- CCLayerColor::initWithColor(ccc4(255,255,255,255));
- CCImage *pImage = new CCImage();
- pImage->autorelease();
- pImage->initWithImageFile("arraw.png",CCImage::EImageFormat::kFmtPng);
- //遍历图片的所有像素.
- unsigned char *pData = pImage->getData();
- int nPixelIndex = 0;
- for (int nCol = 0; nCol < pImage->getHeight(); nCol ++)
- {
- for (int nRow = 0; nRow < pImage->getWidth(); nRow ++)
- {
- //取图片的RGB值.
- int nBeginPos = nPixelIndex;
- unsigned int nRValue = pData[nPixelIndex];
- nPixelIndex++;
- unsigned int nGValue = pData[nPixelIndex];
- nPixelIndex ++;
- unsigned int nBValue = pData[nPixelIndex];
- nPixelIndex ++;
- unsigned int nAValue = pData[nPixelIndex];
- nPixelIndex ++;
-
- int nAlphaRatio = 0;
- //本代码的核心:取RGB中的最大值赋给nAlphaRatio。如果nAlphaRatio为0,则像素中的alpha通道就为0,否则像素中的
- //alpha通道值就是nAlphaRatio。这样做是为了在图片中颜色渐变过渡比较大的区域实现平滑的过渡。让最终形成的
- //图片看起来不粗糙.
- nAlphaRatio = nRValue>nGValue?(nRValue>nBValue?nRValue:nBValue):(nGValue>nBValue?nGValue:nBValue);
- if(nAlphaRatio != 0)
- {
- nAValue = nAlphaRatio;
- }
- else
- {
- nAValue= 0;
- }
- pData[nBeginPos] = (unsigned char)nRValue;
- pData[nBeginPos+ 1] = (unsigned char)nGValue;
- pData[nBeginPos + 2] = (unsigned char)nBValue;
- //修改原图的alpha值.
- pData[nBeginPos + 3] = (unsigned char)nAValue;
- }
- }
- CCTexture2D *pTexture = new CCTexture2D;
- pTexture->autorelease();
- pTexture->initWithImage(pImage);
- CCTexture2DPixelFormat ccpf = pTexture->getPixelFormat();
- CCAssert(ccpf == kTexture2DPixelFormat_RGBA8888, "your png file's pixel format is not RGBA8888 or not have alpha panel");
- CCSprite* pArrowSprite= new CCSprite();
- pArrowSprite->initWithTexture(pTexture);
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- pArrowSprite->setPosition(ccp(size.width/2 + 20, size.height/2 - 20));
- this->addChild(pArrowSprite, 6);
直接看代码注释就懂了。贴上效果图,打完收工。
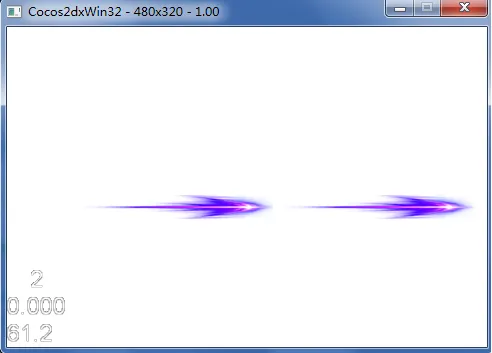