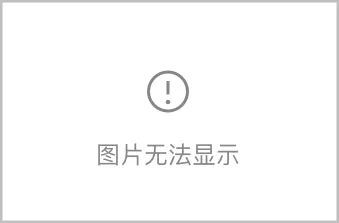
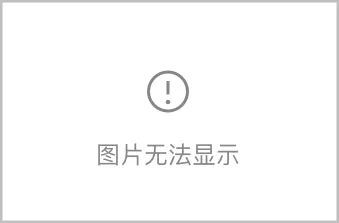
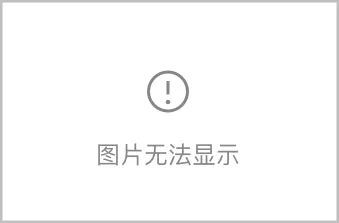
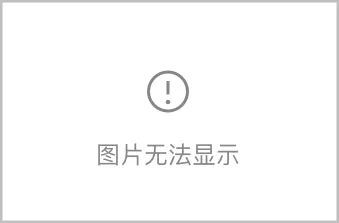
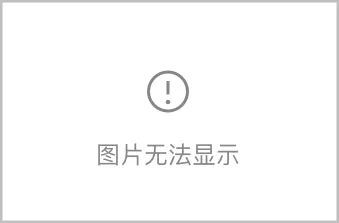
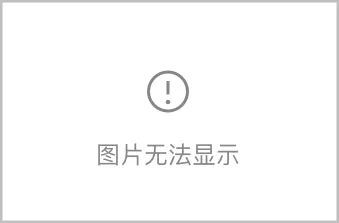
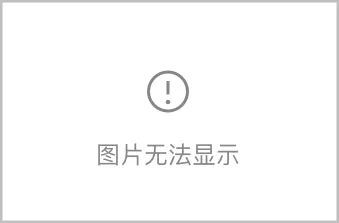
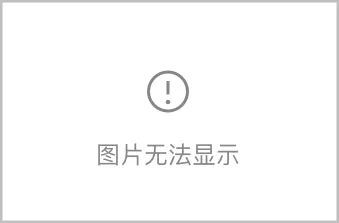
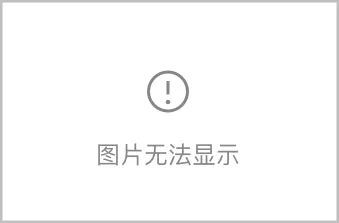
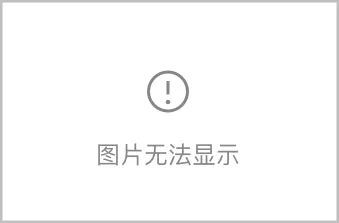
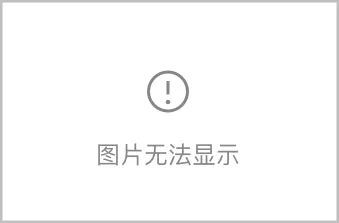
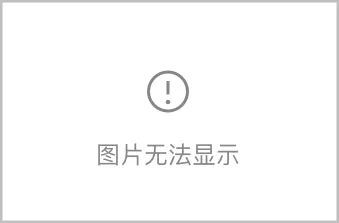
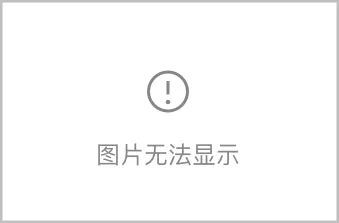
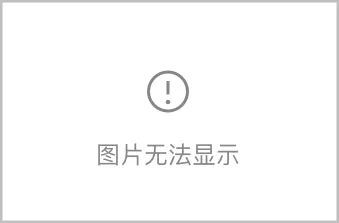
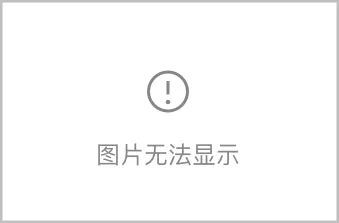
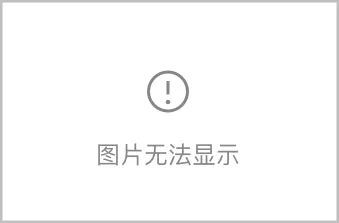
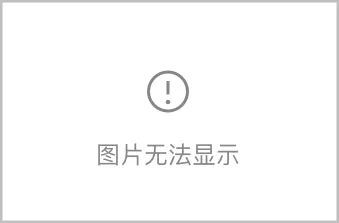
原形继承法:
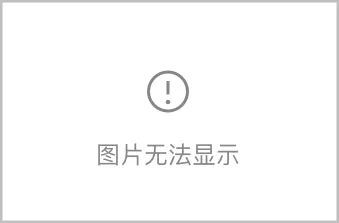
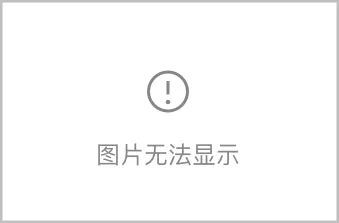
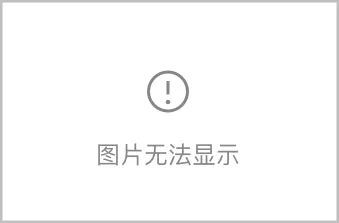
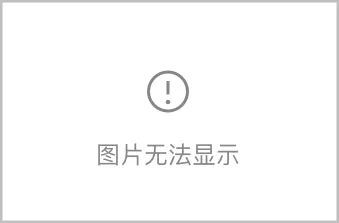
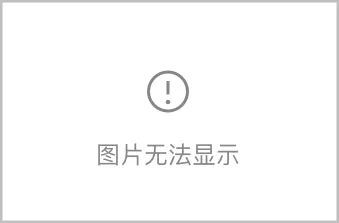
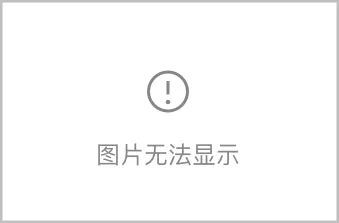
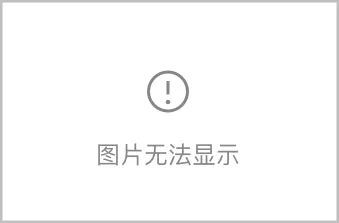
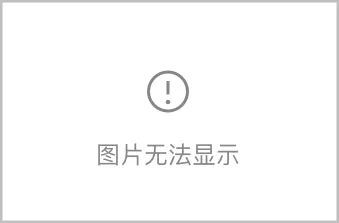
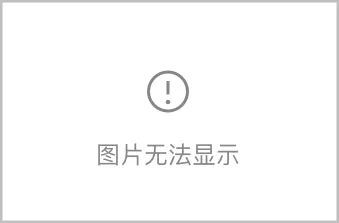
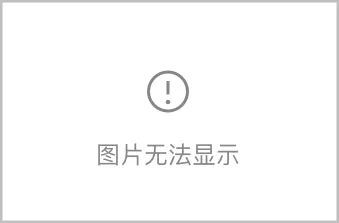
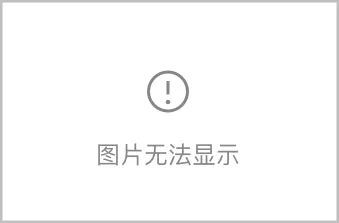
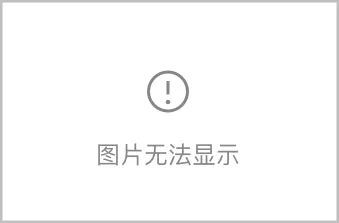
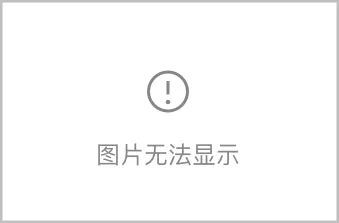
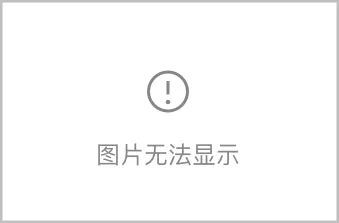
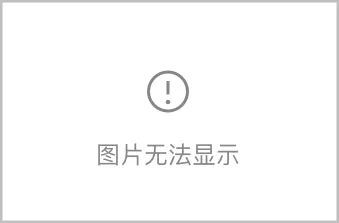
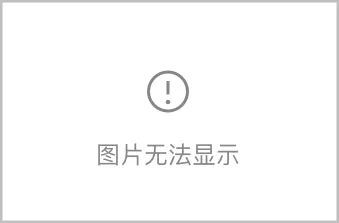
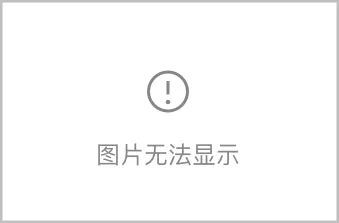
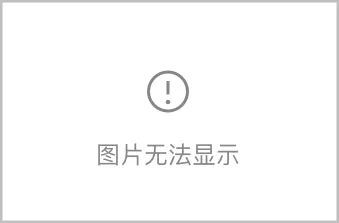
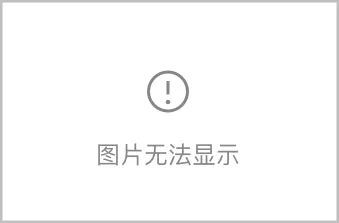
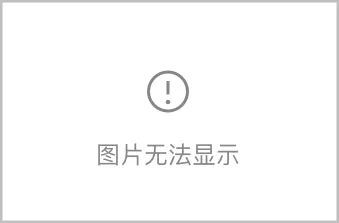
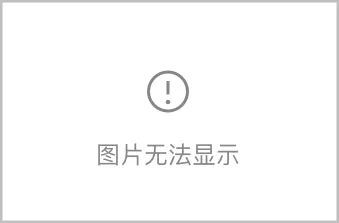
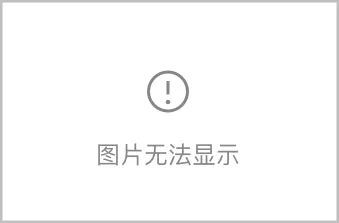
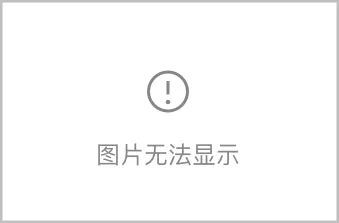
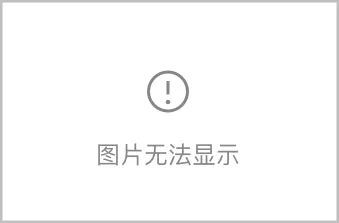
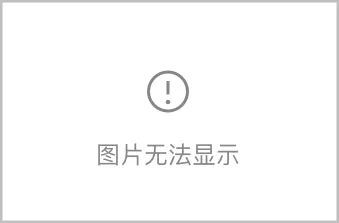
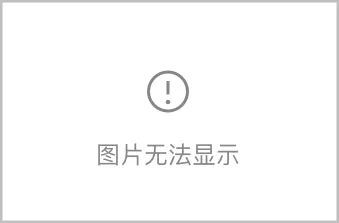
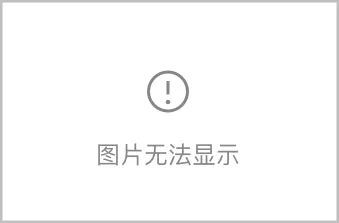
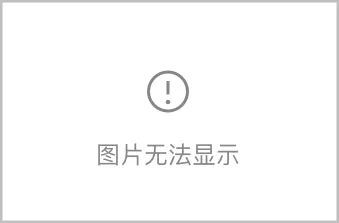
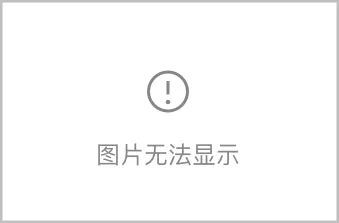
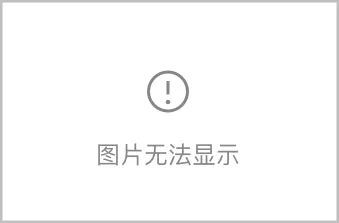
实例继承法:
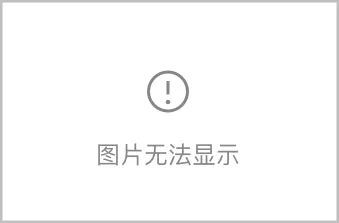
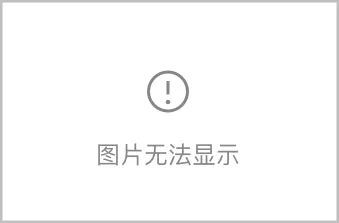
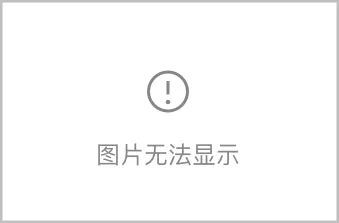
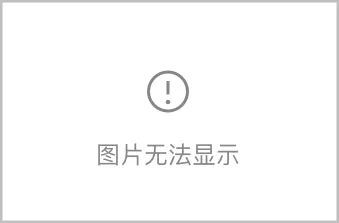
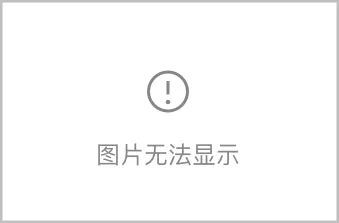
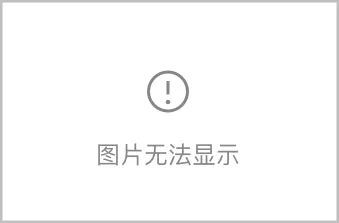
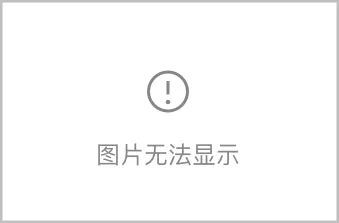
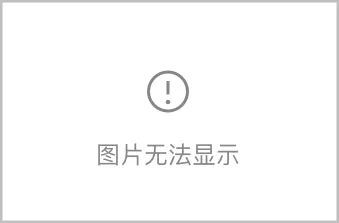
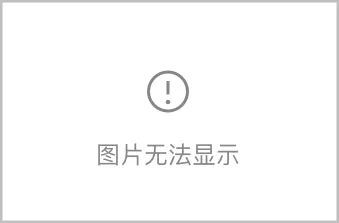
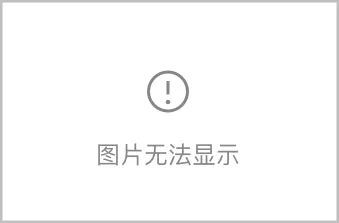
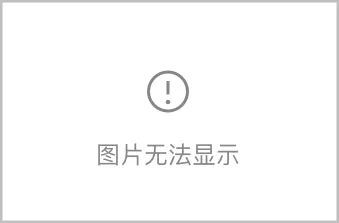
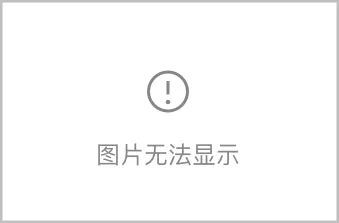
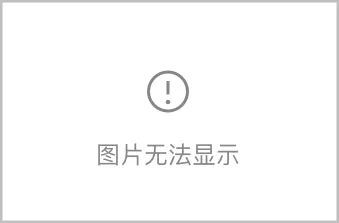
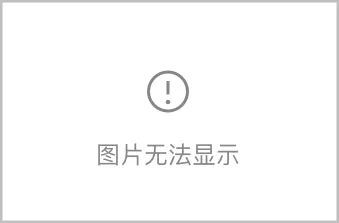
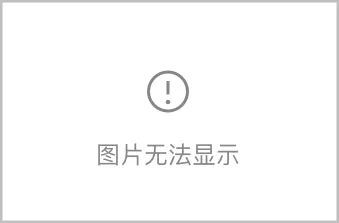
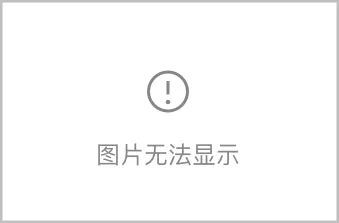
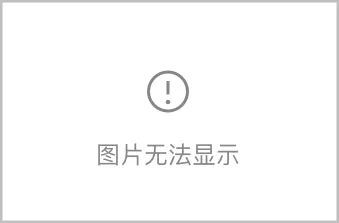
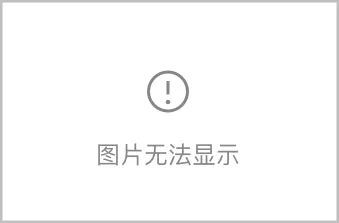
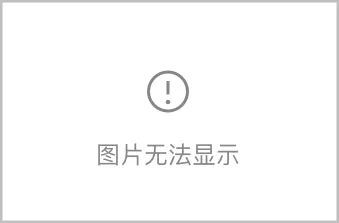
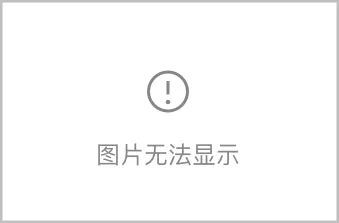
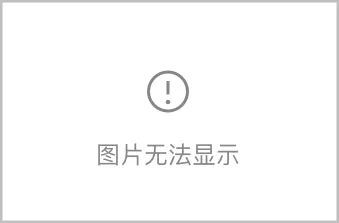
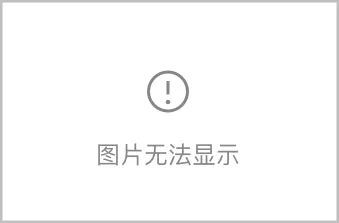
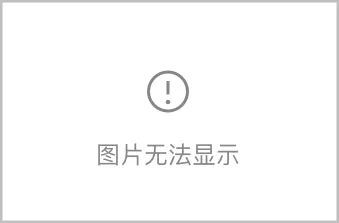
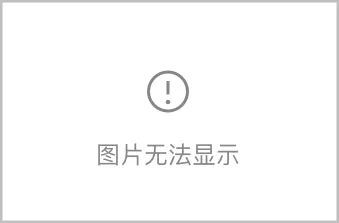
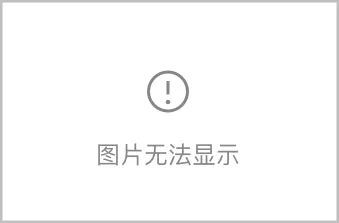
附加继承法:
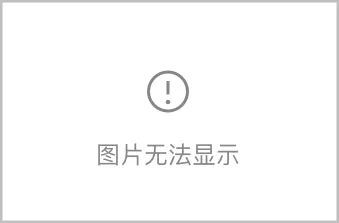
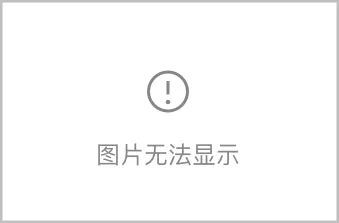
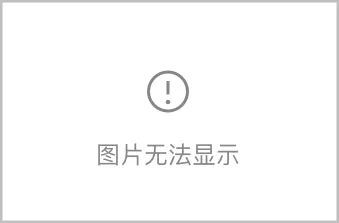
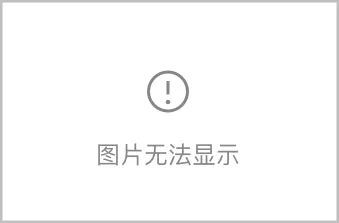
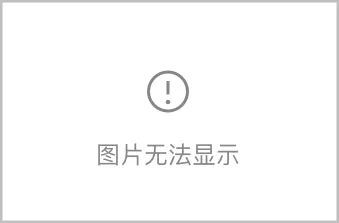
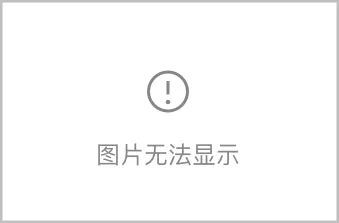
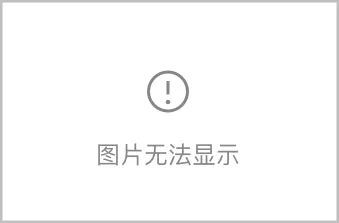
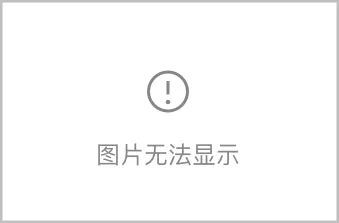
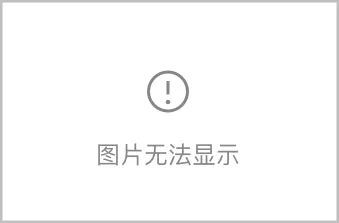
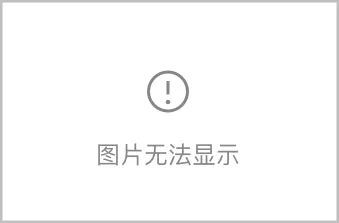
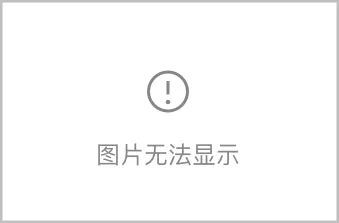
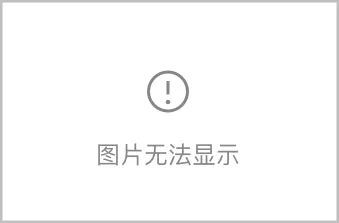
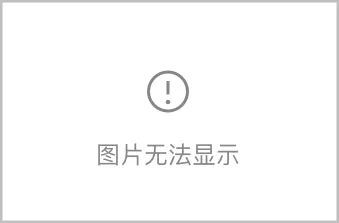
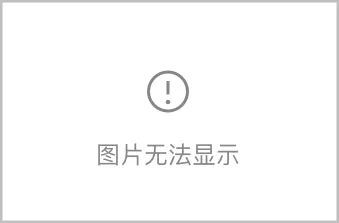
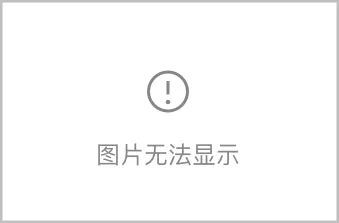
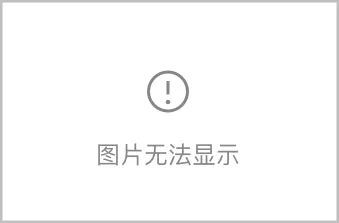
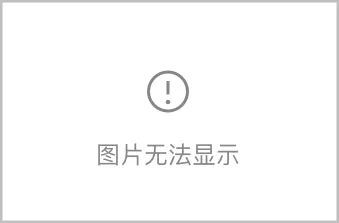
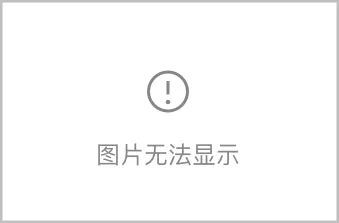
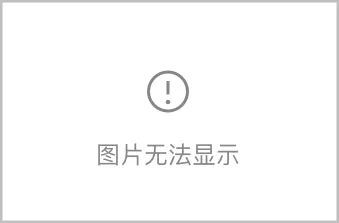
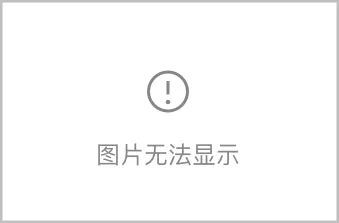
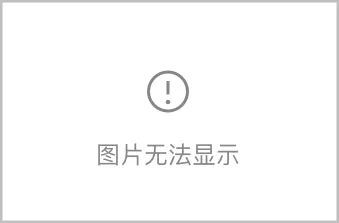
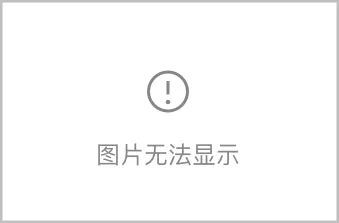
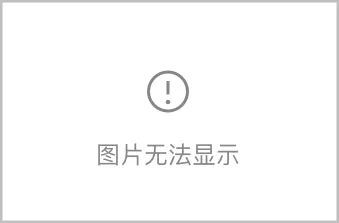
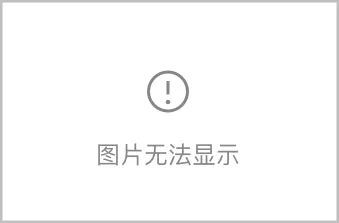
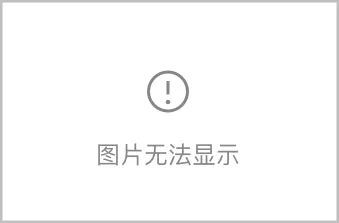
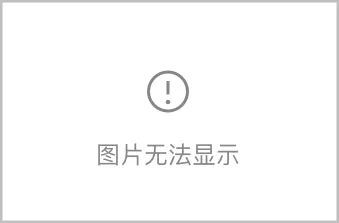
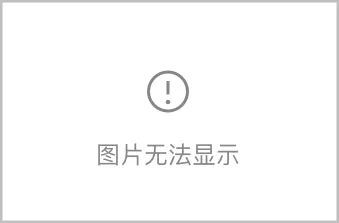
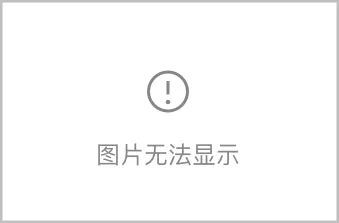
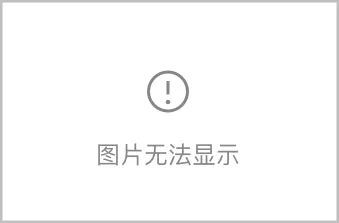
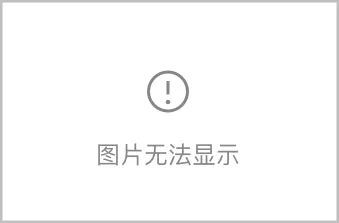
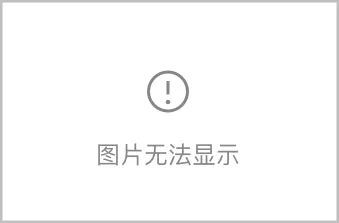
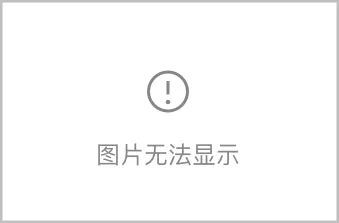
派生类中的foo是一个新增加的函数,用来输出类的类型和m_InnerArray里的数据。toString()相当于override了CollectionBase中的toString(),不过其实就是赋值覆盖,和'从JavaScript函数重名看其初始化方式'一文中说到的原理是一样的。这个四种方法的原理和区别我稍候再作分析,要了...
本文转自博客园鸟食轩的博客,原文链接:http://www.cnblogs.com/birdshome/,如需转载请自行联系原博主。