一.创建Web工程
创建一个Web站点或者Web应用程序,添加对Castle.ActiveRecord.dll的引用。
二.创建需要持久化的业务实体
在.NET2.0下,由于引入了泛型,创建业务实体比1.1下简单了许多,业务实体只需要继承于泛型的ActiveRecordBase类,其中默认已经实现了一些静态的方法,不需要我们再在业务实体中实现。

































































三.设置配置信息
在Web.config中设置如下信息,这部分与1.1没有什么区别

































四.初始化
ActiveRecord
在Global.asax的
Application_Start
添加初始化代码








五.使用业务实体
这部分也是与1.1一样,同样可以使用Create(),Save(),Update()等方法,不详细说了,这里我们用一个GridView来展示读取国家为UK的员工列表









































后台代码:






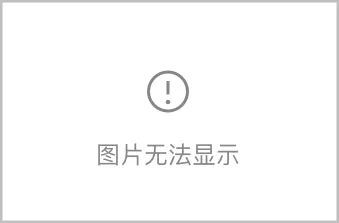
内容有些简单,后续有时间会继续介绍
Castle Active Record for .NET2.0

本文转自lihuijun51CTO博客,原文链接:
http://blog.51cto.com/terrylee/67670
,如需转载请自行联系原作者