继上一篇已过两月有余,上一篇时正巧遇出差。回来时找不到当初的demo程序,于是此系列就暂时放下了。
上一篇:CYQ.Data 轻量数据访问层(六) 自定义数据表实现绑定常用的数据控件(中)
不过,还是得补一篇下,先让它为之小小完整一下:
还记得当初以为似找到:行数组Copy之后,再array.GetEnumerator();就可行。
实际操作之后,发现不可行,于是,这不可行的路就不写了,避免浪费大伙精力看了。
以下讲可行之路:
通过Reflector找到SqlDataReader类,因为它也是可绑定之一的数据源,虽然直拉绑定往往造成链接未关闭事件。
通过研究:
public
class
SqlDataReader : DbDataReader, IDataReader, IDisposable, IDataRecord
发现其继承自DbDataReader,于是,点进DbDataReader:
public
abstract
class
DbDataReader : MarshalByRefObject, IDataReader, IDisposable, IDataRecord, IEnumerable
这里,我们的MDataTable只要继承自中间的IDataReader, IEnumerable两个即可,就可以实现绑定之路了。
继承之后,当然就是要实现其它接口了:
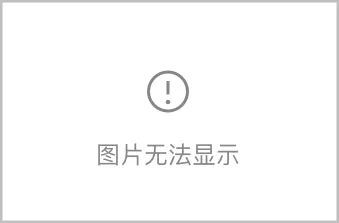
#region
IDataReader 成员
public void Close()
{
_Mdr.Clear();
}
public int Depth
{
get
{
if (_Mdr != null )
{
return _Mdr.Count;
}
return 0 ;
}
}
public DataTable GetSchemaTable()
{
return null ;
}
public bool IsClosed
{
get
{
return true ;
}
}
public bool NextResult()
{
if (_Ptr < _Mdr.Count - 1 )
{
return true ;
}
else
{
return false ;
}
}
public bool Read()
{
if (_Ptr < _Mdr.Count)
{
_Ptr ++ ;
return true ;
}
else
{
return false ;
}
}
public int RecordsAffected
{
get
{
return - 1 ;
}
}
#endregion
#region IDataRecord 成员
private int _Ptr = 0 ;
public int FieldCount
{
get
{
if ( this .Columns != null )
{
return this .Columns.Count;
}
return 0 ;
}
}
public bool GetBoolean( int i)
{
return ( bool )_Mdr[_Ptr][i].Value;
}
public byte GetByte( int i)
{
return ( byte )_Mdr[_Ptr][i].Value;
}
public long GetBytes( int i, long fieldOffset, byte [] buffer, int bufferoffset, int length)
{
throw new Exception( " The method or operation is not implemented. " );
}
public char GetChar( int i)
{
return ( char )_Mdr[_Ptr][i].Value;
}
public long GetChars( int i, long fieldoffset, char [] buffer, int bufferoffset, int length)
{
throw new Exception( " The method or operation is not implemented. " );
}
public IDataReader GetData( int i)
{
return this ;
}
public string GetDataTypeName( int i)
{
return DataType.GetDbTypeFromSqlDbType(_Mdr[_Ptr].Columns[i].SqlType.ToString()).ToString();
}
public DateTime GetDateTime( int i)
{
return (DateTime)_Mdr[_Ptr][i].Value;
}
public decimal GetDecimal( int i)
{
return ( decimal )_Mdr[_Ptr][i].Value;
}
public double GetDouble( int i)
{
return ( double )_Mdr[_Ptr][i].Value;
}
public Type GetFieldType( int i)
{
return Type.GetType( " System. " + GetDataTypeName(i));
}
public float GetFloat( int i)
{
return ( float )_Mdr[_Ptr][i].Value;
}
public Guid GetGuid( int i)
{
return (Guid)_Mdr[_Ptr][i].Value;
}
public short GetInt16( int i)
{
return ( short )_Mdr[_Ptr][i].Value;
}
public int GetInt32( int i)
{
return ( int )_Mdr[_Ptr][i].Value;
}
public long GetInt64( int i)
{
return ( long )_Mdr[_Ptr][i].Value;
}
public string GetName( int i)
{
return _Mdr[_Ptr][i].ColumnName;
}
public int GetOrdinal( string name)
{
throw new Exception( " The method or operation is not implemented. " );
}
public string GetString( int i)
{
return _Mdr[_Ptr][i].ColumnName.ToString();
}
public object GetValue( int i)
{
return null ;
// return _Mdr[_Ptr][i-1].Value;
}
public int GetValues( object [] values)
{
for ( int i = 0 ; i < values.Length; i ++ )
{
values[i] = _Mdr[_Ptr - 1 ][i].Value;
}
return values.Length;
}
public bool IsDBNull( int i)
{
return _Mdr[_Ptr][i].IsNull;
}
public object this [ string name]
{
get
{
return null ;
}
}
public object this [ int i]
{
get
{
return _Mdr[i];
}
}
#endregion
#region IEnumerable 成员
public IEnumerator GetEnumerator()
{
return new System.Data.Common.DbEnumerator( this );
}
#endregion
public void Close()
{
_Mdr.Clear();
}
public int Depth
{
get
{
if (_Mdr != null )
{
return _Mdr.Count;
}
return 0 ;
}
}
public DataTable GetSchemaTable()
{
return null ;
}
public bool IsClosed
{
get
{
return true ;
}
}
public bool NextResult()
{
if (_Ptr < _Mdr.Count - 1 )
{
return true ;
}
else
{
return false ;
}
}
public bool Read()
{
if (_Ptr < _Mdr.Count)
{
_Ptr ++ ;
return true ;
}
else
{
return false ;
}
}
public int RecordsAffected
{
get
{
return - 1 ;
}
}
#endregion
#region IDataRecord 成员
private int _Ptr = 0 ;
public int FieldCount
{
get
{
if ( this .Columns != null )
{
return this .Columns.Count;
}
return 0 ;
}
}
public bool GetBoolean( int i)
{
return ( bool )_Mdr[_Ptr][i].Value;
}
public byte GetByte( int i)
{
return ( byte )_Mdr[_Ptr][i].Value;
}
public long GetBytes( int i, long fieldOffset, byte [] buffer, int bufferoffset, int length)
{
throw new Exception( " The method or operation is not implemented. " );
}
public char GetChar( int i)
{
return ( char )_Mdr[_Ptr][i].Value;
}
public long GetChars( int i, long fieldoffset, char [] buffer, int bufferoffset, int length)
{
throw new Exception( " The method or operation is not implemented. " );
}
public IDataReader GetData( int i)
{
return this ;
}
public string GetDataTypeName( int i)
{
return DataType.GetDbTypeFromSqlDbType(_Mdr[_Ptr].Columns[i].SqlType.ToString()).ToString();
}
public DateTime GetDateTime( int i)
{
return (DateTime)_Mdr[_Ptr][i].Value;
}
public decimal GetDecimal( int i)
{
return ( decimal )_Mdr[_Ptr][i].Value;
}
public double GetDouble( int i)
{
return ( double )_Mdr[_Ptr][i].Value;
}
public Type GetFieldType( int i)
{
return Type.GetType( " System. " + GetDataTypeName(i));
}
public float GetFloat( int i)
{
return ( float )_Mdr[_Ptr][i].Value;
}
public Guid GetGuid( int i)
{
return (Guid)_Mdr[_Ptr][i].Value;
}
public short GetInt16( int i)
{
return ( short )_Mdr[_Ptr][i].Value;
}
public int GetInt32( int i)
{
return ( int )_Mdr[_Ptr][i].Value;
}
public long GetInt64( int i)
{
return ( long )_Mdr[_Ptr][i].Value;
}
public string GetName( int i)
{
return _Mdr[_Ptr][i].ColumnName;
}
public int GetOrdinal( string name)
{
throw new Exception( " The method or operation is not implemented. " );
}
public string GetString( int i)
{
return _Mdr[_Ptr][i].ColumnName.ToString();
}
public object GetValue( int i)
{
return null ;
// return _Mdr[_Ptr][i-1].Value;
}
public int GetValues( object [] values)
{
for ( int i = 0 ; i < values.Length; i ++ )
{
values[i] = _Mdr[_Ptr - 1 ][i].Value;
}
return values.Length;
}
public bool IsDBNull( int i)
{
return _Mdr[_Ptr][i].IsNull;
}
public object this [ string name]
{
get
{
return null ;
}
}
public object this [ int i]
{
get
{
return _Mdr[i];
}
}
#endregion
#region IEnumerable 成员
public IEnumerator GetEnumerator()
{
return new System.Data.Common.DbEnumerator( this );
}
#endregion
至此,MDataTable已经可以替代常用的DataTable了。
同样具有常见的行,列,数据结构,绑定控件等功能。
版权声明:本文原创发表于博客园,作者为路过秋天,原文链接:
http://www.cnblogs.com/cyq1162/archive/2010/01/29/1658976.html