EasyX之跳跳球
- 实现绘制小球和矩形到固定位置
- 让矩形向左移动
- 使小球实现起跳与下落
- 解决小球二次起跳问题
- 判断小球起跳的过程中是否碰到矩形的左边,上边,以及右边,若碰到,游戏结束,分数清零
- 分数的打印
#define _CRT_SECURE_NO_WARNINGS
#include<graphics.h> //图形库头文件
#include<cstdio>
#include<conio.h>
#include<time.h>
#define WIDTH 800
#define HEIGHT 400
//打印分数
void printScore(int score)
{
char buffer[20]; //注意使用项目属性为多字节字符集
sprintf(buffer, "分数: %d ", score);
settextcolor(RED); //设置字体颜色
settextstyle(40, 0, "宋体"); //设置字体大小以及风格
outtextxy(50, 50, buffer); //输出字体
}
int main()
{
srand((unsigned int)time(NULL)); //随机数种子
initgraph(WIDTH, HEIGHT); //创建窗口
setbkcolor(WHITE); //设置窗口背景色
cleardevice(); //清屏
setfillcolor(RED); //给小球和方块一个初始填充颜色
//初始化小球数据
int radius = 10;
int ball_x = WIDTH / 4;
int ball_y = HEIGHT - radius;
int ball_vy = 0; //y方向速度
int ball_a = 14; //小球加速度
//初始化矩形数据
int rec_width = 20;
int rec_height = 100;
int rec_left_topx = 3*WIDTH/4;
int rec_left_topy = HEIGHT- rec_height;
int rec_vx = -20; //矩形X方向速度
int score = 0; //分数
bool flag = false; //判断小球是否能起跳标志
char userKey = '\0'; //用户按键输入变量接收
while (1)
{
BeginBatchDraw(); //双缓冲绘图
//如果矩形跑到最左边然后重新生成一个矩形
if ((rec_left_topx + rec_width) <= 0)
{
score+=10;
rec_width = rand() % 21 + 10;
rec_height = rand() % 81 + 80;
rec_left_topx = WIDTH;
rec_left_topy = HEIGHT - rec_height;
rec_vx = rand() % 5 + (-40);
setfillcolor(RGB(rand() % 255, rand() % 255, rand() % 255));
}
if (_kbhit()) //判断是否有按键按下
{
char userKey = _getch();
if (userKey == ' ' && flag == true) //小球在地上并且用户输入为空格
{
flag = false;
ball_vy = -80; //给小球一个向上的速度
}
}
ball_vy = ball_vy + ball_a; //赋予加速度
ball_y = ball_y + ball_vy; //小球起跳
//小球落地
if (ball_y >= HEIGHT - radius)
{
flag = true;
ball_vy = 0; //将速度置为0
ball_y = HEIGHT - radius; //防止小球落到地上
}
rec_left_topx = rec_left_topx + rec_vx; //设置矩形速度
//设置游戏结束标志
if ((ball_x + radius >= rec_left_topx) && //小球在矩形左边
(ball_y + radius >= HEIGHT - rec_height) && //小球在矩形上边
(ball_x - radius <= rec_left_topx + rec_width)) //小球在矩形右边
{
score = 0;
Sleep(100);
MessageBox(GetHWnd(), "You die,Now B!", "游戏结束", MB_OK); //告知玩家游戏结束
}
cleardevice();
//绘制小球
fillcircle(ball_x, ball_y, radius);
//绘制矩形
fillrectangle(rec_left_topx, rec_left_topy,
rec_left_topx + rec_width, rec_left_topy + rec_height);
//打印分数
printScore(score);
Sleep(100);
EndBatchDraw();
}
closegraph();
system("pause");
return 0;
}
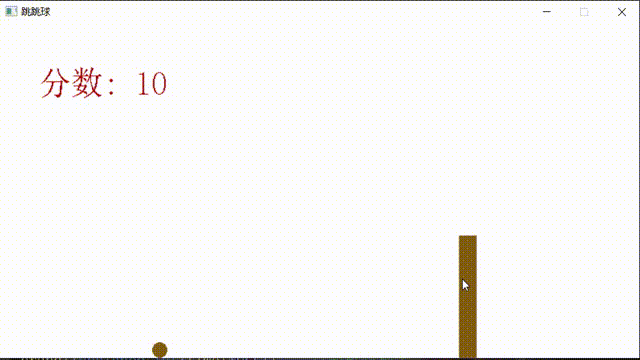