1、场景
实现一个聊天室功能,聊城室就是一个中介者,参与聊天的人就是同事对象
,
如下图所示
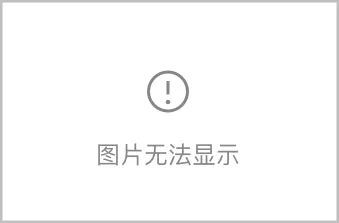
AbstractChatroom
类
:抽象聊天室类,做为
Participant
的交互的中介。
Register()
方法
:
会员注册功能;
Send()
方法:发送消息功能。
Chatroom
类
:具体聊天室类,实现抽象聊天室类中的方法。
Participant
类
:参与者类,主要有发送消息
Send()
功能和接受消息
Receive()
功能。
Beatle
类,NonBeatle
类
:参与者类的具体实现,实现父类
Paticipant
类中的方法。
2、代码
1
、抽象聊天室类
AbstractChatroom
及其具体聊天室
Chatroom
|
///
<summary>
///
The 'Mediator' abstract class
///
</summary>
abstract
class AbstractChatroom
{
public abstract void
Register(Participant
participant);
public abstract void
Send(string
from, string
to, string
message);
}
///
<summary>
///
The 'ConcreteMediator' class
///
</summary>
class
Chatroom
: AbstractChatroom
{
private Dictionary
<string
, Participant
> _participants =new Dictionary
<string
, Participant
>();
public override void
Register(Participant
participant)
{
if
(!_participants.ContainsValue(participant))
{
_participants[participant.Name] = participant;
}
participant.Chatroom = this
;
}
public override void
Send(string
from, string
to, string
message)
{
Participant
participant = _participants[to];
if
(participant != null
)
{
participant.Receive(from, message);
}
}
}
|
2
、参与者
Participant
类及其实现
Beatle
、NonBeatle
|
///
<summary>
///
The 'AbstractColl
eague' class
///
</summary>
class
Participant
{
private Chatroom
_chatroom;
private string
_name;
// Constructor
public
Participant(string
name)
{
this
._name = name;
}
// Gets participant name
public string
Name
{
get
{ return
_name; }
}
// Gets chatroom
public Chatroom
Chatroom
{
set
{ _chatroom = value
; }
get
{ return
_chatroom; }
}
// Sends message to given participant
public void
Send(string
to, string
message)
{
_chatroom.Send(_name, to, message);
}
// Receives message from given participant
public virtual void
Receive(string
from, string
message)
{
Console
.WriteLine("{0} to {1}: '{2}'"
,from, Name, message);
}
}
///
<summary>
///
A 'ConcreteColl
eague' class
///
</summary>
class
Beatle
: Participant
{
// Constructor
public
Beatle(string
name)
: base
(name)
{
}
public override void
Receive(string
from, string
message)
{
Console
.Write("To a Beatle: "
);
base
.Receive(from, message);
}
}
///
<summary>
///
A 'ConcreteColl
eague' class
///
</summary>
class
NonBeatle
: Participant
{
// Constructor
public
NonBeatle(string
name)
: base
(name)
{
}
public override void
Receive(string
from, string
message)
{
Console
.Write("To a non-Beatle: "
);
base
.Receive(from, message);
}
}
|
3
、客户端代码
|
static
void Main
(string
[] args)
{
// Create chatroom
Chatroom
chatroom = new Chatroom
();
// Create participants and register them
Participant
George = new Beatle
("George"
);
Participant
Paul = new Beatle
("Paul"
);
Participant
Ringo = new Beatle
("Ringo"
);
Participant
John = new Beatle
("John"
);
Participant
Yoko = new NonBeatle
("Yoko"
);
chatroom.Register(George);
chatroom.Register(Paul);
chatroom.Register(Ringo);
chatroom.Register(John);
chatroom.Register(Yoko);
// Chatting participants
Yoko.Send("John"
, "Hi John!"
);
Paul.Send("Ringo"
, "All you need is love"
);
Ringo.Send("George"
, "My sweet Lord"
);
Paul.Send("John"
, "Can't buy me love"
);
John.Send("Yoko"
, "My sweet love"
);
// Wait for user
Console
.ReadKey();
}
|
3、运行结果
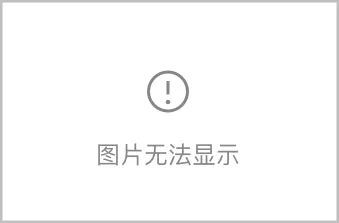
中介者模式(
Mediator Pattern
),定义一个中介对象来封装系列对象之间的交互。中介者使各个对象不需要显示地相互引用,从而使其耦合性松散,而且可以独立地改变他们之间的交互。中介者模式一般应用于一组对象以定义良好但是复杂的方法进行通信的场合,以及想定制一个分布在多个类中的行为,而不想生成太多的子类的场合。
本文转自 灵动生活 51CTO博客,原文链接:http://blog.51cto.com/smartlife/277270,如需转载请自行联系原作者