前言
开发中会遇到这样的一个情况,我们得到一个dto对象,里面有几十个属性值,需要将这几十个属性值的N个通过VO传输另外一个地方,一般我们的做法是:
创建VO类,new vo() 对象,通过vo.set(dto.get)的方式不断的设置值。
但是这种方式在属性少量的情况之下还是没有问题,但是在属性不断变化的情况下就令人烦恼了,但是我们可以选择使用反射来完成A对象拷贝到B对象的这一过程。
其主要原理是通过反射获取到A对象的get方法和数据类型,然后截取get之后的字符串,拼接成set方法,再通过反射调用B的set方法和传值。
代码
public class CopyPropertiesUtil {
/**
* 利用反射实现对象之间属性复制
*
* @param from
* @param to
*/
public static void copyProperties(Object from, Object to) throws Exception {
copyPropertiesExclude(from, to, null);
}
/**
* 复制对象属性
*
* @param from
* @param to
* @param excludsArray 排除属性列表
* @throws Exception
*/
@SuppressWarnings("unchecked")
public static void copyPropertiesExclude(Object from, Object to, String[] excludsArray) throws Exception {
List<String> excludesList = null;
if (excludsArray != null && excludsArray.length > 0) {
excludesList = Arrays.asList(excludsArray); //构造列表对象
}
Method[] fromMethods = from.getClass().getDeclaredMethods();
Method[] toMethods = to.getClass().getDeclaredMethods();
Method fromMethod = null, toMethod = null;
String fromMethodName = null, toMethodName = null;
for (int i = 0; i < fromMethods.length; i++) {
fromMethod = fromMethods[i];
fromMethodName = fromMethod.getName();
if (!fromMethodName.contains("get") || fromMethodName.contains("getId"))
continue;
//排除列表检测
if (excludesList != null && excludesList.contains(fromMethodName.substring(3).toLowerCase())) {
continue;
}
toMethodName = "set" + fromMethodName.substring(3);
toMethod = findMethodByName(toMethods, toMethodName);
if (toMethod == null)
continue;
Object value = fromMethod.invoke(from, new Object[0]);
if (value == null)
continue;
//集合类判空处理
if (value instanceof Collection) {
Collection newValue = (Collection) value;
if (newValue.size() <= 0)
continue;
}
toMethod.invoke(to, new Object[]{value});
}
}
/**
* 对象属性值复制,仅复制指定名称的属性值
*
* @param from
* @param to
* @param includsArray
* @throws Exception
*/
@SuppressWarnings("unchecked")
public static void copyPropertiesInclude(Object from, Object to, String[] includsArray) throws Exception {
List<String> includesList = null;
if (includsArray != null && includsArray.length > 0) {
includesList = Arrays.asList(includsArray); //构造列表对象
} else {
return;
}
Method[] fromMethods = from.getClass().getDeclaredMethods();
Method[] toMethods = to.getClass().getDeclaredMethods();
Method fromMethod = null, toMethod = null;
String fromMethodName = null, toMethodName = null;
for (int i = 0; i < fromMethods.length; i++) {
fromMethod = fromMethods[i];
fromMethodName = fromMethod.getName();
if (!fromMethodName.contains("get"))
continue;
//排除列表检测
String str = fromMethodName.substring(3);
if (!includesList.contains(str.substring(0, 1).toLowerCase() + str.substring(1))) {
continue;
}
toMethodName = "set" + fromMethodName.substring(3);
toMethod = findMethodByName(toMethods, toMethodName);
if (toMethod == null)
continue;
Object value = fromMethod.invoke(from, new Object[0]);
if (value == null)
continue;
//集合类判空处理
if (value instanceof Collection) {
Collection newValue = (Collection) value;
if (newValue.size() <= 0)
continue;
}
toMethod.invoke(to, new Object[]{value});
}
}
/**
* 从方法数组中获取指定名称的方法
*
* @param methods
* @param name
* @return
*/
public static Method findMethodByName(Method[] methods, String name) {
for (int j = 0; j < methods.length; j++) {
if (methods[j].getName().equals(name))
return methods[j];
}
return null;
}
}
最后
未完待续、敬请期待!
我的博客地址
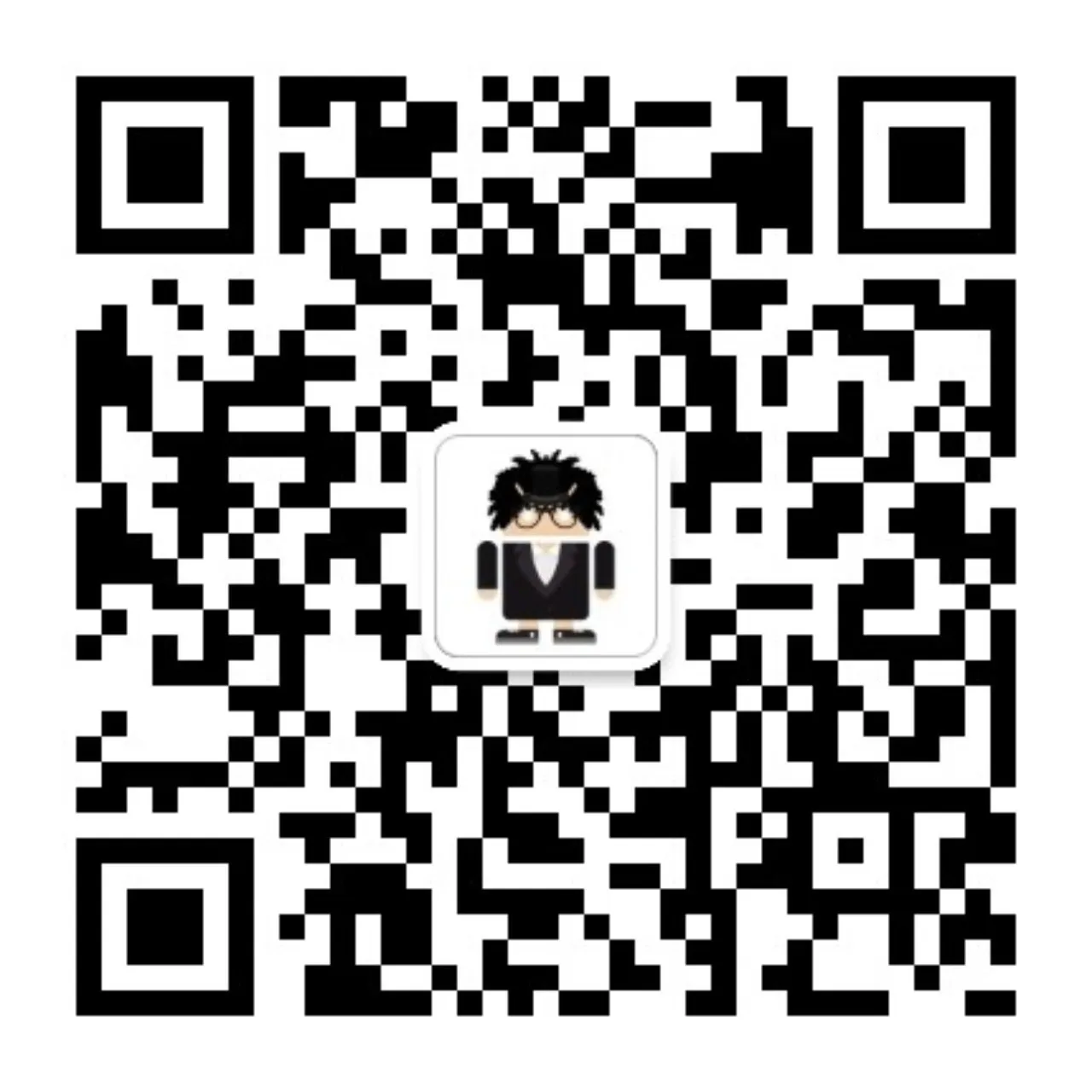
FullScreenDeveloper