使用Silverlight 2的Canvas,写了一个动态创建Rectangle的示例,由于时间的原因所以难免有些不足之处,但程序功能都正常使用.用鼠标可以点击画布任何位置拖出一个矩形对象,松开鼠标即可完成一个矩形的创建!
程序运行效果:
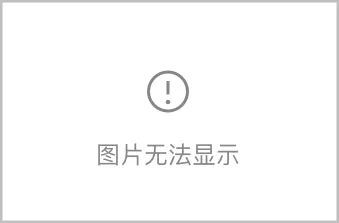
XAML代码:
<UserControl x:Class="Sample.dragrect"
xmlns=" http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x=" http://schemas.microsoft.com/winfx/2006/xaml"
Width="780" Height="400">
<StackPanel Background="Green"
Orientation="Horizontal">
<Canvas x:Name="LayoutRoot"
Background="GreenYellow"
Width="650" Height="400"
MouseMove="Canvas_MouseMove"
MouseLeftButtonDown="Canvas_MouseLeftButtonDown"
MouseLeftButtonUp="Canvas_MouseLeftButtonUp"/>
<StackPanel Background="Gold" Margin="10">
<TextBlock Text="选择颜色:"/>
<Button x:Name="btnRed"
Width="100" Height="50"
FontSize="20" Content="Red" Margin="5"
Click="btnRed_Click"/>
<Button x:Name="btnBlue"
Width="100" Height="50"
FontSize="20" Content="Blue" Margin="5"
Click="btnBlue_Click"/>
<Button x:Name="btnGreen"
Width="100" Height="50"
FontSize="20" Content="Green" Margin="5"
Click="btnGreen_Click"/>
<Button x:Name="btnClear"
Width="100" Height="50"
FontSize="20" Content="Clear" Margin="5"
Background="Red"
Click="btnClear_Click"/>
</StackPanel>
</StackPanel>
</UserControl>
C#代码:
xmlns=" http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x=" http://schemas.microsoft.com/winfx/2006/xaml"
Width="780" Height="400">
<StackPanel Background="Green"
Orientation="Horizontal">
<Canvas x:Name="LayoutRoot"
Background="GreenYellow"
Width="650" Height="400"
MouseMove="Canvas_MouseMove"
MouseLeftButtonDown="Canvas_MouseLeftButtonDown"
MouseLeftButtonUp="Canvas_MouseLeftButtonUp"/>
<StackPanel Background="Gold" Margin="10">
<TextBlock Text="选择颜色:"/>
<Button x:Name="btnRed"
Width="100" Height="50"
FontSize="20" Content="Red" Margin="5"
Click="btnRed_Click"/>
<Button x:Name="btnBlue"
Width="100" Height="50"
FontSize="20" Content="Blue" Margin="5"
Click="btnBlue_Click"/>
<Button x:Name="btnGreen"
Width="100" Height="50"
FontSize="20" Content="Green" Margin="5"
Click="btnGreen_Click"/>
<Button x:Name="btnClear"
Width="100" Height="50"
FontSize="20" Content="Clear" Margin="5"
Background="Red"
Click="btnClear_Click"/>
</StackPanel>
</StackPanel>
</UserControl>
C#代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace Sample
{
public partial class dragrect : UserControl
{
public dragrect()
{
InitializeComponent();
}
{
public partial class dragrect : UserControl
{
public dragrect()
{
InitializeComponent();
}
bool mouseMoveing = false;
Point mousePoint;
Color rectColor = Colors.Red;
Point mousePoint;
Color rectColor = Colors.Red;
private void Canvas_MouseMove(object sender, MouseEventArgs e)
{
//如果鼠标没有拖动矩形则返回
if (!mouseMoveing)
return;
//获取鼠标当前坐标
Point curPos = e.GetPosition(null);
//取得最小坐标值
double posX = mousePoint.X;
double posY = mousePoint.Y;
//计算矩形的宽和高
double rectWidth = Math.Abs(curPos.X - mousePoint.X);
double rectHeight = Math.Abs(curPos.Y - mousePoint.Y);
//创建一个矩形元素
Rectangle rect = new Rectangle();
//声明矩形的宽和高
rect.Width = rectWidth;
rect.Height = rectHeight;
//填充颜色
rect.Fill = new SolidColorBrush(rectColor);
//声明矩形在Canvas中创建的位置
Canvas.SetLeft(rect, posX);
Canvas.SetTop(rect, posY);
//添加矩形到Canvas中
LayoutRoot.Children.Add(rect);
}
{
//如果鼠标没有拖动矩形则返回
if (!mouseMoveing)
return;
//获取鼠标当前坐标
Point curPos = e.GetPosition(null);
//取得最小坐标值
double posX = mousePoint.X;
double posY = mousePoint.Y;
//计算矩形的宽和高
double rectWidth = Math.Abs(curPos.X - mousePoint.X);
double rectHeight = Math.Abs(curPos.Y - mousePoint.Y);
//创建一个矩形元素
Rectangle rect = new Rectangle();
//声明矩形的宽和高
rect.Width = rectWidth;
rect.Height = rectHeight;
//填充颜色
rect.Fill = new SolidColorBrush(rectColor);
//声明矩形在Canvas中创建的位置
Canvas.SetLeft(rect, posX);
Canvas.SetTop(rect, posY);
//添加矩形到Canvas中
LayoutRoot.Children.Add(rect);
}
private void Canvas_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
//获取当前的鼠标位置
mousePoint = e.GetPosition(null);
//开始创建矩形
mouseMoveing = true;
}
{
//获取当前的鼠标位置
mousePoint = e.GetPosition(null);
//开始创建矩形
mouseMoveing = true;
}
private void Canvas_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
//矩形创建完成
mouseMoveing = false;
}
{
//矩形创建完成
mouseMoveing = false;
}
private void btnRed_Click(object sender, RoutedEventArgs e)
{
//声明矩形颜色为Red
rectColor = Colors.Red;
}
{
//声明矩形颜色为Red
rectColor = Colors.Red;
}
private void btnBlue_Click(object sender, RoutedEventArgs e)
{
//声明矩形颜色为Blue
rectColor = Colors.Blue;
}
{
//声明矩形颜色为Blue
rectColor = Colors.Blue;
}
private void btnGreen_Click(object sender, RoutedEventArgs e)
{
//声明矩形颜色为Green
rectColor = Colors.Green;
}
{
//声明矩形颜色为Green
rectColor = Colors.Green;
}
private void btnClear_Click(object sender, RoutedEventArgs e)
{
//清除所有Canvas内的矩形元素
LayoutRoot.Children.Clear();
}
}
}
{
//清除所有Canvas内的矩形元素
LayoutRoot.Children.Clear();
}
}
}
本文转自dotfun 51CTO博客,原文链接:http://blog.51cto.com/dotfun/285833