import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class doubleBall {
public static void main(String[] args) {
int[] doubleBall = createDoubleBall();
//作弊代码
//System.out.println("双色球号码:" + Arrays.toString(doubleBall));
int[] ballCount = compareDoubleBall(userSelectNumbers(),doubleBall);
//输出获奖信息
outMsg(ballCount);
}
/**
* 比较系统创建的双色球号码和用户输入的双色求号吗大小
* @param userBall 用户输入的双色球号码
* @param creatBall 系统创建的双色秋号码
* @return 返回相同的红球个数和蓝球的个数组成的数组
*/
public static int[] compareDoubleBall(int[] userBall, int[] creatBall) {
int countR = 0;
for (int i = 0; i < userBall.length - 1; i++) {
for (int j = 0; j < creatBall.length - 1; j++) {
if (userBall[i] == creatBall[j]) {
countR++;
break;
}
}
}
int countB = userBall[6] == creatBall[6] ? 1 : 0;
System.out.println("相同的红球有" + countR + "个" + "相同的蓝球有" + countB + "个");
return new int[]{countR,countB};
}
/**
* 创建双色球
* @return 返回系统创建的双色球号码
*/
public static int[] createDoubleBall() {
Random random = new Random();
int[] doubleBall = new int[7];
int count = 0;
while (count < 6){
int rdNum = random.nextInt(33) + 1;
if (exists(doubleBall, rdNum)){
System.out.println("重复双色球号码:" + rdNum);
continue;
} else {
doubleBall[count] = rdNum;
count++;
}
}
doubleBall[6] = random.nextInt(16) + 1;
return doubleBall;
}
/**
*用户输入双色球
* @return 返回用户输入的双色球号码
*/
public static int[] userSelectNumbers() {
Scanner sc = new Scanner(System.in);
Random random = new Random();
int[] doubleBall = new int[7];
int count = 0;
while (count < 6) {
System.out.println("请输入第" + (count + 1) + "个红色双色球号码(1-33)");
int scI = sc.nextInt();
if (scI < 1 || scI > 33 || exists(doubleBall, scI)) {
System.out.println("请输入正确的双色球号码");
continue;
} else {
doubleBall[count] = scI;
count++;
}
}
while (true) {
System.out.println("请输入蓝色双色球号码");
int scBlue = sc.nextInt();
if (scBlue < 1 || scBlue > 16) {
System.out.println("请输入正确的双色球号码");
} else {
doubleBall[6] = scBlue;
break;
}
}
return doubleBall;
}
/**
* 判断数是否在数组中
* @param arr 要判断的数组
* @param kay 要判断的数
* @return 在返回true,不在返回false
*/
public static boolean exists(int[] arr, int kay) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == kay) {
return true;
}
}
return false;
}
/**
* 输出获奖信息
* @param ballCount 传入相同的红蓝球个数
*/
public static void outMsg(int[] ballCount) {
if (ballCount[0] == 6 && ballCount[1] == 1){
System.out.println("恭喜你,获得一等奖(最高1000万)");
} else if (ballCount[0] == 6 && ballCount[1] == 0) {
System.out.println("恭喜你,获得二等奖(最高500万)");
} else if (ballCount[0] == 5 && ballCount[1] == 1) {
System.out.println("恭喜你,获得三等奖(3000元)");
} else if (ballCount[0] == 5 && ballCount[1] == 0) {
System.out.println("恭喜你,获得四等奖(200元)");
} else if (ballCount[0] == 4 && ballCount[1] == 1) {
System.out.println("恭喜你,获得四等奖(200元)");
} else if (ballCount[0] == 4 && ballCount[1] == 0) {
System.out.println("恭喜你,获得五等奖(10元)");
} else if (ballCount[0] == 3 && ballCount[1] == 1) {
System.out.println("恭喜你,获得五等奖(10元)");
} else if (ballCount[0] == 2 && ballCount[1] == 1) {
System.out.println("恭喜你,获得六等奖(5元)");
} else if (ballCount[0] == 1 && ballCount[1] == 1) {
System.out.println("恭喜你,获得六等奖(5元)");
} else if (ballCount[0] == 0 && ballCount[1] == 1) {
System.out.println("恭喜你,获得谢谢惠顾");
}
}
}
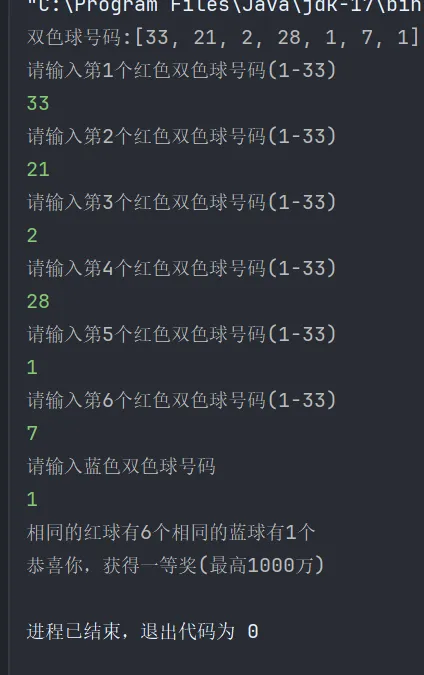