虽然下一个项目需要使用xfire,但是在查资料的过程中还是看到有不少地方都说cxf比xfire更好,cxf继承了xfire,但是不仅仅包含xfire,因此便也一起来尝试尝试。大概是有了xfire的经验吧,cxf的搭建比xfire快了许多。
cxf的许多参数感觉和xfire差不多,因此便不做太多的解释,如果不明白的可以参考之前的xfire搭建来促进理解。
搭建过程如下:
1、使用eclipse创建一个maven项目,maven导包的pom.xml的整体内容如下:
<project xmlns="
http://maven.apache.org/POM/4.0.0
" xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance
"
xsi:schemaLocation="
http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cxfTest</groupId>
<artifactId>cxfTest</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>cxfTest Maven Webapp</name>
<url>
http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-core</artifactId>
<version>3.0.4</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>4.1.6.RELEASE</version>
</dependency>
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-rt-frontend-jaxws</artifactId>
<version>3.0.4</version>
</dependency>
</dependencies>
<build>
<finalName>cxfTest</finalName>
</build>
</project>
2、创建webService服务类的接口:
package cxfTest;
import javax.jws.WebService;
@WebService(serviceName = "CxfTestService", targetNamespace = "
http://cxfTest")
public interface CxfTestService {
public String getUserName();
}
3、实现类代码,@Service实际上是spring注解:
package cxfTest.cxfTestImp;
import javax.jws.WebService;
import org.springframework.stereotype.Service;
import cxfTest.CxfTestService;
@Service("CxfTestService")
@WebService(targetNamespace = "
http://cxfTest")
public class CxfTestServiceImp implements CxfTestService {
@Override
public String getUserName() {
return "name:cxfTest";
}
}
4、cxf配置文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="
http://www.springframework.org/schema/beans"
xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="
http://www.w3.org/2001/XMLSchema"
xmlns:context="
http://www.springframework.org/schema/context"
xmlns:jaxws="
http://cxf.apache.org/jaxws"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
<context:component-scan base-package="cxfTest"/>
<jaxws:server id="getUserName"
serviceClass="cxfTest.CxfTestService"
address="/CxfTestService">
<jaxws:serviceBean>
<bean
class="cxfTest.cxfTestImp.CxfTestServiceImp"></bean>
</jaxws:serviceBean>
</jaxws:server>
</beans>
这里需要注意的是,一般实际应用时可能会加上下边的代码,这两每当有客户端访问的时候都会把相关的日志打印出来:
<jaxws:inInterceptors>
<bean class="org.apache.cxf.interceptor.LoggingInInterceptor"></bean>
</jaxws:inInterceptors>
<jaxws:outInterceptors>
<bean class="org.apache.cxf.interceptor.LoggingOutInterceptor"></bean>
</jaxws:outInterceptors>
这段代码加在
<jaxws:server>和</jaxws:server>中间。
5、web.xml文件:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance" xmlns="
http://java.sun.com/xml/ns/javaee" xmlns:web="
http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="
http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:cxf-context.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<servlet>
<servlet-name>CXFServlet</servlet-name>
<servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>CXFServlet</servlet-name>
<url-pattern>/services/*</url-pattern>
</servlet-mapping>
</web-app>
6、把项目加入到tomcat中启动,然后在浏览器访问
http://localhost:8082/cxfTest/services,看到页面如下:
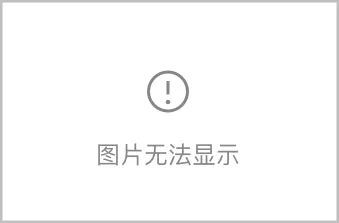
7、页面正常访问后,我新建一个一个项目,导入了服务端导入的所有的jar包,然后写了个简单的main方法进行测试。需要注意的是,跟xfire一样,这里也需要写一个和服务端一模一样的服务接口(包路径可以不一样),如:
package cxfTest1;
import javax.jws.WebService;
@WebService(serviceName = "CxfTestService", targetNamespace = "
http://cxfTest")
public interface CxfTestService {
public String getUserName();
}
然后对应的模拟客户端调用代码如下:
package cxfTest1;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
public class CxfTest {
public static void main(String[] args) {
JaxWsProxyFactoryBean factoryBean = new JaxWsProxyFactoryBean();
// factoryBean.getInInterceptors().add(new LoggingInInterceptor());
// factoryBean.getOutInterceptors().add(new LoggingOutInterceptor());
factoryBean.setServiceClass(CxfTestService.class);
factoryBean
.setAddress("
http://localhost:8082/cxfTest/services/CxfTestService");
CxfTestService impl = (CxfTestService) factoryBean.create();
System.out.println(impl.getUserName());
}
}
上边被注释的代码也是日志相关的,加不加都不影响主要业务功能,可以自己选择用还是不用。执行main方法后控制台输出如下:
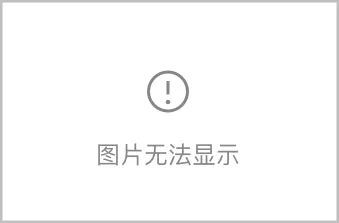