队列是遵循FIFO(First In First Out,先进先出,也称为先来先服务)原则的一组有序的项。
队列在尾部添加新元素,并从顶部移除元素。最新添加的元素必须排在队列的末尾。
在现实中,最常见的队列的例子就是排队。
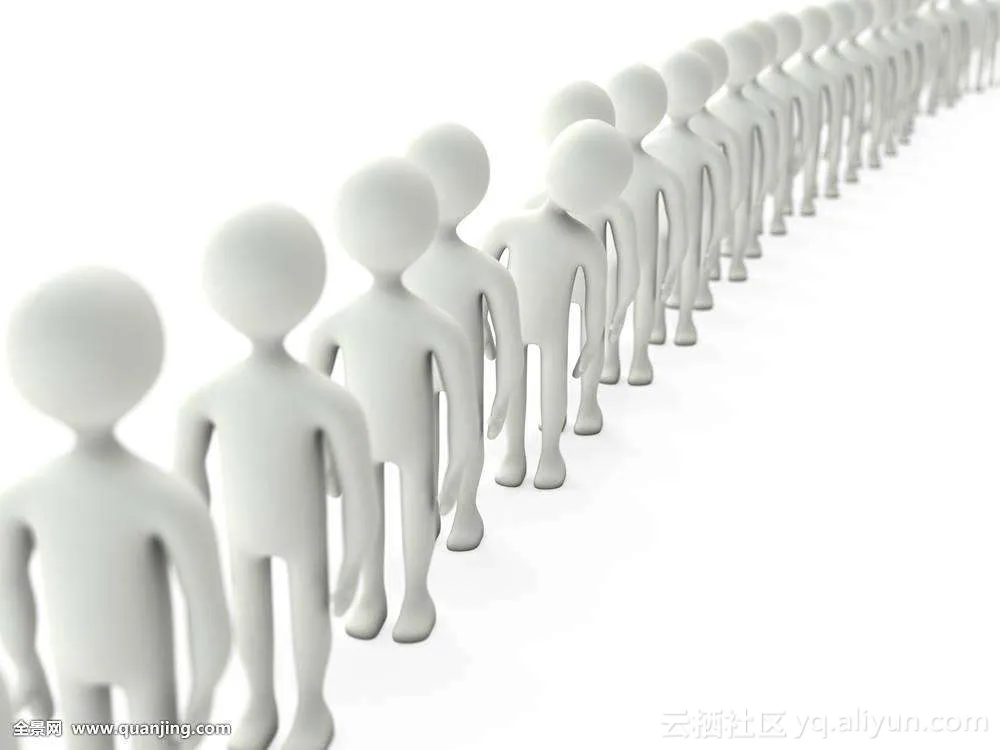
function Queue(){//声明队列对象
var items = [];//队列的承载
//向队列尾部添加一个或多个新的项
this.enqueue = function(el){
items.push(el)
}
//删除队列头部第一个元素
this.dequeue = function(){
return items.shift();
}
//返回队列中第一个元素
this.front = function(){
return items[0];
}
//确定元素是否为空 为空则为true 不为空则为false
this.isEmpty = function(){
return items.length === 0;
}
//返回队列长度
this.size = function(){
return items.length;
}
//打印队列
this.print = function(){
console.log(items.toString())
}
}
使用队列类:
//实例化类
var queue = new Queue();
//验证是否为空
console.log(queue.isEmpty()) //true
queue.enqueue('ma');
queue.enqueue('jack');
queue.print();//ma,jack
console.log(queue.size())//2
queue.dequeue();//jack
queue.print();//jack
console.log(queue.size())//1
