1.准备工作
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--配置编码过滤器,在此之前不能获取任何参数,不然无效-->
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--配置请求方式过滤器,put和delete的HiddenHttpMethodFilter过滤器-->
<filter>
<filter-name>HiddenHttpMethodFilter</filter-name>
<filter-class>org.springframework.web.filter.HiddenHttpMethodFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>HiddenHttpMethodFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--配置springmvc前端控制器-->
<servlet>
<servlet-name>DispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springMVC.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>DispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!--配置扫描组件-->
<context:component-scan base-package="cn.zhao.controller,cn.zhao.dao"></context:component-scan>
<!--配置视图解析器-->
<!--配置 Thymeleaf视图解析器-->
<bean id="viewResolver" class="org.thymeleaf.spring5.view.ThymeleafViewResolver">
<property name="order" value="1"/>
<property name="characterEncoding" value="UTF-8"/>
<property name="templateEngine">
<bean class="org.thymeleaf.spring5.SpringTemplateEngine">
<property name="templateResolver">
<bean class="org.thymeleaf.spring5.templateresolver.SpringResourceTemplateResolver">
<!--视图前缀-->
<property name="prefix" value="/WEB-INF/templates/"/>
<!--视图后缀-->
<property name="suffix" value=".html"/>
<property name="templateMode" value="HTML5"/>
<property name="characterEncoding" value="UTF-8"/></bean>
</property>
</bean>
</property>
</bean>
</beans>
package cn.zhao.pojo;
/**
* @author stevezhao
* @version 1.0
* @description: 员工类
* @date 2022/3/20 17:11
*/
public class Employee {
private Integer id;
private String lastName;
private String email;
/**
* 1 male, 0 female
*/
private Integer gender;
public Employee(Integer id, String lastName, String email, Integer gender) {
this.id = id;
this.lastName = lastName;
this.email = email;
this.gender = gender;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Integer getGender() {
return gender;
}
public void setGender(Integer gender) {
this.gender = gender;
}
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", lastName='" + lastName + '\'' +
", email='" + email + '\'' +
", gender=" + gender +
'}';
}
}
package cn.zhao.dao;
import cn.zhao.pojo.Employee;
import org.springframework.stereotype.Repository;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* @author stevezhao
* @version 1.0
* @description: dao
* @date 2022/3/20 17:14
*/
@Repository
public class EmployeeDao {
private static Map<Integer, Employee> employees = null;
static {
employees = new HashMap<Integer, Employee>();
employees.put(1001,new Employee(1001,"EE-AA","aa@163.com",1));
employees.put(1002,new Employee(1002,"EE-BB","bb@163.com",1));
employees.put(1003,new Employee(1003,"EE-CC","cc@163.com",0));
employees.put(1004,new Employee(1004,"EE-DD","dd@163.com",0));
employees.put(1005,new Employee(1005,"EE-EE","ee@163.com",1));
}
private static Integer initId = 10006;
public void save(Employee employee){
if (employee.getId() == null){
employee.setId(initId++);
}
employees.put(employee.getId(),employee);
}
public Collection<Employee> getALl(){
return employees.values();
}
public Employee get(Integer id){
return employees.get(id);
}
public void delete(Integer id){
employees.remove(id);
}
}
2.完成首页
<!--配置首页-->
<mvc:view-controller path="/" view-name="index"></mvc:view-controller>
<!--开启mvc注解驱动-->
<mvc:annotation-driven></mvc:annotation-driven>
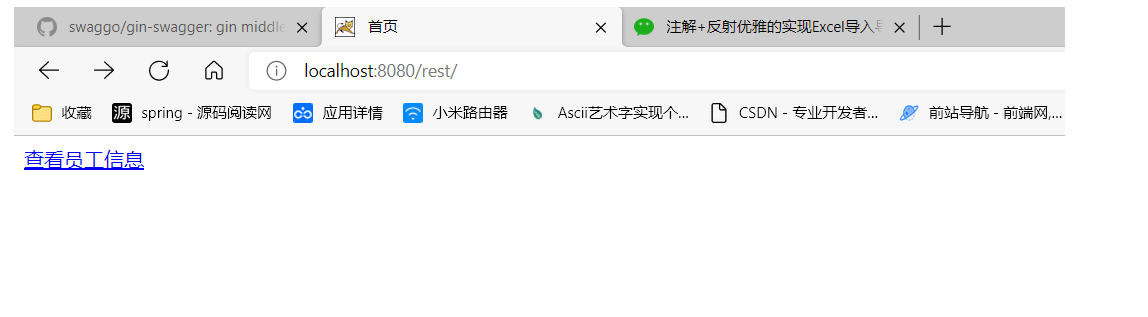
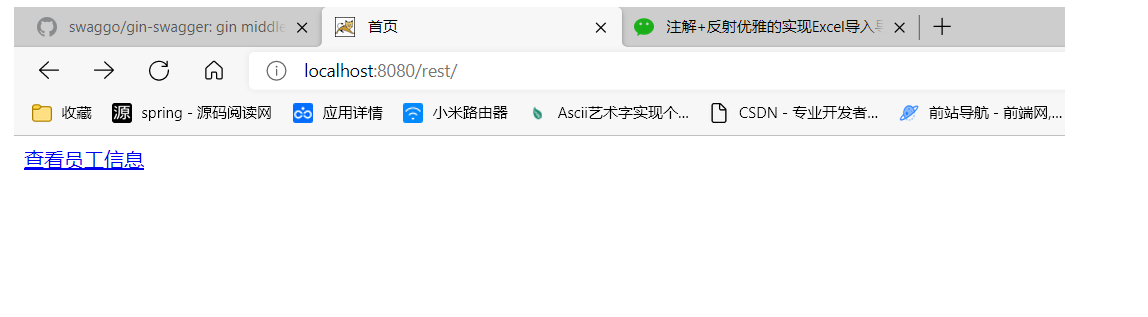
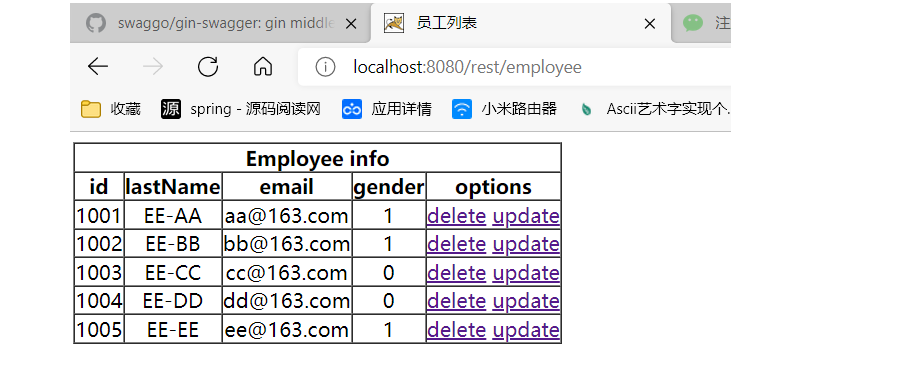
4.删除员工(超链接)
/**
* 删除员工
* @param id
* @return
*/
@RequestMapping(value = "/employee/{id}",method = RequestMethod.DELETE)
public String deleteEmployee(@PathVariable("id") Integer id ){
employeeDao.delete(id);
// 用重定向,让浏览器重发请求,如果是用转发,还是那个请求
return "redirect:/employee";
}
如何获取id
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>员工列表</title>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="0" style="text-align: center">
<tr>
<th colspan="5">Employee info</th>
</tr>
<tr>
<th>id</th>
<th>lastName</th>
<th>email</th>
<th>gender</th>
<th>options</th>
</tr>
<tr th:each="employee : ${employeeList}">
<td th:text="${employee.id}"></td>
<td th:text="${employee.lastName}"></td>
<td th:text="${employee.email}"></td>
<td th:text="${employee.gender}"></td>
<td>
// 第一种方法
<a th:href="@{/employee/}+${employee.id}">delete</a>
<a th:href="@{/employee}">update</a>
</td>
</tr>
</table>
</body>
</html>
另一种方法
<td>
// 第二种方法
<a th:href="@{'/employee/'+${employee.id}}">delete</a>
<a th:href="@{/employee}">update</a>
</td>
继续完善
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>员工列表</title>
</head>
<body>
<table id="dataTable" border="1" cellspacing="0" cellpadding="0" style="text-align: center">
<tr>
<th colspan="5">Employee info</th>
</tr>
<tr>
<th>id</th>
<th>lastName</th>
<th>email</th>
<th>gender</th>
<th>options</th>
</tr>
<tr th:each="employee : ${employeeList}">
<td th:text="${employee.id}"></td>
<td th:text="${employee.lastName}"></td>
<td th:text="${employee.email}"></td>
<td th:text="${employee.gender}"></td>
<td>
<a @click="deleteEmployee" th:href="@{'/employee/'+${employee.id}}" >delete</a>
<a th:href="@{/employee}">update</a>
</td>
</tr>
</table>
<form method="post" id="deleteForm">
<input type="hidden" name="_method" value="delete">
</form>
<script type="text/javascript" th:src="@{/static/js/vue.js}"></script>
<script type="text/javascript">
var vue = new Vue({
el: "#dataTable",
methods: {
deleteEmployee:function (event) {
// 根据id获取表单元素
var deleteForm = document.getElementById("deleteForm");
// 触发点击事件的超链接的href属性赋值给表单action
deleteForm.action = event.target.href;
// 提交表单
deleteForm.submit();
// 取消超链接默认行为
event.preventDefault();
}
}
});
</script>
</body>
</html>
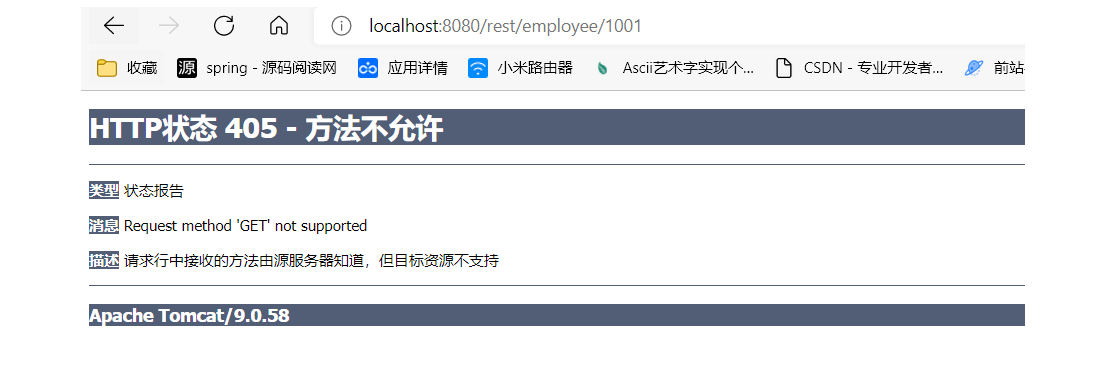