概览
- 了解阿里云用户权限操作
- 开通阿里云短信服务
- 添加短信模板
- 添加签名
- 编写测试代码
- 编写可复用的微服务接口,实现验证码的发送

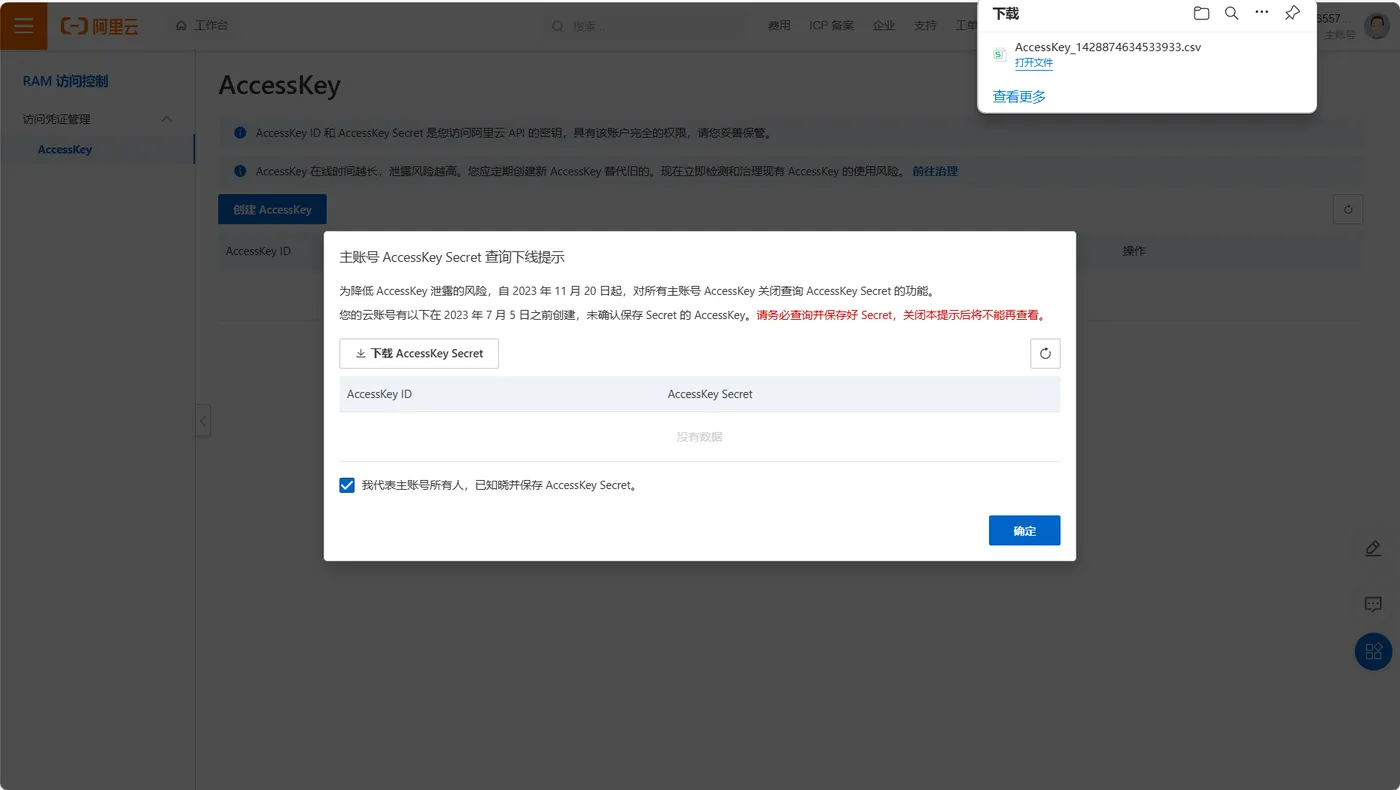
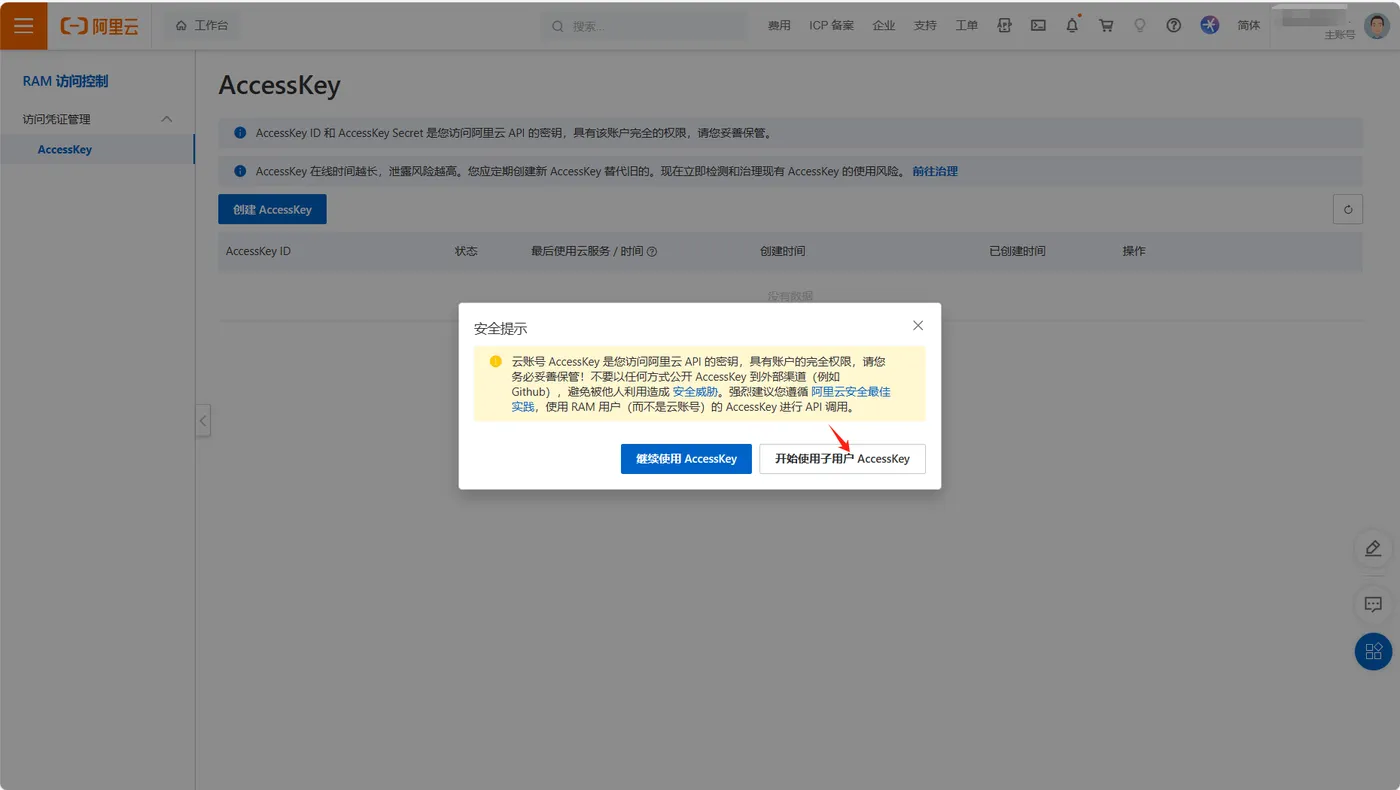
pom依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.28</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.3.3</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.18</version>
</dependency>
yml配置
# 短信配置
ly:
sms:
accessKeyId: xxxxxxxx
accessKeySecret: xxxxxxxx
signName: robindeblog
verifyTemplateCode: SMS_295690184 # 短信模板名称
# redis配置
spring:
data:
redis:
port: 6379
host: localhost
database: 0
timeout: 1800000
配置类
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Component
public class MsmConfig {
@Value("${ly.sms.accessKeyId}")
private String accessKeyId ;
@Value("${ly.sms.accessKeySecret}")
private String accessKeySecret;
@Value("${ly.sms.signName}")
private String signName;
@Value("${ly.sms.verifyTemplateCode}")
private String verifyTemplateCode;
}
service 层
interface
package com.robin.webcliweb.service;
import java.util.Map;
public interface MsmService {
boolean send(Map<String, Object> param, String phone);
}
impl
package com.robin.webcliweb.service.impl;
import com.alibaba.fastjson.JSONObject;
import com.aliyuncs.CommonRequest;
import com.aliyuncs.CommonResponse;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.profile.DefaultProfile;
import com.robin.webcliweb.config.MsmConfig;
import com.robin.webcliweb.service.MsmService;
import jakarta.annotation.Resource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.util.StringUtils;
import java.util.Map;
@Service
public class MsmServiceImpl implements MsmService {
@Resource
private MsmConfig msmConfig;
@Override
public boolean send(Map<String, Object> param, String phone) {
if(StringUtils.isEmpty(phone)) return false;
DefaultProfile profile = DefaultProfile.getProfile("default", msmConfig.getAccessKeyId(), msmConfig.getAccessKeySecret());
IAcsClient client = new DefaultAcsClient(profile);
CommonRequest request = new CommonRequest();
request.setMethod(MethodType.POST);
request.setDomain("dysmsapi.aliyuncs.com");
request.setVersion("2017-05-25");
request.setAction("SendSms");
request.putQueryParameter("PhoneNumbers", phone);
request.putQueryParameter("SignName", msmConfig.getSignName());
request.putQueryParameter("TemplateCode", msmConfig.getVerifyTemplateCode());
request.putQueryParameter("TemplateParam", JSONObject.toJSONString(param));
try {
CommonResponse response = client.getCommonResponse(request);
System.out.println(response.getData());
return response.getHttpResponse().isSuccess();
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
}
controller层
package com.robin.webcliweb.controller;
import cn.hutool.core.util.RandomUtil;
import com.robin.webcliweb.service.MsmService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
@RestController
public class MsmApiController {
@Autowired
private MsmService msmService;
@Autowired
private RedisTemplate<String, String> redisTemplate;
@GetMapping(value = "/send/{phone}")
public Boolean code(@PathVariable String phone) {
String code = redisTemplate.opsForValue().get(phone);
if(!StringUtils.isEmpty(code)) return false;
code = RandomUtil.randomNumbers(6).toString();
Map<String,Object> param = new HashMap<>();
param.put("code", code);
boolean isSend = msmService.send(param,phone);
if(isSend) {
redisTemplate.opsForValue().set(phone, code,5, TimeUnit.MINUTES);
return true;
} else {
return false;
}
}
}