1、接口开发:
1、全选接口开发:
*Controller
:
//全选
@RequestMapping("select_all.do")
@ResponseBody
public ServerResponse<CartVo> selectAll(HttpSession session){
User user =(User) session.getAttribute(Const.CURRENT_USER);
if(user == null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),ResponseCode.NEED_LOGIN.getDesc());
}
return iCartService.selectOrUnselectAll(user.getId(),Const.Cart.CHECKED,null);
}
*Service
:
//购物车选或不选
ServerResponse<CartVo> selectOrUnselectAll(Integer userId,Integer checked,Integer productId);
*ServiceImpl
:
//购物车全选或全不选
public ServerResponse<CartVo> selectOrUnselectAll(Integer userId,Integer checked,Integer productId){
cartMapper.checkedOrUncheckedProduct(userId,checked,productId);
return this.list(userId);
}
checkedOrUncheckedProduct
方法:*Mapper
:
//全选购物车中的商品或者不全选购物车中的商品
int checkedOrUncheckedProduct(@Param("userId") Integer userId,@Param("checked") Integer checked,@Param("productId") Integer productId);
*Mappler.xml
:
<update id="checkedOrUncheckedProduct" parameterType="map">
update mmall_cart
set checked = #{checked},
update_time=now()
where user_id=#{userId}
<if test="productId != null">
and product_id=#{productId}
</if>
</update>
上面Impl
方法中的list
方法在上一篇文章中:20、【购物车模块】——更新、删除、查询购物车功能开发获取购物车信息列表的时候又讲~
2、全反选接口开发:
*Controller
:
//全反选
@RequestMapping("un_select_all.do")
@ResponseBody
public ServerResponse<CartVo> unSelectAll(HttpSession session,Integer productId){
User user =(User) session.getAttribute(Const.CURRENT_USER);
if(user == null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),ResponseCode.NEED_LOGIN.getDesc());
}
return iCartService.selectOrUnselectAll(user.getId(),Const.Cart.UN_CHECKED,null);
}
*Service
:
//购物车选或不选
ServerResponse<CartVo> selectOrUnselectAll(Integer userId,Integer checked,Integer productId);
*ServiceImpl
:
//购物车全选或全不选
public ServerResponse<CartVo> selectOrUnselectAll(Integer userId,Integer checked,Integer productId){
cartMapper.checkedOrUncheckedProduct(userId,checked,productId);
return this.list(userId);
}
checkedOrUncheckedProduct
方法:*Mapper
:
//全选购物车中的商品或者不全选购物车中的商品
int checkedOrUncheckedProduct(@Param("userId") Integer userId,@Param("checked") Integer checked,@Param("productId") Integer productId);
*Mappler.xml
:
<update id="checkedOrUncheckedProduct" parameterType="map">
update mmall_cart
set checked = #{checked},
update_time=now()
where user_id=#{userId}
<if test="productId != null">
and product_id=#{productId}
</if>
</update>
其实全选不全反选接口是差不多的,只是在现在的是否选中的状态进行了判断~
3、单选接口开发:
*Controller
:
//单独选
@RequestMapping("select.do")
@ResponseBody
public ServerResponse<CartVo> Select(HttpSession session,Integer productId){
User user =(User) session.getAttribute(Const.CURRENT_USER);
if(user == null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),ResponseCode.NEED_LOGIN.getDesc());
}
return iCartService.selectOrUnselectAll(user.getId(),Const.Cart.CHECKED,productId);
}
4、单反选接口开发:
//单独反选
@RequestMapping("un_select.do")
@ResponseBody
public ServerResponse<CartVo> unSelect(HttpSession session,Integer productId){
User user =(User) session.getAttribute(Const.CURRENT_USER);
if(user == null){
return ServerResponse.createByErrorCodeMessage(ResponseCode.NEED_LOGIN.getCode(),ResponseCode.NEED_LOGIN.getDesc());
}
return iCartService.selectOrUnselectAll(user.getId(),Const.Cart.UN_CHECKED,productId);
}
其中单选,单反选同意也是用了上面selectOrUnselectAll
方法,只是传递的参数不同~
5、查询购物车数量接口开发:
*Controller
:
//查询当前用户购物车的数量,如果一个产品数量有十个,我们显示是1个
@RequestMapping("get_cart_product_count.do")
@ResponseBody
public ServerResponse<Integer> getCartProductCount(HttpSession session){
User user =(User) session.getAttribute(Const.CURRENT_USER);
if(user == null){
return ServerResponse.createBySuccess(0);
}
return iCartService.getCartProductCount(user.getId());
}
*Service
:
//获取用户购物车中商品数量
ServerResponse<Integer> getCartProductCount(Integer userId);
*ServiceImpl
:
//获取用户购物车中商品数量
public ServerResponse<Integer> getCartProductCount(Integer userId){
if(userId == null){
return ServerResponse.createBySuccess(0);
}
return ServerResponse.createBySuccess(cartMapper.selectProductCountByUserId(userId));
}
*Mapper
:
//根据用户Id查询购物车中商品的数量
int selectProductCountByUserId(Integer userId);
*Mappler.xml
:
<select id="selectProductCountByUserId" resultType="int" parameterType="int" >
select IFNULL(sum(quantity),0) as count from mmall_cart where user_id = #{userId}
</select>
2、接口测试:
1、全选接口测试:
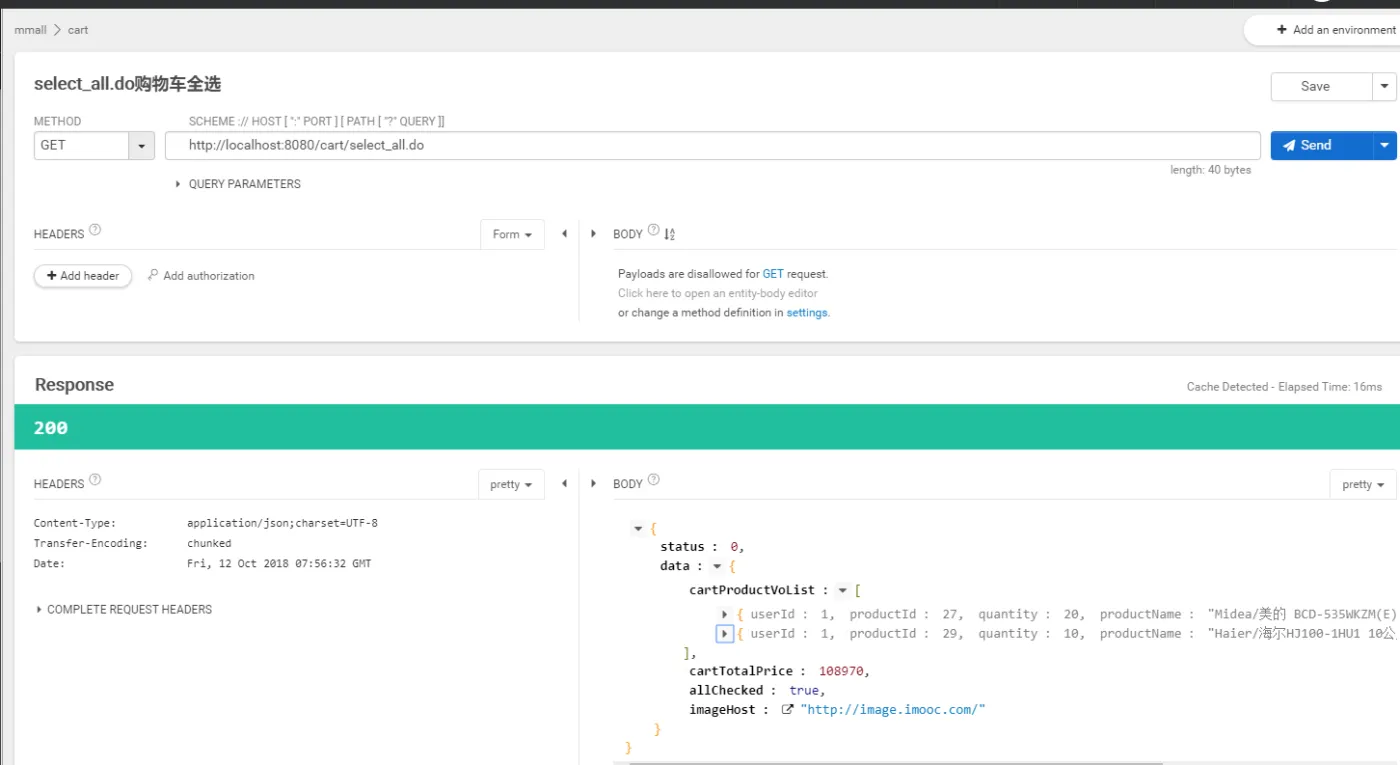
image.png
2、全反选接口测试:
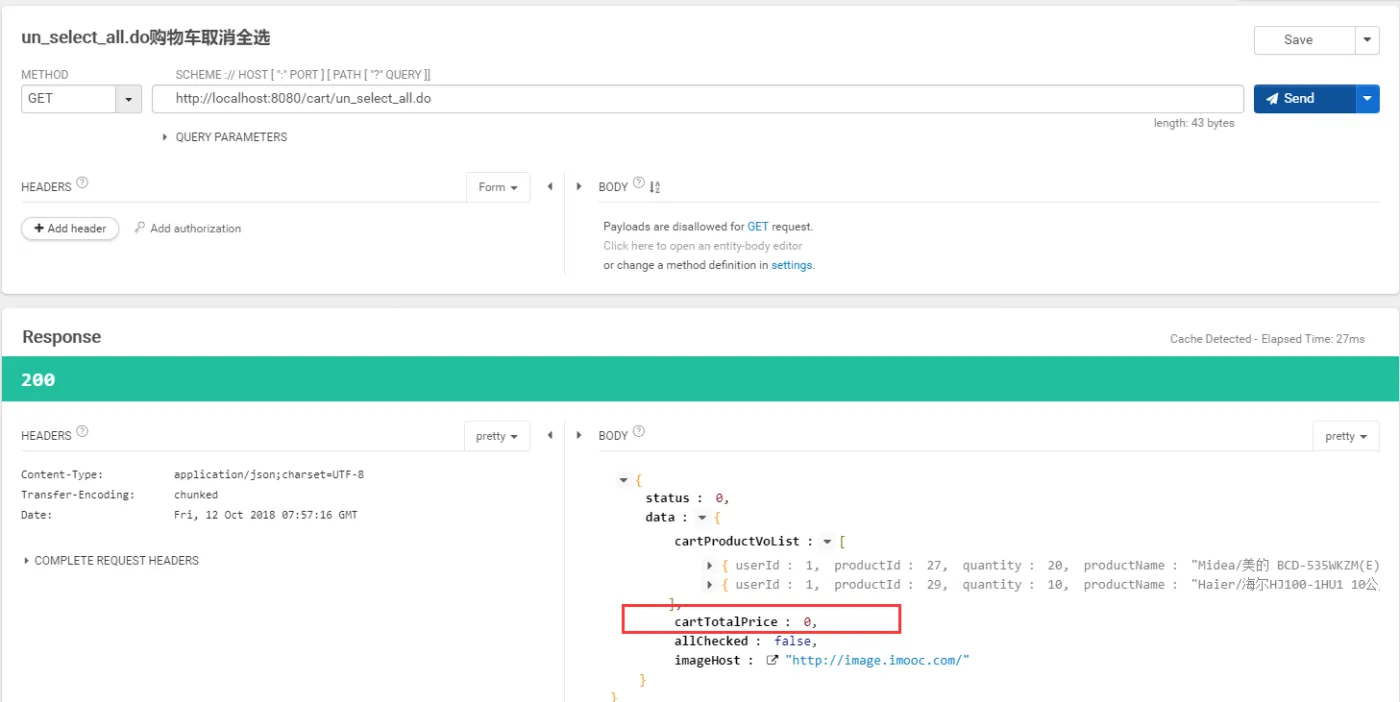
image.png
3、单选接口测试:

image.png
4、单反选接口测试:
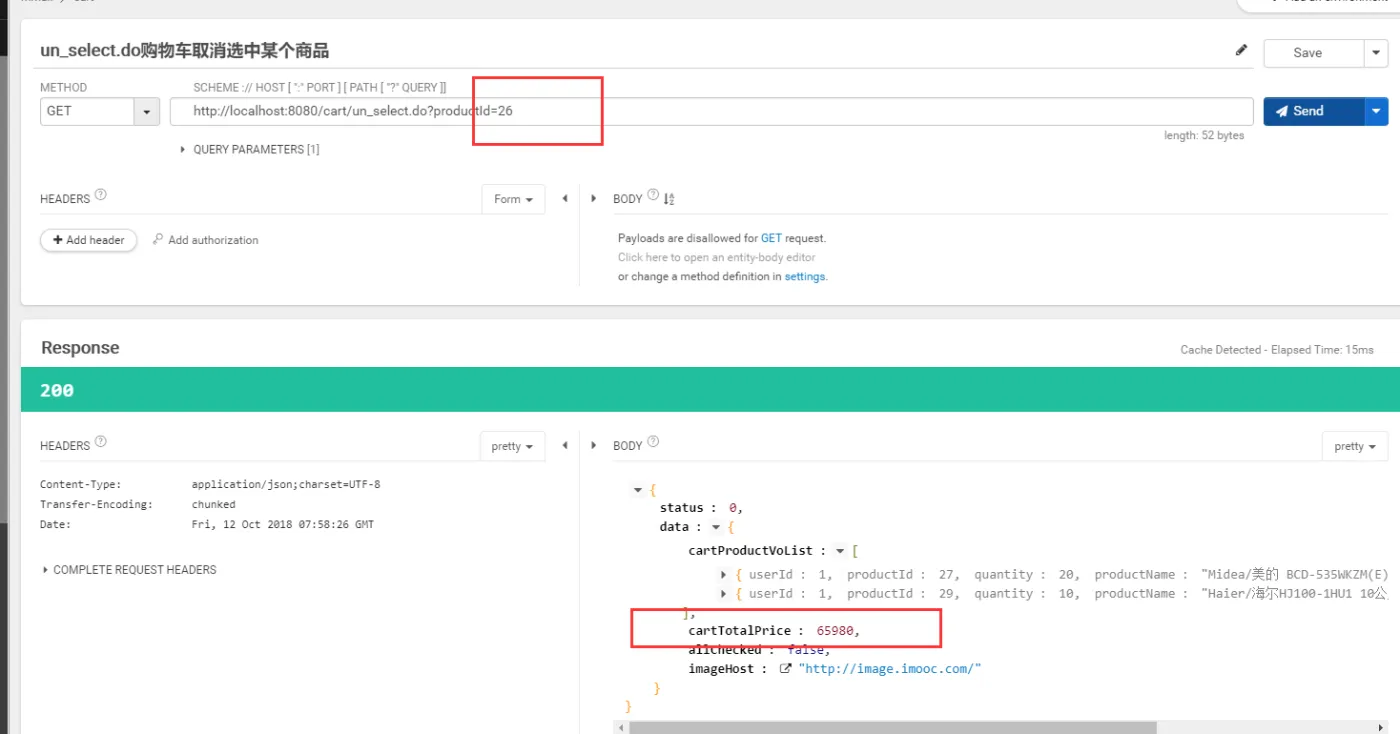
image.png
5、获取购物车数量接口测试:

image.png