样式很好理解,就像CSS里的一样,无需多加解释
1. 样式中的Setter
使用示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
<
Window
x:Class="DeepXAML.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:DeepXAML"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
Title="MainWindow" Height="250" Width="450">
<
Window.Resources
>
<
Style
TargetType="Button">
<
Setter
Property="FontSize" Value="30"></
Setter
>
<
Setter
Property="Margin" Value="10"></
Setter
>
</
Style
>
</
Window.Resources
>
<
StackPanel
>
<
Button
>New</
Button
>
<
Button
>Save</
Button
>
<
Button
>Exit</
Button
>
</
StackPanel
>
</
Window
>
<
a
href="http://images.cnblogs.com/cnblogs_com/cnblogsfans/201102/201102271111593876.png"><
img
style="background-image: none; border-right-width: 0px; margin: 0px; padding-left: 0px; padding-right: 0px; display: inline; border-top-width: 0px; border-bottom-width: 0px; border-left-width: 0px; padding-top: 0px" title="image" border="0" alt="image" src="https://images.cnblogs.com/cnblogs_com/cnblogsfans/201102/201102271112008793.png" width="244" height="137"></
a
>
|
很明显,使用样式让我们代码更精简,而且界面的外观可以集中处理。
2. 样式中的Trigger
当某些条件满足时,触发一个行为
a. 基本Trigger
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
<
Window
x:Class="DeepXAML.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:DeepXAML"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
Title="MainWindow" Height="250" Width="450">
<
Window.Resources
>
<
Style
TargetType="CheckBox">
<
Setter
Property="FontSize" Value="30"></
Setter
>
<
Setter
Property="Margin" Value="10"></
Setter
>
<
Style.Triggers
>
<
Trigger
Property="IsChecked" Value="true">
<
Trigger.Setters
>
<
Setter
Property="Foreground" Value="Red"></
Setter
>
</
Trigger.Setters
>
</
Trigger
>
</
Style.Triggers
>
</
Style
>
</
Window.Resources
>
<
StackPanel
>
<
CheckBox
>.Net</
CheckBox
>
<
CheckBox
>Java</
CheckBox
>
<
CheckBox
>Ruby</
CheckBox
>
<
CheckBox
>Python</
CheckBox
>
</
StackPanel
>
</
Window
>
|
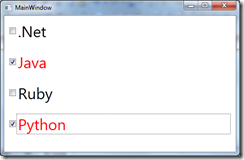
b. MultiTrigger
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
<
Window
x:Class="DeepXAML.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:DeepXAML"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
Title="MainWindow" Height="250" Width="450">
<
Window.Resources
>
<
Style
TargetType="CheckBox">
<
Setter
Property="FontSize" Value="30"></
Setter
>
<
Setter
Property="Margin" Value="10"></
Setter
>
<
Style.Triggers
>
<
MultiTrigger
>
<
MultiTrigger.Conditions
>
<
Condition
Property="IsChecked" Value="true"></
Condition
>
<
Condition
Property="Content" Value="Java"></
Condition
>
</
MultiTrigger.Conditions
>
<
MultiTrigger.Setters
>
<
Setter
Property="Foreground" Value="Red"></
Setter
>
</
MultiTrigger.Setters
>
</
MultiTrigger
>
</
Style.Triggers
>
</
Style
>
</
Window.Resources
>
<
StackPanel
>
<
CheckBox
>.Net</
CheckBox
>
<
CheckBox
>Java</
CheckBox
>
<
CheckBox
>Ruby</
CheckBox
>
<
CheckBox
>Python</
CheckBox
>
</
StackPanel
>
</
Window
>
|
c. DataTrigger
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
<
Window
x:Class="DeepXAML.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:DeepXAML"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
Title="MainWindow" Height="250" Width="450">
<
Window.Resources
>
<
Style
TargetType="TextBox">
<
Setter
Property="Margin" Value="10"></
Setter
>
<
Style.Triggers
>
<
DataTrigger
Binding="{Binding Path=Name}" Value="Jack">
<
Setter
Property="Foreground" Value="Red"></
Setter
>
</
DataTrigger
>
</
Style.Triggers
>
</
Style
>
</
Window.Resources
>
<
StackPanel
x:Name="stackPanel">
<
TextBox
Text="{Binding Path=Name}"></
TextBox
>
<
TextBox
Text="{Binding Path=Age}"></
TextBox
>
<
TextBox
></
TextBox
>
</
StackPanel
>
</
Window
>
|
1
2
3
4
5
6
7
8
9
10
11
12
13
|
public
MainWindow()
{
InitializeComponent();
Person p =
new
Person { Name =
"Jack"
, Age = 30 };
this
.stackPanel.DataContext = p;
}
public
class
Person
{
public
string
Name {
get
;
set
; }
public
int
Age {
get
;
set
; }
}
|
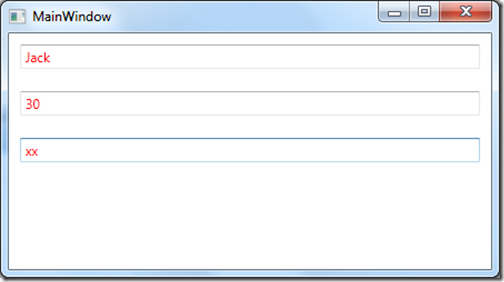
d. 多数据条件的trigger
MultiDataTrigger这个类似上面的MultiTrigger,不用细说了。
e. EventTrigger
是由事件来触发
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
<
Window
x:Class="DeepXAML.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:DeepXAML"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
Title="MainWindow" Height="250" Width="450">
<
Window.Resources
>
<
Style
TargetType="Button">
<
Setter
Property="Margin" Value="10"></
Setter
>
<
Style.Triggers
>
<
EventTrigger
RoutedEvent="MouseEnter">
<
BeginStoryboard
>
<
Storyboard
>
<
DoubleAnimation
To="20" Duration="0:0:0.2" Storyboard.TargetProperty="Width"></
DoubleAnimation
>
<
DoubleAnimation
To="70" Duration="0:0:0.2" Storyboard.TargetProperty="Height"></
DoubleAnimation
>
</
Storyboard
>
</
BeginStoryboard
>
</
EventTrigger
>
<
EventTrigger
RoutedEvent="MouseLeave">
<
BeginStoryboard
>
<
Storyboard
>
<
DoubleAnimation
To="100" Duration="0:0:0.2" Storyboard.TargetProperty="Width"></
DoubleAnimation
>
<
DoubleAnimation
To="20" Duration="0:0:0.2" Storyboard.TargetProperty="Height"></
DoubleAnimation
>
</
Storyboard
>
</
BeginStoryboard
>
</
EventTrigger
>
</
Style.Triggers
>
</
Style
>
</
Window.Resources
>
<
StackPanel
x:Name="stackPanel">
<
Button
Width="100" Height="20">OK</
Button
>
</
StackPanel
>
</
Window
>
<
font
color="#ff0000">这里DoubleAnimation必须显示设置Button的Width和Height,不能是用默认的,否则会报错。</
font
>
|
王德水