- activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.mazaiting.expandablelistview.MainActivity"
>
<ExpandableListView
android:id="@+id/main_expandable_list_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
</RelativeLayout>
- layout_parent.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
>
<TextView
android:id="@+id/tv_show_parent"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_gravity="center"
android:gravity="center" />
</LinearLayout>
- layout_child.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
>
<TextView
android:id="@+id/tv_show_child"
android:layout_width="match_parent"
android:layout_height="40dp"
android:layout_gravity="center"
android:gravity="center" />
</LinearLayout>
- MainActivity.java
public class MainActivity extends AppCompatActivity {
private ExpandableListView mExpandableListView = null;
private List<String> mList = null;
private Map<String, List<String>> mMap = null;
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mExpandableListView = (ExpandableListView) this.findViewById(R.id.main_expandable_list_view);
initData();
mExpandableListView.setAdapter(new MyAdapter());
mExpandableListView.setOnChildClickListener(new ExpandableListView.OnChildClickListener() {
@Override public boolean onChildClick(ExpandableListView parent, View v, int groupPosition,
int childPosition, long id) {
mExpandableListView.collapseGroup(groupPosition);
return true;
}
});
}
/**
* 初始化数据
*/
private void initData() {
mList = new ArrayList<>();
mList.add("parent1");
mList.add("parent2");
mList.add("parent3");
mMap = new HashMap<>();
List<String> list1 = new ArrayList<>();
list1.add("child1-1");
list1.add("child1-2");
list1.add("child1-3");
mMap.put(mList.get(0), list1);
List<String> list2 = new ArrayList<>();
list2.add("child2-1");
list2.add("child2-2");
list2.add("child2-3");
mMap.put(mList.get(1), list2);
List<String> list3 = new ArrayList<>();
list3.add("child3-1");
list3.add("child3-2");
list3.add("child3-3");
mMap.put(mList.get(2), list3);
}
class MyAdapter extends BaseExpandableListAdapter{
/**获取父条目个数*/
@Override public int getGroupCount() {
return mList.size();
}
/**获取当前条目下的子条目数据*/
@Override public int getChildrenCount(int groupPosition) {
String string = mList.get(groupPosition);
int size = mMap.get(string).size();
return size;
}
/**获取父条目对象*/
@Override public Object getGroup(int groupPosition) {
return mList.get(groupPosition);
}
/**获取指定位置的子条目*/
@Override public Object getChild(int groupPosition, int childPosition) {
String string = mList.get(groupPosition);
String child = mMap.get(string).get(childPosition);
return child;
}
/**获取父条目id*/
@Override public long getGroupId(int groupPosition) {
return groupPosition;
}
/**获取子条目id*/
@Override public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
/**是否稳定*/
@Override public boolean hasStableIds() {
return true;
}
/**绑定父条目布局*/
@Override public View getGroupView(int groupPosition, boolean isExpanded, View convertView,
ViewGroup parent) {
if (null == convertView){
convertView = LayoutInflater.from(MainActivity.this).inflate(R.layout.layout_parent, null);
}
TextView textView = (TextView) convertView.findViewById(R.id.tv_show_parent);
textView.setText(mList.get(groupPosition));
return convertView;
}
/**绑定子条目布局*/
@Override public View getChildView(int groupPosition, int childPosition, boolean isLastChild,
View convertView, ViewGroup parent) {
if (null == convertView){
convertView = LayoutInflater.from(MainActivity.this).inflate(R.layout.layout_child, null);
}
TextView textView = (TextView) convertView.findViewById(R.id.tv_show_child);
String string = mList.get(groupPosition);
String result = mMap.get(string).get(childPosition);
textView.setText(result);
return convertView;
}
/**是否有子条目被选择*/
@Override public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
效果:
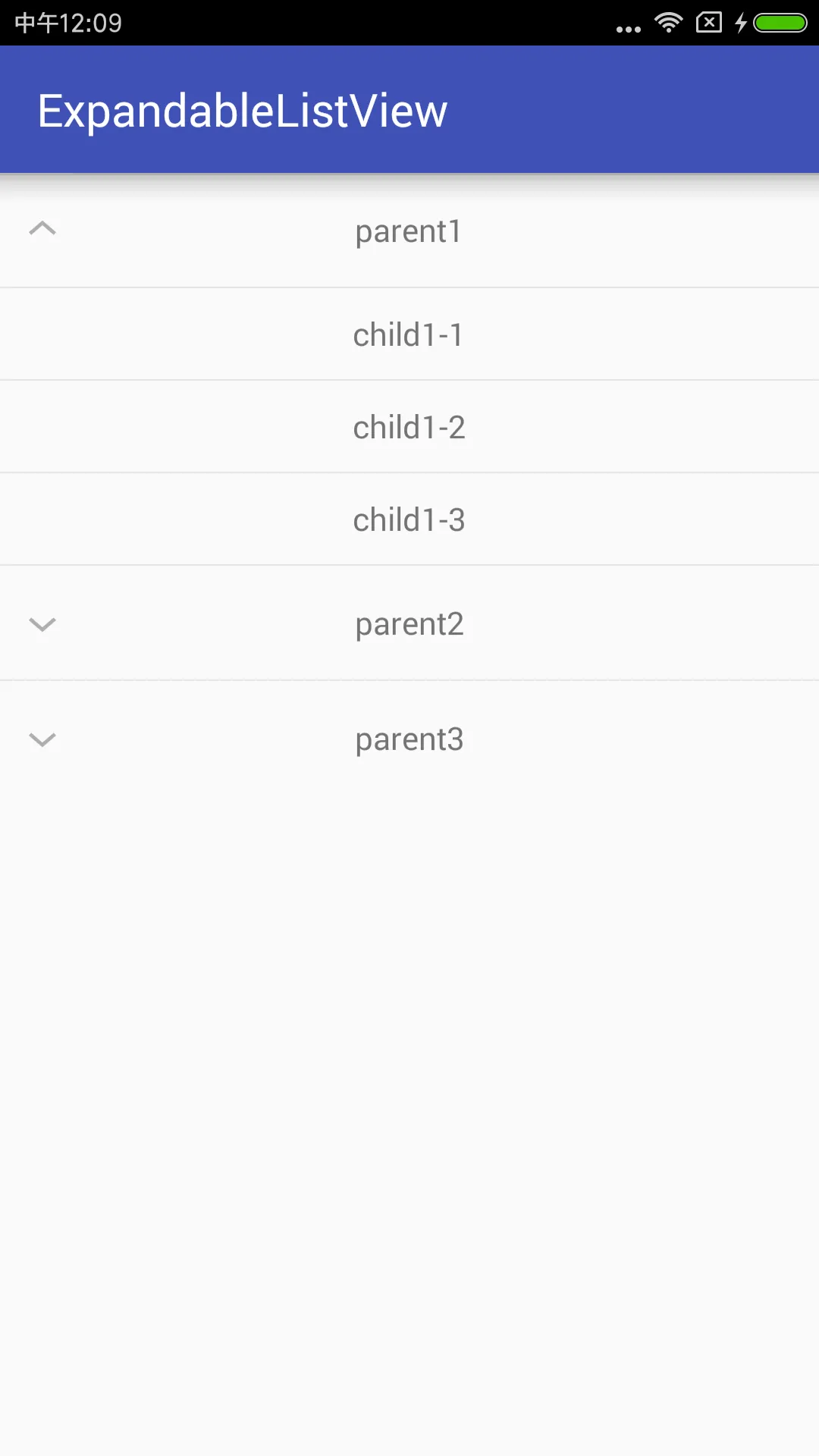
效果.png