---------------------------------------------------------------------------------UITextView---------------------------------------------------------------------------------------
1:禁止 UITextView 拖动
textView.scrollEnabled = NO;
注:如果动态修改textView的Frame时,不设置为NO,输入光标的显示位置将不会友好.
2: - (void)textViewDidChangeSelection:(UITextView *)textView
修改光标位置代码:
NSRange range; range.location = 0; range.length = 0; textView.selectedRange = range;
注:手动调整光标位置时触发的委托,在第一次启动TextView编辑时,也会触发,会优先于键盘启动观察者事件,可以在这里区别是哪个TextView启动了键盘.
本委托的优先执行率高于 textViewDidBeginEditing 代理 也高于 UIKeyboardWillShowNotification 通知
3:监听TextView 点击了ReturnKey 按钮
- (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text { if ([text isEqualToString:@"\n"]) { //这里写按了ReturnKey 按钮后的代码 return NO; } return YES; }
4:为UITextView 添加 Placeholder
#import <UIKit/UIKit.h> @interface FEUITextView : UITextView @property(nonatomic) UILabel *placeHolderLabel; @property(nonatomic) NSString *placeholder; @property(nonatomic) UIColor *placeholderColor; @end
- (id)initWithCoder:(NSCoder *)aDecoder { self = [super initWithCoder:aDecoder]; if (self) { [self FE_defaultFunction]; } return self; } #pragma mark - Private Function - (void)FE_defaultFunction { [self setText:@""]; [self.layer setBorderWidth:1.0f]; [self.layer setBorderColor:[UIColor colorWithRed:196.0/255.0 green:196.0/255.0 blue:196.0/255.0 alpha:1.0].CGColor]; [self.layer setCornerRadius:5.0f]; [self setBackgroundColor:[UIColor whiteColor]]; [self setPlaceholder:@""]; [self setPlaceholderColor:[UIColor lightGrayColor]]; [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(FE_textChanged:) name:UITextViewTextDidChangeNotification object:nil]; } - (void)FE_textChanged:(NSNotification *)notification { if([[self placeholder] length] == 0) { return; } if([[self text] length] == 0) { [[self viewWithTag:999] setAlpha:1]; } else { [[self viewWithTag:999] setAlpha:0]; } } - (void)setText:(NSString *)text { [super setText:text]; [self FE_textChanged:nil]; } - (void)drawRect:(CGRect)rect { if( [[self placeholder] length] > 0 ) { if ( placeHolderLabel == nil ) { placeHolderLabel = [[UILabel alloc] initWithFrame:CGRectMake(8,8,self.bounds.size.width - 16,0)]; placeHolderLabel.lineBreakMode = UILineBreakModeWordWrap; placeHolderLabel.numberOfLines = 0; placeHolderLabel.font = self.font; placeHolderLabel.backgroundColor = [UIColor clearColor]; placeHolderLabel.textColor = self.placeholderColor; placeHolderLabel.alpha = 0; placeHolderLabel.tag = 999; [self addSubview:placeHolderLabel]; } placeHolderLabel.text = self.placeholder; [placeHolderLabel sizeToFit]; [self sendSubviewToBack:placeHolderLabel]; } if( [[self text] length] == 0 && [[self placeholder] length] > 0 ) { [[self viewWithTag:999] setAlpha:1]; } [super drawRect:rect]; }
5:键盘启动监听
//视图成功展现出来以后调用 - (void)viewDidAppear:(BOOL)animated { //监听键盘切换 [[NSNotificationCenter defaultCenter]addObserver:self selector:@selector(FE_keyboardWillShow:) name:UIKeyboardWillShowNotification object:nil]; [self.coopRemarkTextView becomeFirstResponder]; } //视图准备回收时调用 - (void)viewWillDisappear:(BOOL)animated { [self.coopRemarkTextView resignFirstResponder]; [UIView animateWithDuration:0.25 delay:0 options:0 animations:^{ [self.coopRemarkTextView setHelp_height:1]; } completion:^(BOOL finished) { }]; [[NSNotificationCenter defaultCenter] removeObserver:self]; } /** * @brief 当系统键盘被使用是触发此通知 * * @param notification 通知 */ - (void)FE_keyboardWillShow:(NSNotification*)notification { NSDictionary *userInfo = [notification userInfo]; NSValue* aValue = [userInfo objectForKey:UIKeyboardFrameEndUserInfoKey]; CGRect keyboardRect = [aValue CGRectValue]; CGRect keyboardFrame = [self.view convertRect:keyboardRect fromView:[[UIApplication sharedApplication] keyWindow]]; CGFloat keyboardHieght =keyboardFrame.size.height ;//这里记录键盘启动或者是切换其他类型的键盘时 当前View相对键盘的Y轴 [UIView animateWithDuration:0.25 delay:0 options:0 animations:^{ [self.coopRemarkTextView setHelp_height:self.view.Help_height - keyboardHieght-24]; } completion:^(BOOL finished) { }]; }
---------------------------------------------------------------------------------UITextView---------------------------------------------------------------------------------------
---------------------------------------------------------------------------------UITextField---------------------------------------------------------------------------------------
1: 监听 UITextField 值变动事件
[searchTextField addTarget:self action:@selector(FE_fieldValueChange:) forControlEvents:UIControlEventEditingChanged];
2:将输入值显示成保密状态
self.password.secureTextEntry = YES;
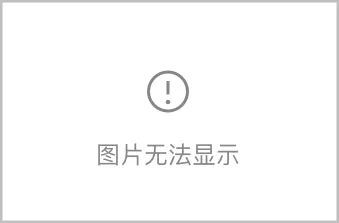
3:修改UITextField 输入光标的边距(类似Padding的概念)位置(参考:UITextField设置padding)
- (void)Parent_setTextCursorWithLeftPadding:(CGFloat)paddingLeft withRightPadding:(CGFloat)paddingRight { self.Parent_paddingLeft = paddingLeft; self.Parent_paddingRight = paddingRight; } - (CGRect)textRectForBounds:(CGRect)bounds { return CGRectMake(bounds.origin.x + self.Parent_paddingLeft, bounds.origin.y , bounds.size.width - self.Parent_paddingRight, bounds.size.height); } - (CGRect)editingRectForBounds:(CGRect)bounds { return [self textRectForBounds:bounds]; }
4:去掉键盘输入时的辅助提示
self.testTextField.autocorrectionType = UITextAutocorrectionTypeNo;
5:设置UITextField在空值时禁止Return Key的使用
self.testTextField.enablesReturnKeyAutomatically = YES;
6:设置键盘风格
self.testTextField.keyboardAppearance = UIKeyboardAppearanceAlert;//黑色风格 目前只有两种
7:为UITextField加入清除输入值按钮
[_flowCalendarTimeInputView setClearButtonMode:UITextFieldViewModeWhileEditing];
8:修复UITextField 当前光标所在位置 (参考:光标移动)
- (void)Help_moveCursorWithDirection:(UITextLayoutDirection)direction offset:(NSInteger)offset { UITextRange *range = self.selectedTextRange; UITextPosition* start = [self positionFromPosition:range.start inDirection:direction offset:offset]; if (start) { [self setSelectedTextRange:[self textRangeFromPosition:start toPosition:start]]; } }
注:1:所入光标移动的方向 和 位置值
2:如果需要在键盘刚启动时就修改键盘光标位置请在 becomeFirstResponder前调用
[_flowCalendarTimeInputView becomeFirstResponder]; [_flowCalendarTimeInputView Help_moveCursorWithDirection:UITextLayoutDirectionLeft offset:1];
9:基于第八点技术实现手工输入时间数字校验代码:
第一步: 先设置委托和监听
[_flowCalendarTimeInputView setDelegate:self]; [_flowCalendarTimeInputView addTarget:self action:@selector(Flow_inputTimeValueChange:) forControlEvents:UIControlEventEditingChanged];第二步:实现
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string { if ([string isEqualToString:@""]) { return NO; } switch (textField.text.length) { case 1: if ([string intValue] > 2) { return NO; } break; case 2: if ([string intValue] > 3) { return NO; } break; case 3: if ([string intValue] > 5) { return NO; } break; case 4: break; default: break; } return YES; } - (void)Flow_inputTimeValueChange:(UITextField *)textField { if (textField.text.length == 0) { [textField setText:@":"]; [textField Help_moveCursorWithDirection:UITextLayoutDirectionLeft offset:1]; } if (textField.text.length == 5) { [self setFlowCalendarSelectedDate:[NSDate Help_dateWithDateString:[NSString stringWithFormat:@"2012-11-01 %@:00",textField.text]] modifyMode:FlowCalendarSelectedDateModifyModeTime]; } if (textField.text.length >= 6) { textField.text = [textField.text substringWithRange:(NSRange){0,textField.text.length-1}]; } if (textField.text.length == 3) { [textField Help_moveCursorWithDirection:UITextLayoutDirectionRight offset:1]; } }
10:为 键盘类型: UIKeyboardTypeNumberPad 添加 完成按钮(参考:http://www.winddisk.com/2012/04/06/uikeyboardtypenumberpad_add_returnkey/)
一:注册监听事件:
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(Flow_keyboardDidShow:) name:UIKeyboardDidShowNotification object:nil];
/** * @brief 当系统键盘展现出来以后触发此通知 * * @param notification 通知 */ - (void)Flow_keyboardDidShow:(NSNotification*)notification { [self Flow_addFinishButtonToKeyboard]; }那么当键盘展现出来时,就会触发这个方法:
二:添加完成按钮方法:
- (void)Flow_addFinishButtonToKeyboard { // create custom button UIButton *doneButton = [UIButton buttonWithType:UIButtonTypeCustom]; doneButton.frame = CGRectMake(0, 163, 106, 53); doneButton.adjustsImageWhenHighlighted = NO; [doneButton setImage:[UIImage imageNamed:@"DoneUp3.png"] forState:UIControlStateNormal]; [doneButton setImage:[UIImage imageNamed:@"DoneDown3.png"] forState:UIControlStateHighlighted]; [doneButton addTarget:self action:@selector(Flow_finishSelectedAction:) forControlEvents:UIControlEventTouchUpInside]; // locate keyboard view UIWindow* tempWindow = [[[UIApplication sharedApplication] windows] objectAtIndex:1]; UIView* keyboard; for(int i=0; i<[tempWindow.subviews count]; i++) { keyboard = [tempWindow.subviews objectAtIndex:i]; if([[keyboard description] hasPrefix:@"<UIPeripheralHost"] == YES) [keyboard addSubview:doneButton]; } }找到keywindow后再依次找到键盘视图的原理
三:不使用的时候必须撤销这个键盘监听事件,否则影响其他视图键盘的使用
[[NSNotificationCenter defaultCenter] removeObserver:self];
11:设置UITextField 水平居中,垂直居中
[_flowCalendarTimeInputView setTextAlignment:NSTextAlignmentCenter]; [_flowCalendarTimeInputView setContentVerticalAlignment:UIControlContentVerticalAlignmentCenter];
---------------------------------------------------------------------------------UITextField---------------------------------------------------------------------------------------