本篇介绍一下自动装配的知识,Spring为了简化配置文件的编写。采用自动装配方式,自动的装载需要的bean。
自动装配 有以下几种方式:
1 byName 通过id的名字与属性的名字进行判断,要保证Bean实例中属性名字与该装配的id名字相同。
2 byType 通过类型确定装配的bean,但是当存在多个类型符合的bean时,会报错。
3 contructor 在构造注入时,使用该装配方式,效果如同byType。
4 autodetect 自动装配,这个测试了,3.0.5版本不可用了,不知道是不是被移除了。
下面简单的看下,自动装配的所需代码:
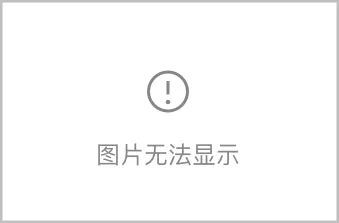
public class Instrumentalist implements Performer{ private String song; private int age; private Instrument instrument; public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getSong() { return song; } public void setSong(String song) { this.song = song; } public Instrument getInstrument() { return instrument; } public void setInstrument(Instrument instrument) { this.instrument = instrument; } public Instrumentalist(){} public Instrumentalist(String song,int age,Instrument instrument){ this.song = song; this.age = age; this.instrument = instrument; } public void perform() throws PerformanceException { System.out.println("Instrumentalist age:"+age); System.out.print("Playing "+song+":"); instrument.play(); } }
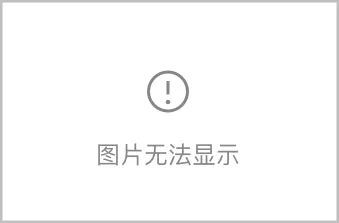
public interface Instrument { public void play(); }
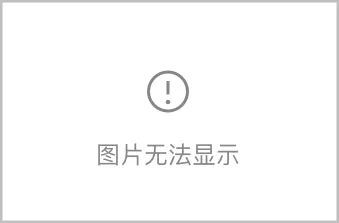
public class Saxophone implements Instrument { public Saxophone(){} public void play() { System.out.println("TOOT TOOT TOOT"); } }
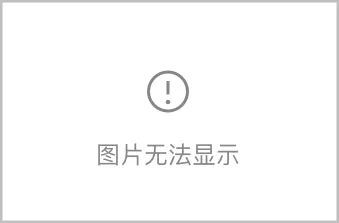
public class test { public static void main(String[] args) throws PerformanceException { ApplicationContext ctx = new ClassPathXmlApplicationContext("bean.xml"); Instrumentalist performer = (Instrumentalist)ctx.getBean("kenny"); performer.perform(); } }
采用byName方式的配置文件如下:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://www.springframework.org/schema/beans" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="instrument" class="com.spring.test.setter.Saxophone"/> <bean id="kenny" class="com.spring.test.setter.Instrumentalist" autowire="byName"> <property name="song" value="Jingle Bells" /> <property name="age" value="25" /> </bean> </beans>
采用byType的配置文件如下:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://www.springframework.org/schema/beans" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="test2" class="com.spring.test.setter.Saxophone"/> <bean id="test1" class="com.spring.test.setter.Saxophone" primary="true"/> <!-- 默认是false --> <bean id="kenny" class="com.spring.test.setter.Instrumentalist" autowire="byType"> <property name="song" value="Jingle Bells" /> <property name="age" value="25" /> </bean> </beans>
如果有多个类型匹配的bean,则可以采用 primary 来设置主要装配的bean,默认情况下是false。
本文转自博客园xingoo的博客,原文链接:【Spring实战】—— 8 自动装配,如需转载请自行联系原博主。