生成二维码
方法一:qrcanvas(支持中文)
1. 安装依赖:
npm i qrcanvas --save
2. 新建一个组件Component,通过这个组件来提供生成二维码的能力
ionic g component qrcode
3. 在qrcode包下新增一个qrcode.module.ts文件,文件及文件内容如下:
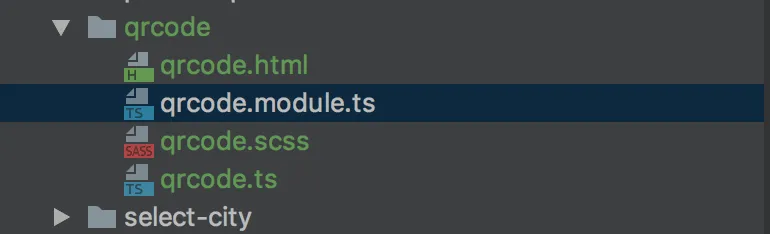
qrcode
import {NgModule} from '@angular/core';
import {IonicPageModule} from 'ionic-angular';
import {QrcodeComponent} from "./qrcode";
@NgModule({
declarations: [
QrcodeComponent,
],
imports: [
IonicPageModule.forChild(QrcodeComponent)
],
exports: [
QrcodeComponent
]
})
export class I2fQrCodeModule {
}
4. 编写qrcode.ts文件代码实现二维码生成,代码如下:
import {AfterViewInit, Component, ElementRef, Input, OnChanges, SimpleChanges} from '@angular/core';
import qrcanvas from 'qrcanvas';
@Component({
selector: 'i2f-qrcode',
templateUrl: 'qrcode.html'
})
export class QrcodeComponent implements AfterViewInit, OnChanges {
options: any;
effects = ['none', 'liquid', 'round', 'spot'];
@Input() size: string;
@Input() data: string;
@Input() logo: string;
constructor(private elementRef: ElementRef) {
}
ngAfterViewInit() {
}
ngOnChanges(changes: SimpleChanges) {
if (
'backgroundAlpha' in changes ||
'foregroundAlpha' in changes ||
'mime' in changes ||
'padding' in changes ||
'size' in changes ||
'data' in changes ||
'logo' in changes ||
'canvas' in changes) {
this.generateQrCode();
}
}
generateQrCode() {
let innerHTML = '';
this.elementRef.nativeElement.querySelector('#qrcode').innerHTML = innerHTML;
this.options = {
cellSize: 8,
size: this.size,
correctLevel: 'H',
data: this.data,
effect: {
key: 'none',
value: 1,
}
};
this.options.effect.key = this.effects[Math.floor(Math.random() * this.effects.length)];
if (this.logo) {
let image = new Image();
image.src = this.logo;
this.options.logo = {
image,
size: 10 / 100
};
image.onload = () => {
const canvas = qrcanvas(this.options);
this.elementRef.nativeElement.querySelector('#qrcode').appendChild(canvas);
};
} else {
const canvas = qrcanvas(this.options);
this.elementRef.nativeElement.querySelector('#qrcode').appendChild(canvas);
}
}
}
5. 编写页面qrcode.html,代码如下:
<div id="qrcode" (click)="generateQrCode()"></div>
6. 使用I2f-qrcode生成二维码:
在页面中:
<i2f-qrcode [data]="'中文'" [size]="180" [logo]="'assets/img/logo.png'" ></i2f-qrcode>
在module.ts中引入
imports: [
IonicPageModule.forChild(QrCodePage),
I2fQrCodeModule
],
7. 结果展示
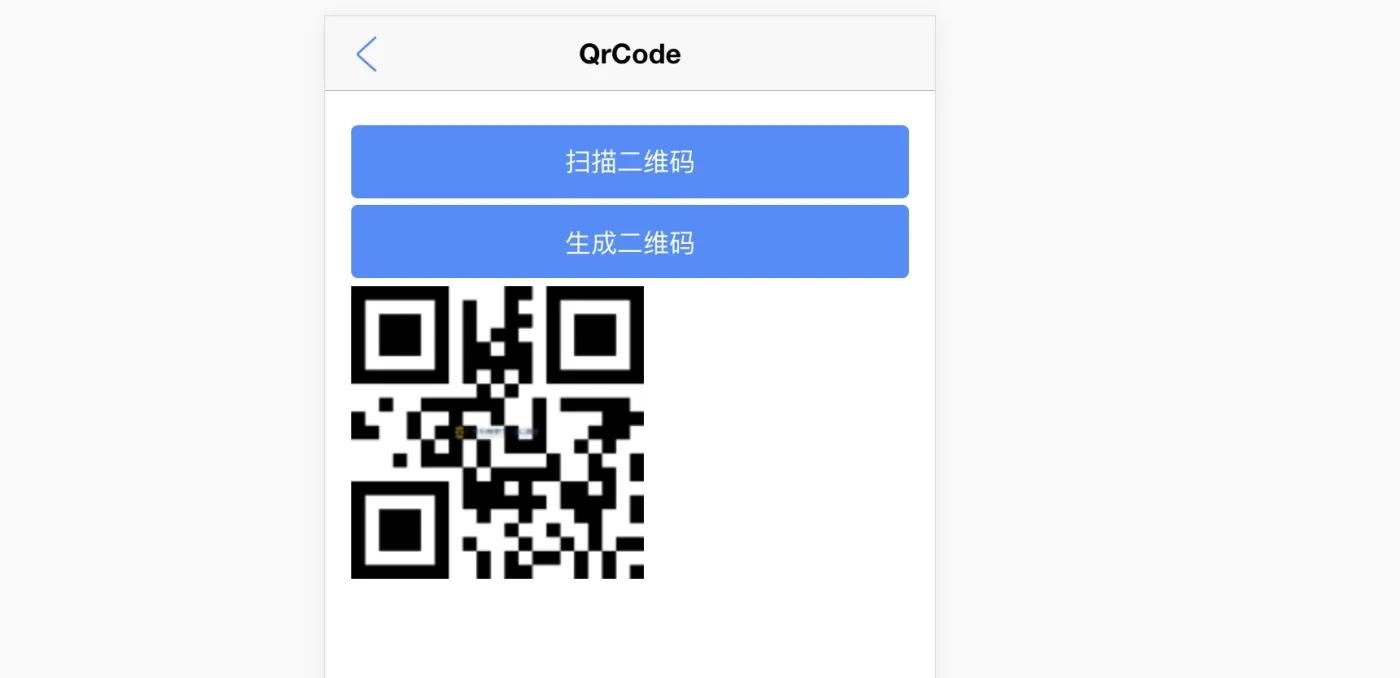
image.png
方法二. angular2-qrcode(不支持中文)
1. 安装npm依赖:
npm install angular2-qrcode --save
2. 在app.module.ts中引入QrCodeModule模块
import { QRCodeModule } from 'angular2-qrcode';
imports: [... QRCodeModule... ],
3. 在实际调用页面的module.ts中引入QRCodeModule,并在页面中以指令方式使用
imports: [QRCodeModule],
<qr-code [value]="qrCodeValue"></qr-code>
qrCodValue是一个变量,也可以是一个写死的值。
- 可选参数:
参数 | 类型 | 默认值 | 描述 |
---|---|---|---|
value | String | '' | Your data string |
size | Number | 128 | This is the height/width of your QR Code component |
level | String | 'M' | QR Correction level ('L', 'M', 'Q', 'H') |
type | Number | 4 | Buffer size for data string |
background | String | 'white' | The color for the background |
backgroundAlpha | Number | 1.0 | The opacity of the background |
foreground | String | 'black' | The color for the foreground |
foregroundAlpha | Number | 1.0 | The opacity of the foreground |
mime | String | 'image/png' | The mime type for the output image |
padding | Number | null | The padding around the QR Code |
canvas | Boolean | false | Will output a canvas element if true |