BulkCopy.ps1
#BulkCopy.ps1
Set-Location "C:/Logs"
$files=Get-ChildItem |where {$_.extension -eq ".log"}
foreach ($file in $files) {
$filename=($file.FullName).ToString()
$arr=@($filename.split("."))
$newname=$arr[0]+".old"
Write-Host "copying "$file.Fullname "to"$newname
copy $file.fullname $newname -force
}
BulkRename.ps1
#BulkRename.ps1
Set-Location "C:/Logs"
$files=get-childitem -recurse |where {$_.extension -eq ".Log"}
foreach ($file in $files) {
$filename=($file.name).ToString()
$arr=@($filename.split("."))
$newname=$arr[0]+".old"
Write-Host "renaming"$file.Fullname "to"$newname
ren $file.fullname $newname -force
}
ListWMIProperties.ps1
#ListWMIProperties.ps1
$class=Read-Host "Enter a Win32 WMI Class that you are interested in"
$var=get-WMIObject -class $class -namespace "root/Cimv2"
$properties=$var | get-member -membertype Property
Write-Host "Properties for "$Class.ToUpper()
foreach ($property in $properties) {$property.name}
ListWMIValues.ps1
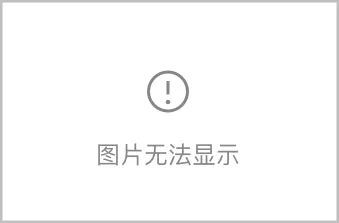 |
#ListWMIValues.ps1
$class=Read-Host "Enter a Win32 WMI Class that you are interested in"
$var=get-WMIObject -class $class -namespace "root/CimV2"
$properties=$var | get-member -membertype Property
Write-Host -foregroundcolor "Yellow" "Properties for "$Class.ToUpper()
# if more than one instance was returned then $var will be an array
# and we need to loop through it
$i=0
if ($var.Count -ge 2) {
do {
foreach ($property in $properties) {
#only display values that aren't null and don't display system
#properties that start with __
if ($var[$i].($property.name) -ne $Null -AND `
$property.name -notlike "__*") {
write-Host -foregroundcolor "Green" `
$property.name"="$var[$i].($property.name)
}
}
Write-Host "***********************************"
#divider between instances
$i++
}while($i -le ($var.count-1))
}
# else $var has only one instance
else {
foreach ($property in $properties) {
if ($var.($property.name) -ne $Null -AND `
$property.name -notlike "__*") {
write-Host -foregroundcolor "Green" `
$property.name"="$var.($property.name)
}
}
}
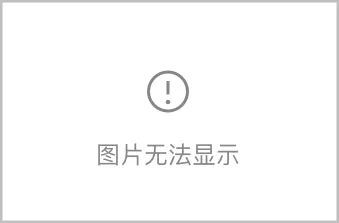 |
WMIReport.ps1
#WMIReport.ps1
$OS=Get-WmiObject -class win32_operatingsystem `
| Select-Object Caption,CSDVersion
#select fixed drives only by specifying a drive type of 3
$Drives=Get-WmiObject -class win32_logicaldisk | `
where {$_.DriveType -eq 3}
Write-Host "Operating System:" $OS.Caption $OS.CSDVersion
#Write-Host `n
Write-Host "Drive Summary:"
write-Host "Drive"`t"Size (MB)"`t"FreeSpace (MB)"
foreach ($d in $Drives) {
$free="{0:N2}" -f ($d.FreeSpace/1048576)
$size="{0:N0}" -f ($d.size/1048576)
Write-Host $d.deviceID `t $size `t $free
}
GetOwnerReport.ps1
#GetOwnerReport
$report="C:/OwnerReport.csv"
$StartingDir=Read-Host "What directory do you want to start at?"
Get-ChildItem $StartingDir -recurse |Get-Acl `
| select Path,Owner | Export-Csv $report -NoTypeInformation
#send two beeps when report is finished
write-Host `a `a `n"Report finished. See "$report
ChangeACL.ps1
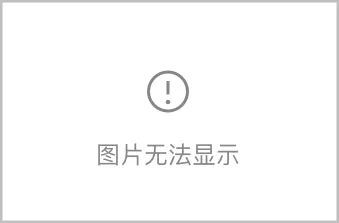 |
#ChangeACL.ps1
$Right="FullControl"
#The possible values for Rights are
# ListDirectory
# ReadData
# WriteData
# CreateFiles
# CreateDirectories
# AppendData
# ReadExtendedAttributes
# WriteExtendedAttributes
# Traverse
# ExecuteFile
# DeleteSubdirectoriesAndFiles
# ReadAttributes
# WriteAttributes
# Write
# Delete
# ReadPermissions
# Read
# ReadAndExecute
# Modify
# ChangePermissions
# TakeOwnership
# Synchronize
# FullControl
$StartingDir=Read-Host " What directory do you want to start at?"
$Principal=Read-Host " What security principal do you want to grant" `
"$Right to? `n Use format domain/username or domain/group"
#define a new access rule
#the $rule line has been artificially broken for print purposes
#It needs to be one line. The online version of the script is properly
#formatted.
$rule=new-object System.Security.AccessControl.FileSystemAccessRule
($Principal,$Right,"Allow")
foreach ($file in $(Get-ChildItem $StartingDir -recurse)) {
$acl=get-acl $file.FullName
#display filename and old permissions
write-Host -foregroundcolor Yellow $file.FullName
#uncomment if you want to see old permissions
#write-Host $acl.AccessToString `n
#Add this access rule to the ACL
$acl.SetAccessRule($rule)
#Write the changes to the object
set-acl $File.Fullname $acl
#display new permissions
$acl=get-acl $file.FullName
Write-Host -foregroundcolor Green "New Permissions"
Write-Host $acl.AccessToString `n
}
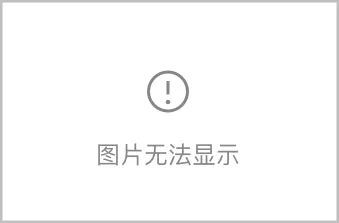 |